(click on shapes to activate)
This project I was inspired by some of the shapes shown as examples on the project description. I thought the round shapes were really cute and I could imagine them as a pattern. I made it so that each shape you could manipulate individually by clicking on them. This way there could be more options for what you could create based on the mouse.
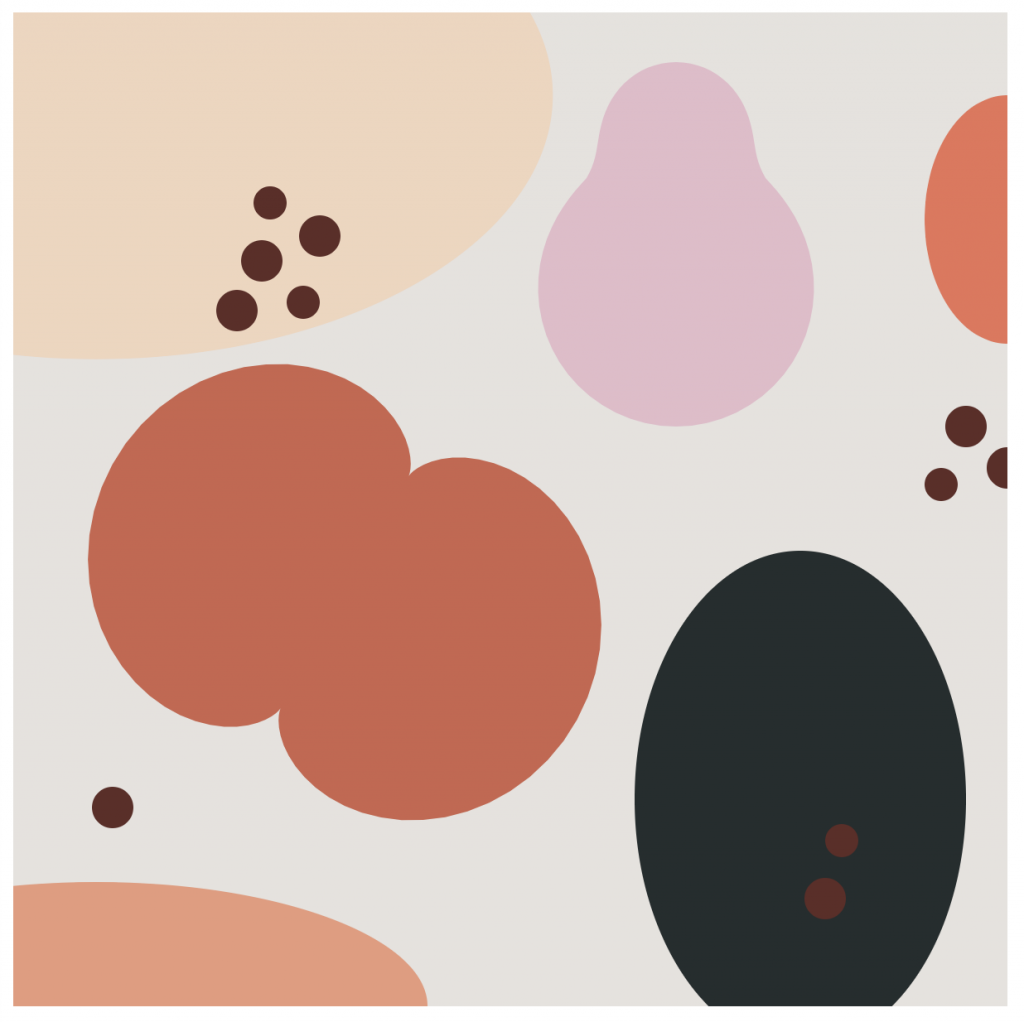
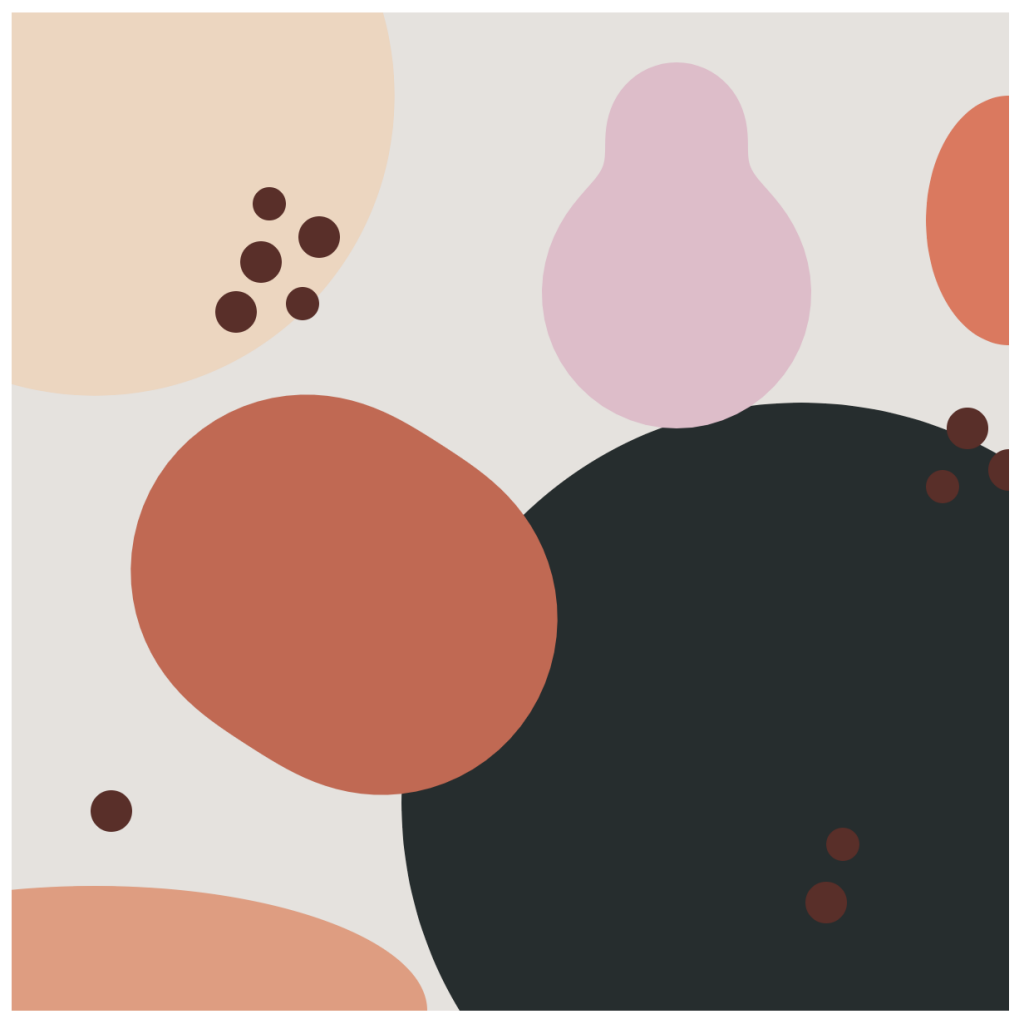
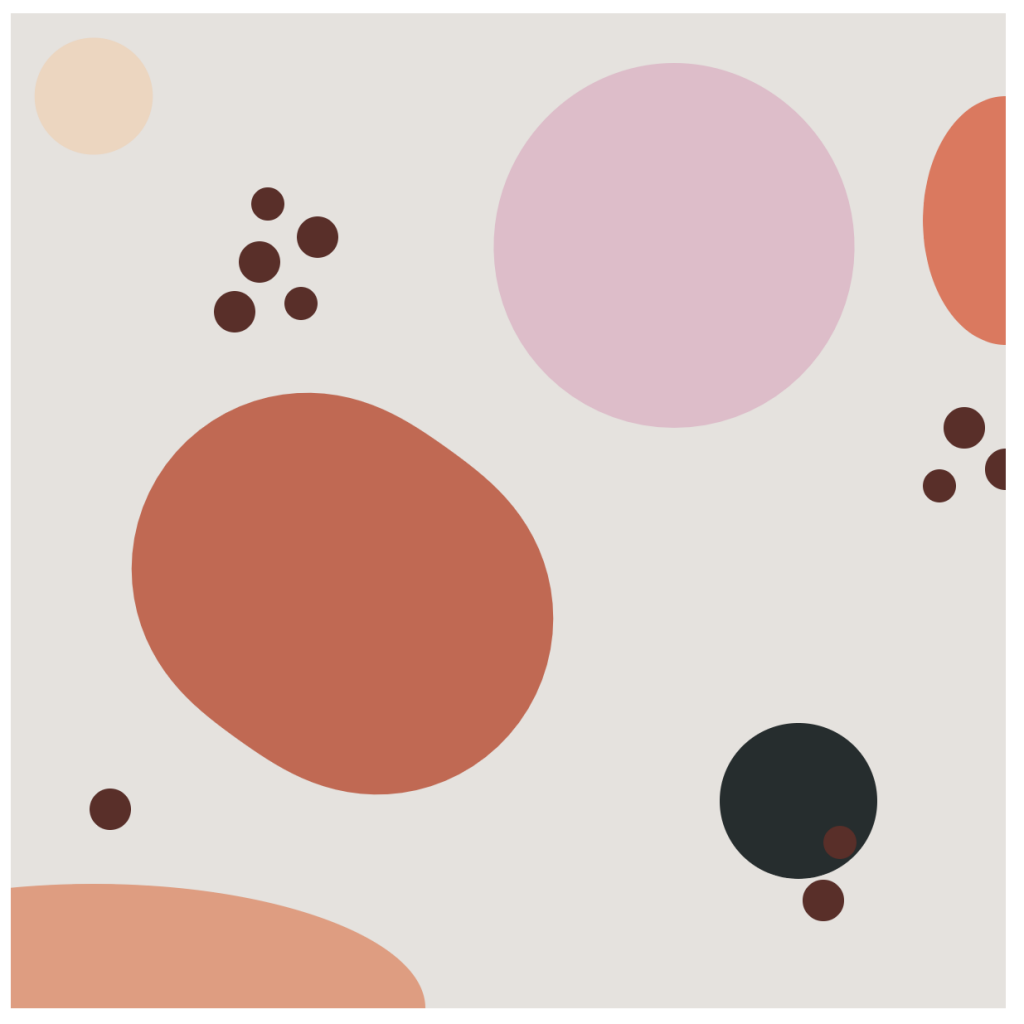
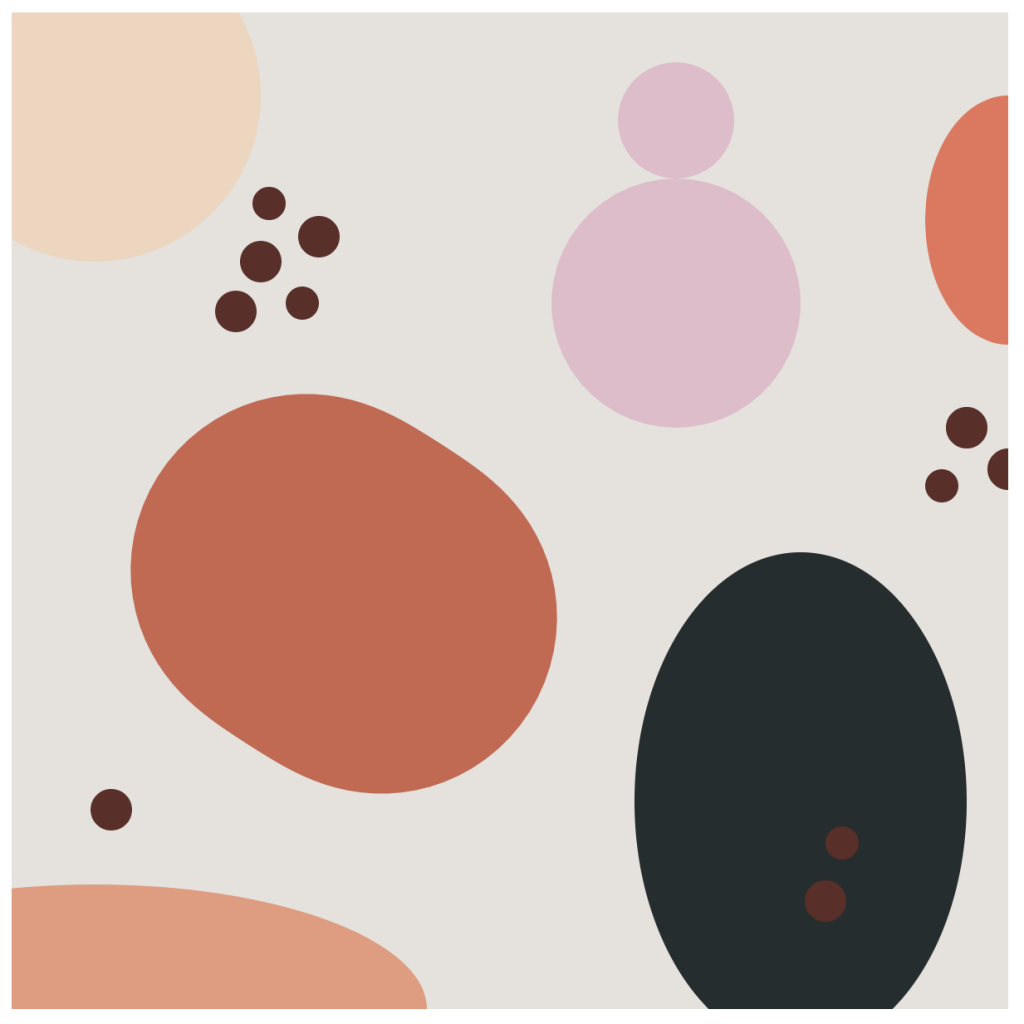
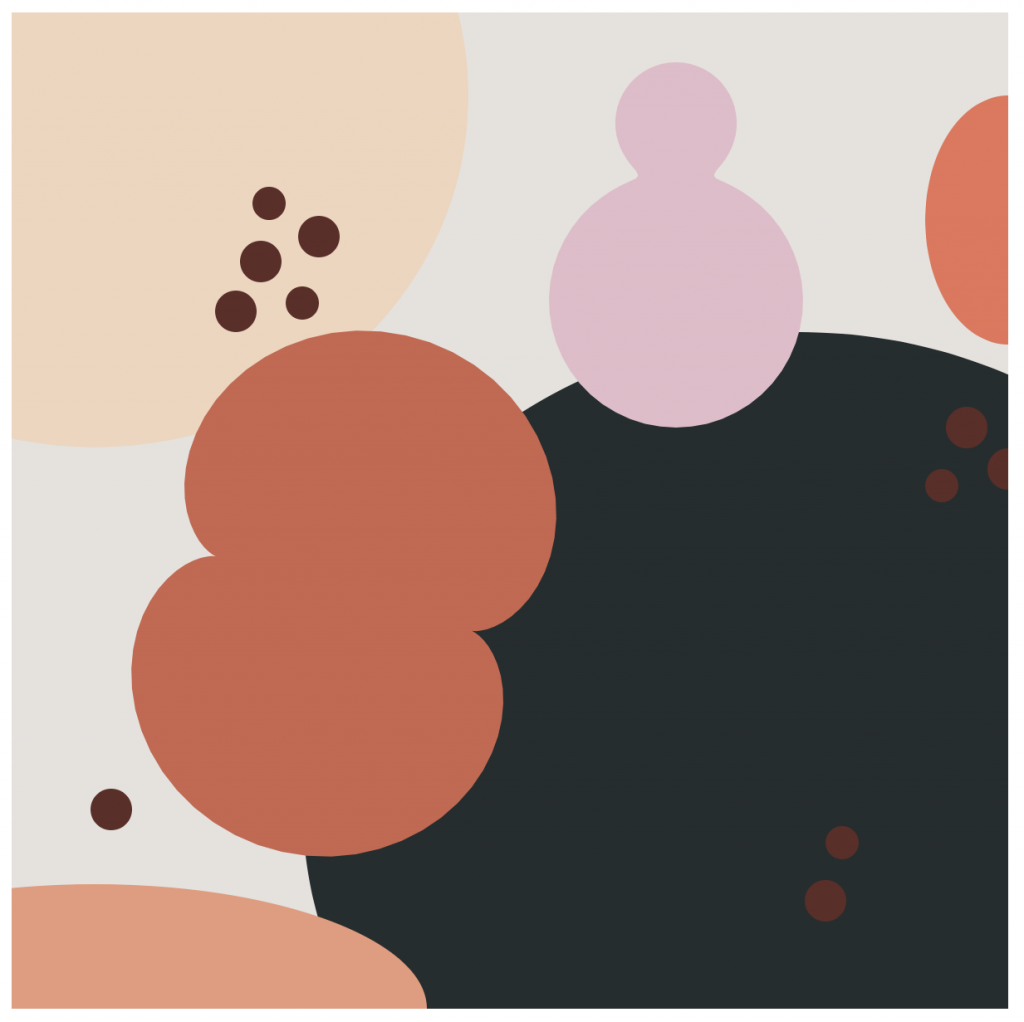
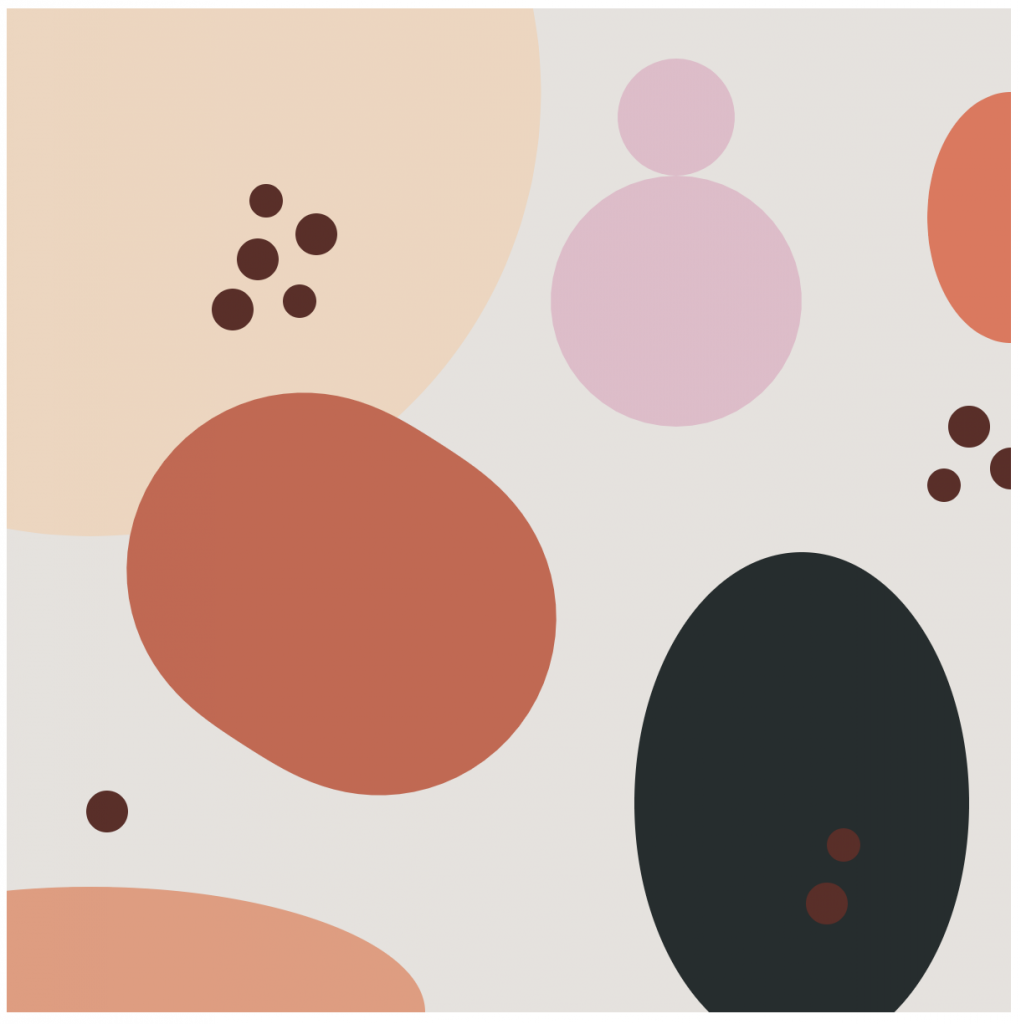
