Look for a while to see all the details!
/*
Min Jun Kim
15104
Project 10
This program draws a highway setting with randomly made objects
*/
var terrainSpeed = 0.0001;
var terrainDetail = 0.0005;
var terrainmapx = [];
var terrainmapy = [];
var dec = 0; //counter for time
var cars = []; //array of cars
function setup() {
//sets up canvas and initial 3 cars
createCanvas(640, 400);
for (var i = 0; i < 3; i++) {
var rx = random(width);
cars[i] = makeCar(rx);
}
}
function draw() {
background(0);
fill(0);
stroke(0);
beginShape();
for (var x = -30; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0,1, 0, height)+100;
//draws the wavey terrain background
vertex(0,height);
vertex(width/2, height);
vertex(width, height);
vertex(x, y);
//index the values for access
terrainmapx[x]=x;
terrainmapy[x]= y;
}
endShape();
//specifications of drawing future items
stroke(100);
rectMode(CENTER);
//when the array gets too full erase the old values
for (i = 0; i < terrainmapx.length; i+=1) {
if(terrainmapx.length > 700) {
terrainmapx.pop();
terrainmapy.pop();
}
//draws the highway
stroke(50);
fill(50);
rect(i, terrainmapy[i]-65,30,110);
fill(100);
stroke(100);
rect(i, terrainmapy[i],30,20);
rect(i, terrainmapy[i]-130,20,20);
}
//draws the while center line of the highway
for (i = 0; i < width+55; i += 50) {
fill(240);
rect(i+20, terrainmapy[i]-60, 30, 7);
}
//calls functions for the car
DisplaynUpdateCars();
addCarWithSomeRandomProbability();
//draw the car going right that stays on the canvas
push();
translate(150, terrainmapy[150]-30);
rotate(degrees((atan2(terrainmapy[640]-terrainmapy[150]-30,640-150))+360%360))
fill(250,240,95,80);
noStroke();
triangle(15,0,100,40,100,-40);
fill(0);
rect(0,0, 60, 30);
fill(240,0,0);
rect(0,0, 30, 30);
pop()
//draws the moon
fill(250,240,95);
ellipse(50, terrainmapy[50]-300, 50,50);
fill(0);
ellipse(62, terrainmapy[50]-300, 30,30);
//draws the raindrops
for (var y = 5; y <= 480; y+=20) {
for (var x = 5; x <= 640; x += 10) {
stroke(0,40,50,80);
line(x,y,x,y+random(0,20));
}
}
}
function carMove() {
//updates the speed and location of the car
if (oldspeed > this.speed) {
this.speed = this.speed+ 10;
}
var oldspeed = this.speed;
this.x += this.speed;
}
function DisplaynUpdateCars() {
//calls the display and update function
for (var i = 0; i < cars.length; i++) {
cars[i].display();
cars[i].move();
}
}
function carsDisplay() {
//draws the car itself
noStroke();
var heighty= terrainmapy[round(this.x)];
push();
for (i=0; i<10; i++) {
fill(250,240,95,10);
triangle(this.x, heighty-85, this.x-this.sizeX, heighty-85-40,this.x-this.sizeX,heighty-85+40);
fill(this.colory,100,100);
rect(this.x, heighty-85, this.sizeX, this.sizeX/2);
push();
fill(200);
rect(this.x, heighty-85, this.sizeX/2, this.sizeX/2);
pop()
}
pop();
}
function addCarWithSomeRandomProbability() {
//probability of a car increases over time, which guarentees
//that there won't be too long of a gap between cars
dec += 1;
if (random(0,1) < dec/500) {
cars.push(makeCar(width));
dec = 0;
}
}
function makeCar(birthLocationX) {
//specifications of the car
var car = {x: birthLocationX,
sizeX: random(60,120),
colory: random(250),
speed: random(-20,-15),
move: carMove,
display: carsDisplay
}
return car;
}
I wanted to make a program that draws on uniquely created terrain, so I studied the way of using noise to create new terrain. Then I made it such that the terrain appears to be smooth and wavy. I indexed the values for later use, and made it such that it disappears when reaching a certain limit- This helped improve the speed of the animation and reduced the need for indexing the x value. I then made it such that random cars appear on both sides, but it looked unrealistic (physics wise) so I made one car stay in the same spot. To make things more moody, I changed it to a night-setting with transparent headlights, a moon and the rain. One problem that I had was that sometimes, the car would overlap when initially starting out, and I tried to go around this problem by changing the range of the speed and adding a counter to the probability of making a new car. I really liked how this project turned out and it taught me well about object programming.
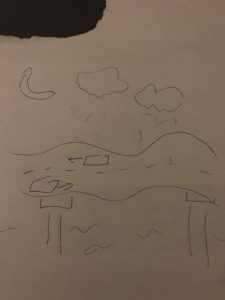