//Steven Fei
//zfei@andrew.cmu.edu
//project 11
//section-A
var stars = [];
function setup() {
createCanvas(480, 480);
frameRate(8);
//set the initial display of the stars
for(var i = 0; i<8; i++){
var sx = random(width);
var sy = random(50, height/3);
stars[i] = makeStars(sx, sy);
}
}
function draw() {
background("black");
//create the terrain in the far back of the canvas
var terrain3 = terrain(30, 2, height/5, height * 3/4, 3);
//create the terrain in the middle of the canvas
var terrain2 = terrain(80, 2, height/3, height * 3/4, 2);
//create the terrain in the front of the canvas
var terrain1 = terrain("white", 2, height/2, height * 3/4, 1);
//create the blue lake at the bottom of the canvas
noStroke();
fill(0,33,41);
rect(0, height * 4/5, width, height);
//create trees
for (var x = 0; x < width; x+=5){
var orange = random(80,150);
stroke(240, orange, 80);//define the color of the tree to draw
strokeWeight(1.2);
push();
translate(x * random(5,10), height * 4/5);
drawTree(0, random(5,15), int(random(3,6)));//calling the function to draw the tree
pop();
}
//update star by showing the stars and the moving positions of the stars
updateStar();
//only keep the stars that are still on the canvas
removeStar();
//add new stars under a certain probability
addStars();
}
//showing the star positions
function updateStar(){
for (var i = 0; i<stars.length; i++){
stars[i].move();
stars[i].display();
}
}
//only keep the stars on the canvas
function removeStar(){
var starKeep = [];
for ( var i = 0; i < stars.length; i++){
if(stars[i].x > 0){
starKeep.push(stars[i]);
}
}
stars = starKeep;
}
//add more stars by chance
function addStars(){
var starProbability = 0.01;
if(random(0,1) < starProbability){
stars.push(makeStars(width,random(50,height/3)));
}
}
//defining the moving speed of the star
function starMove(){
this.x += this.speed;
}
//displaying the star by drawing the circle
function starDisplay(){
fill("white");
noStroke();
push();
translate(this.x, this.y);
circle(0,0,this.size);
pop();
}
//main function to make the star
function makeStars(birthX, birthY){
var str = { x: birthX,
y: birthY,
speed: -1.5,
size: random(5,15),
move: starMove,
display: starDisplay};
return str;
}
//making the terrain
var terrainSpeed = 0.0005;
var terrainDetail = 0.005;
function terrain(color,strokeweight,min,max,noiseseed){
var verticesX = [];//array to append values for x coordinates
var verticesY = [];//array to append values for y coordinates
noiseSeed(noiseseed);//decide the pseudo random number seed
stroke(color);//the color to choose to draw the terrain
strokeWeight(strokeweight);//the strokeweight to choose to draw
noFill();
beginShape();
for ( var x = 0; x < width; x++){
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 1, min, max);
vertex(x,y);//generating the terrain points
verticesX.push(x);
verticesY.push(y);
if(verticesX.length > width){
verticesX.shift();
verticesY.shift();
}
}
for ( var j = 0; j < verticesX.length; j++){
line(verticesX[j], verticesY[j], verticesX[j],height);//add vertical coloring to the terrain
}
endShape();
}
//function to draw trees by defining depth(number of branches), len(branch length), seed(upper limit of the branch counts)
function drawTree(depth, len, seed){
if(depth < seed){
rotate(radians(-10));
drawBranch(depth,len, seed);
rotate(radians(20));
drawBranch(depth,len, seed);
}
}
function drawBranch(depth, len, seed){
line(0,0,0,-len);
push();
translate(0,-len);
drawTree(depth + 1, len, seed);
pop();
}
For this project, I was intended to create a serene atmosphere by the lake. The mountains are majorly created in 3 layers with different shades to represent a sense of depth and distance. Symbolic maple trees were drawn in different saturations of orange and different sizes and branch counts to have a “realistic” impression of the diverse range of the trees. The circular stars are moving in the upper part of the canvas to strengthen the sense of moving. By setting different moving speed of those different objects, I was aiming to deepen a spatial feeling in the visual hierarchy. The maple trees were moving fastest because they are relatively in the very front of the canvas, while the mountains are constantly moving slower, and the stars are the slowest. The project gives me some chances to make different objects and functions and use different strategies to fulfill those elements through building a connection between the draw function and the other specific functions I make for the elements.
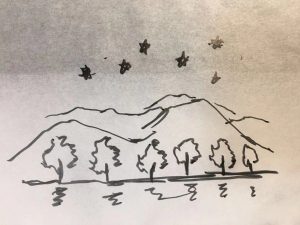