//Margot Gersing - Project 7 - mgersing@andrew.cmu.edu- section E
var oneX = 50; //for mouse press
var oneY = 50;
var act1 = false; //peach shape 'activation'
var twoX = 200; //for mouse press
var twoY = 350;
var act2 = false; //rusty orange shape 'activation'
var threeX = 400; //for mouse press
var threeY = 100;
var act3 = false; //pink shape 'activation'
var fourX = 500; //for mouse press
var fourY = 500;
var act4 = false; //gray shape 'activation'
var nPoints = 100;
function setup() {
createCanvas(600,600);
frameRate(10);
}
function draw(){
background(230, 226, 222);
noStroke();
one();
four();
two();
three();
//filler shapes (static)
fill(233, 154, 123);
ellipse(50, 600, 400, 150);
fill(233, 115, 88);
ellipse(600, 125, 100, 150);
fill(95, 44, 39);
ellipse(150, 150, 25, 25);
ellipse(175, 175, 20, 20);
ellipse(135, 180, 25, 25);
ellipse(185, 135, 25, 25);
ellipse(155, 115, 20, 20);
ellipse(500, 500, 20, 20);
ellipse(490, 535, 25, 25);
ellipse(600, 275, 25, 25);
ellipse(575, 250, 25, 25);
ellipse(560, 285, 20, 20);
ellipse(60, 480, 25, 25);
}
function one(){
//peach shape
var w1 = 200;
var h1 = 200;
//click to activate otherwise static
if(act1){
w1 = mouseX * .75;
h1 = mouseY * .75;
} else{
w1 = 200;
h1 = 200;
}
fill(240, 213, 189);
ellipse(50, 50, w1, h1);
}
