/*
Mari Kubota
Section D
mkubota@andrew.cmu.edu
Assignment-09
*/
var lines = []; // Empty array to store ellipses
// Load image
function preload() {
underlyingImage = loadImage("https://i.imgur.com/23aauRel.jpg");
}
function setup() {
createCanvas(550, 420);
underlyingImage.loadPixels();
}
// Determines the properties
function makelines() {
var ix = constrain(floor(this.x), 0, width-1);
var iy = constrain(floor(this.y), 0, height-1);
var theColorAtLocationXY = underlyingImage.get(this.x, this.y); //gets the color value of the image at (x,y)
var linethickness= 5;//thickness of lines
fill(theColorAtLocationXY) // Fills the ellipse with the color of the image at (x,y)
noStroke();
ellipse(this.x, this.y, linethickness); // Draws the ellipse at (x,y) with the width and the height dimension of 'ballsize' which is a random number between 2 and 8
}
// Makes the lines move
function linespeed() {
this.x += this.dx; // MouseY will be later assigned as 'y'
}
// function to create properties of ellipse to be called on later
function drawPortrait(originalx, originaly, originaldx) {
p = {x: originalx,
y: originaly,
dx: originaldx,
speed: linespeed,
lines: makelines,
}
return p;
}
//draws the lines
function draw() {
newLines = []; // Creates an empty array for new lines
for (var i = 0; i < lines.length; i++) {
var p = lines[i];
p.speed(); //returns the function speed from drawPortrait
p.lines(); //returns the function balls from drawPortrait
newLines.push(p);
}
lines = newLines;
}
// When the mouse is moved it replaces the originalx, originaly, and originaldx values with mouseX, mouseY and random(-20,20) and make the drawPortrait function actually happen and to be stored in the newball array
function mouseMoved() {
var newLines = drawPortrait(mouseX, mouseY, random(-20, 20));
lines.push(newLines);
this.x += random(this.x-3, this.x+3);
}
For this project I used a picture of me from over the summer when I was learning how to drive. The image appears when you mouse over the picture and the picture appears with horizontal strips which were made using ellipse().
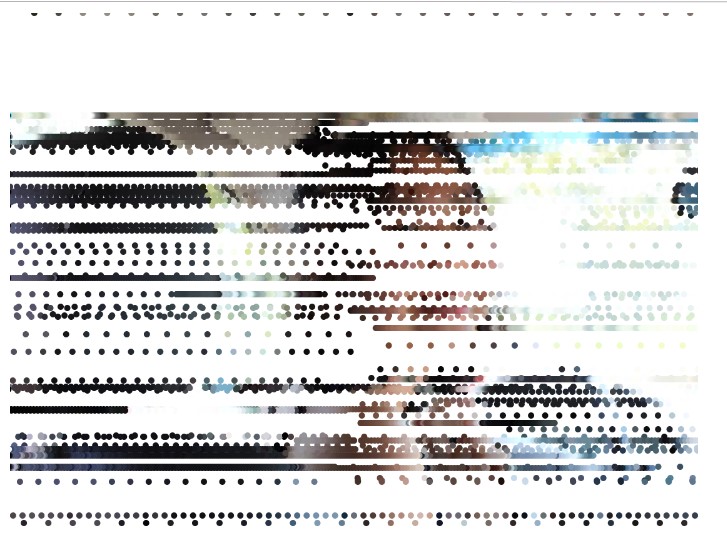
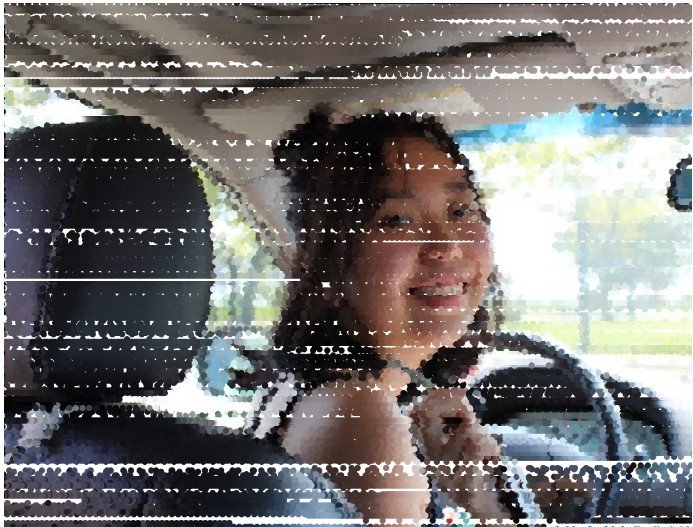