/* Mari Kubota
49-104 Section D
mkubota@andrew.cmu.edu
Assignment 6
*/
function setup(){
createCanvas(400,400);
}
function draw(){
background(178,208,166);
noStroke();
//creates vars to call on time
var sec= second();
var min= minute();
var hr= hour();
var size= 200;
//Mapping time to shape components
var mappedSec = map(sec, 0,59, width/2-size,width/2+size);
var mappedMin = map(min,0, 59, 0, size);
var mappedHr = map(hr, 0, 23, 270, 630);
//blue circle
fill(178,208,220);
ellipse(width/2, height/2, size, size);
//Background color circle ellipsing blue circle (seconds)
fill(178,208,166);
ellipse(mappedSec, height/2, size, size);
//white circle (minutes)
fill(225);
ellipse(width/2, height/2, mappedMin, mappedMin);
//yellow arc (hours)
angleMode(DEGREES);
stroke(255,248,175);
noFill();
strokeWeight(10);
arc(width/2, height/2, size+50, size+50, 270, mappedHr);
}
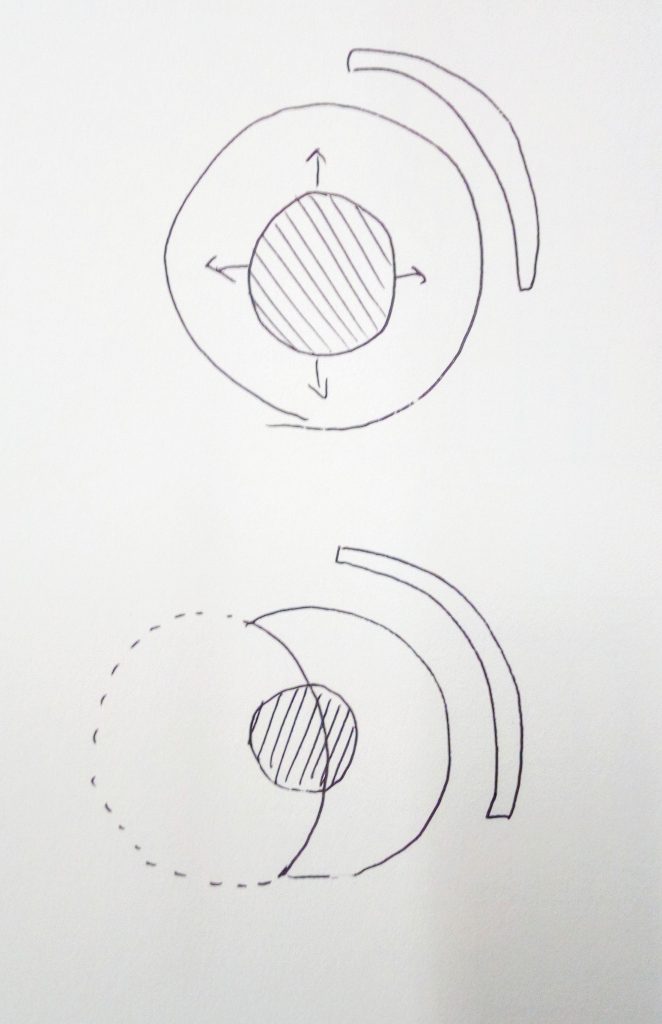
In this project I was inspired by the waxing and waning of the moon. Every second shifted a background colored circle over the blue circle to give an illusion of an eclipse. The center white circle grows bigger every minute. And the yellow arc on the outside grows longer every hour in a 24 hour period. The most challenging part of this assignment was adjusting the time variables to the right numbers and components.