judyli: Composition Project 11
/*
Judy Li
Section A
judyli@andrew.cmu.edu
Project-11
*/
var ttl1 = [];//turtle 1
var ttl2 = [];//turtle 2
var ttl3 = [];//turtle 3
var ttl4 = [];//turtle 4
var opacity = 255;//starting color
function setup() {
createCanvas(480, 480);
background("white");
strokeJoin(MITER);
strokeCap(PROJECT);
//ttl1
for (var i = 0; i < 10; i++) {
ttl1.push(makeTurtle(0, 0));
ttl1[i].penDown();
ttl1[i].right(36 * i);
}
//ttl2
for (var j = 0; j < 10; j++) {
ttl2.push(makeTurtle(480, 0));
ttl2[j].penDown();
ttl2[j].left(36 * j);
}
//ttl3
for (var k = 0; k < 10; k++) {
ttl3.push(makeTurtle(0, 480));
ttl3[k].penDown();
ttl3[k].right(36 * k);
}
//ttl4
for (var l = 0; l < 10; l++) {
ttl4.push(makeTurtle(480, 480));
ttl4[l].penDown();
ttl4[l].left(36 * l);
}
}
function draw() {
var dir = random(15, 90);
var dist = random(10, 25);
if (opacity > 100) {
opacity -= 10;
}
else opacity = 205;
for (var i = 0; i < 10; i++) {
//1
ttl1[i].setColor(opacity);
ttl1[i].setWeight(2);
ttl1[i].forward(dist);
ttl1[i].right(dir);
//2
ttl2[i].setColor(opacity);
ttl2[i].setWeight(2);
ttl2[i].forward(dist);
ttl2[i].left(dir);
//3
ttl3[i].setColor(opacity);
ttl3[i].setWeight(2);
ttl3[i].forward(dist);
ttl3[i].right(dir);
//4
ttl4[i].setColor(opacity);
ttl4[i].setWeight(2);
ttl4[i].forward(dist);
ttl4[i].left(dir);
}
}
function turtleLeft(d) {
this.angle -= d;
}
function turtleRight(d) {
this.angle += d;
}
function turtleForward(p) {
var rad = radians(this.angle);
var newx = this.x + cos(rad) * p;
var newy = this.y + sin(rad) * p;
this.goto(newx, newy);
}
function turtleBack(p) {
this.forward(-p);
}
function turtlePenDown() {
this.penIsDown = true;
}
function turtlePenUp() {
this.penIsDown = false;
}
function turtleGoTo(x, y) {
if (this.penIsDown) {
stroke(this.color);
strokeWeight(this.weight);
line(this.x, this.y, x, y);
}
this.x = x;
this.y = y;
}
function turtleDistTo(x, y) {
return sqrt(sq(this.x - x) + sq(this.y - y));
}
function turtleAngleTo(x, y) {
var absAngle = degrees(atan2(y - this.y, x - this.x));
var angle = ((absAngle - this.angle) + 360) % 360.0;
return angle;
}
function turtleTurnToward(x, y, d) {
var angle = this.angleTo(x, y);
if (angle < 180) {
this.angle += d;
} else {
this.angle -= d;
}
}
function turtleSetColor(c) {
this.color = c;
}
function turtleSetWeight(w) {
this.weight = w;
}
function turtleFace(angle) {
this.angle = angle;
}
function makeTurtle(tx, ty) {
var turtle = {x: tx, y: ty,
angle: 0.0,
penIsDown: true,
color: color(128),
weight: 1,
left: turtleLeft, right: turtleRight,
forward: turtleForward, back: turtleBack,
penDown: turtlePenDown, penUp: turtlePenUp,
goto: turtleGoTo, angleto: turtleAngleTo,
turnToward: turtleTurnToward,
distanceTo: turtleDistTo, angleTo: turtleAngleTo,
setColor: turtleSetColor, setWeight: turtleSetWeight,
face: turtleFace};
return turtle;
}
For this project, I wanted to create a turtle pattern that fills up the background. I made turtles at four different starting points so that the canvas is filled up more quickly and with more depth of color through differences in color as they move about. When I played with different moves and directions, I liked this composition the best because it looks like the way a knit hat is supposed to be made.
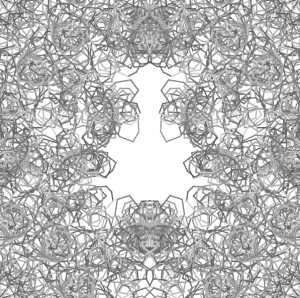