function two(){
//epitrochoid
var x;
var y;
var a = 80.0;
var b = a / 2.0;
var h2 = constrain(100 / 8.0, 0, b);
var ph2 = 100 / 50.0;
//click to activate otherwise static
if(act2){
h2 = constrain(mouseX / 8.0, 0, b);;
ph2 = mouseX / 50.0;
} else{
h2 = constrain(100 / 8.0, 0, b);
h1 = 100 / 50.0;
}
fill(205, 100, 77);
beginShape(); //drawing shape
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x = (a + b) * cos(t) - h2 * cos(ph2 + t * (a + b) / b);
y = (a + b) * sin(t) - h2 * sin(ph2 + t * (a + b) / b);
vertex(x + 200, y + 350);
}
endShape(CLOSE);
}
function three(){
//cranioid
var x;
var y;
var r;
var a = 40.0;
var b = 10.0;
var c = 100.0;
var p = constrain((width/2), 0.0, 1.0);
var q = constrain((height/2), 0.0, 1.0);
//click to activate otherwise static
if(act3){
var p = constrain((mouseX / width), 0.0, 1.0);
var q = constrain((mouseY / height), 0.0, 1.0);
} else{
var p = constrain((width/2), 0.0, 1.0);
var q = constrain((height/2), 0.0, 1.0);
}
fill(227, 187, 202);
beginShape(); //drawing shape
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
r =
a * sin(t) +
b * sqrt(1.0 - p * sq(cos(t))) +
c * sqrt(1.0 - q * sq(cos(t)));
x = r * cos(t);
y = r * sin(t);
vertex(x + 400, y + 100);
}
endShape(CLOSE);
}
function four(){
//gray shape
var w4 = 200;
var h4 = 300;
//click to activate otherwise static
if(act4){
w4 = mouseX;
h4 = mouseY;
} else{
w4 = 200;
h4 = 300;
}
fill(37, 45, 46);
ellipse(475, 475, w4, h4);
}
function mousePressed(){
//if clicked within in defined distance then activation state is...
//changed from true to false so the mouseX and mouseY will now take over
var d = dist(oneX, oneY, mouseX, mouseY);
if(d < 200){
act1 = !act1;
}
var d2 = dist(twoX, twoY, mouseX, mouseY);
if(d2 < 100){
act2 = !act2;
}
var d3 = dist(threeX, threeY, mouseX, mouseY);
if(d3 < 100){
act3 = !act3;
}
var d4 = dist(fourX, fourY, mouseX, mouseY);
if(d4 < 100){
act4 = !act4;
}
}