//Minjae Jeong
//Section B
//minjaej@andrew.cmu.edu
//Project-04
function setup() {
createCanvas(400,300);
background("black");
strokeWeight(0.1);
}
function draw() {
stroke(mouseX / 3, mouseY / 2, 255); //diagonal line
line(0, 300, 400, 0);
for (i = 0; i < 400; i += 10) { //top left purple curve
stroke(204, 153, 255);
line(0, 300 - i, i, 0);
}
for (i = 0; i < 400; i += 10) { //bottom right emerald curve
stroke(0, 255, 255);
line(i, 300, 400, 300 - i);
}
//center
for (i = 0; i <= 200; i += 20) { //1st quadrant
stroke(153, 102, 255);
line(200, i - 50, 200 + i, 150);
}
for (i = 0; i <= 200; i += 20){ //3rd quadrant
stroke(0, 255, 204);
line(i, 150, 200, 150 + i);
}
}
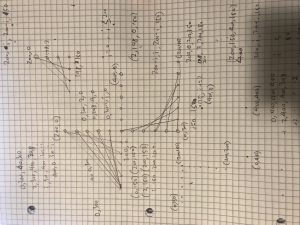
First it was very confusing to integrate for loops and line function to create string art. But after understanding the basics, It was a very fun and creative project to work on.