//Jai Sawkar
//jsawkar@andrew.cmu.edu
//Section C
//Project 11: Generative Landscape
var objectSpeed = 0.00005; //speed of objects
var mountainDetail = 0.007; //threshold of variance in mountains
var waterDetail = 0.002 //threshold of variance in water
var xMove = 520 //initial point
var spec = []; //snow
function setup() {
createCanvas(480, 480);
for (var i = 0; i < 100; i++){ //setting up for loop that determines bounds of snow
var specX = random(0, width); //determines x
var specY = random(0, height/2); //determines y
spec[i] = makeSpec(specX, specY); //uses i from for loop
}
print(spec);
}
function draw() {
background(249, 233, 161);
drawMountains(); //calls mountain object
displaySpec(); //calls the snow
fill(228, 118, 47);
noStroke();
ellipse(width/2, height/2, 95);
// calls water object
drawWater();
//calls text
drawText();
xMove -= .1 //moves text
if (xMove < -90) { //makes it so that when text goes off screen, it reappears
xMove = 480
}
}
function drawText() {
//makes text of album
fill(0)
textFont('Courier');
textSize(24);
textAlign(CENTER);
text('K A U A I', xMove, 30)
textSize(8);
text('WITH JADEN SMITH AS', xMove, 40)
text('"THE BOY"', xMove, 50)
textSize(24);
text('G A M B I N O', xMove, 70)
}
function drawMountains() { //makes generative mountain
push();
beginShape();
fill(90, 57, 27);
vertex(0,height/2 + 50); //makes height of vertex of mountain
for (var x = 0; x < width; x++) {
var t = (x * mountainDetail) + (millis() * objectSpeed); //allows object to be generative based on time
var y = map(noise(t), 0, 1, 0, height); //remaps values to fit in desired area
vertex(x, y-15); //final vertex
}
vertex(width, height/2 + 50); //final vertex out of for loop
endShape();
pop();
}
function makeSpec(specX, specY){ //makes object for snow
var makeSpec = {x: specX,
y: specY, //calls y coordinate
draw: drawSpec} //allows it to be called to draw
return makeSpec;
}
function drawSpec(){
fill('WHITE')
ellipse(this.x, this.y, 3) //makes circles
}
function displaySpec(){
for(i = 0; i < spec.length; i++){
spec[i].draw(); //allows spec to be displayed
}
}
function drawWater(){ //makes water
fill(51,107,135,255);
push()
beginShape();
noStroke();
fill(170, 210, 241);
for (var x = 0; x < width; x++) { //makes height of vertex of mountain
var t = (x * waterDetail) + (millis() * objectSpeed); //allows object to be generative based on time
var y = map(noise(t), 0,1, height/2 - 20, height/2 + 50); //remaps values to fit in desired area
vertex(0, 900); //inital vertex
vertex(1000,900); //final vertex
vertex(x, y);
}
endShape();
pop()
}
This week, I used the Generative Landscape project to create an album cover of one of my favorite artists, Childish Gambino. I began with the initial cover, which is a Hawaiian sunset abstracted into three objects, the sky, the water, and a semi-circle. I first created the water to be generated randomly, followed by adding mountains in the back to create depth. Moreover, I used a javaScript Object to create snow! It was used to juxtapose the calm nature of Kauai with Gambino’s other, more rowdy album, Because the Internet. Lastly, I made the title for the album slide in & out of the landscape.
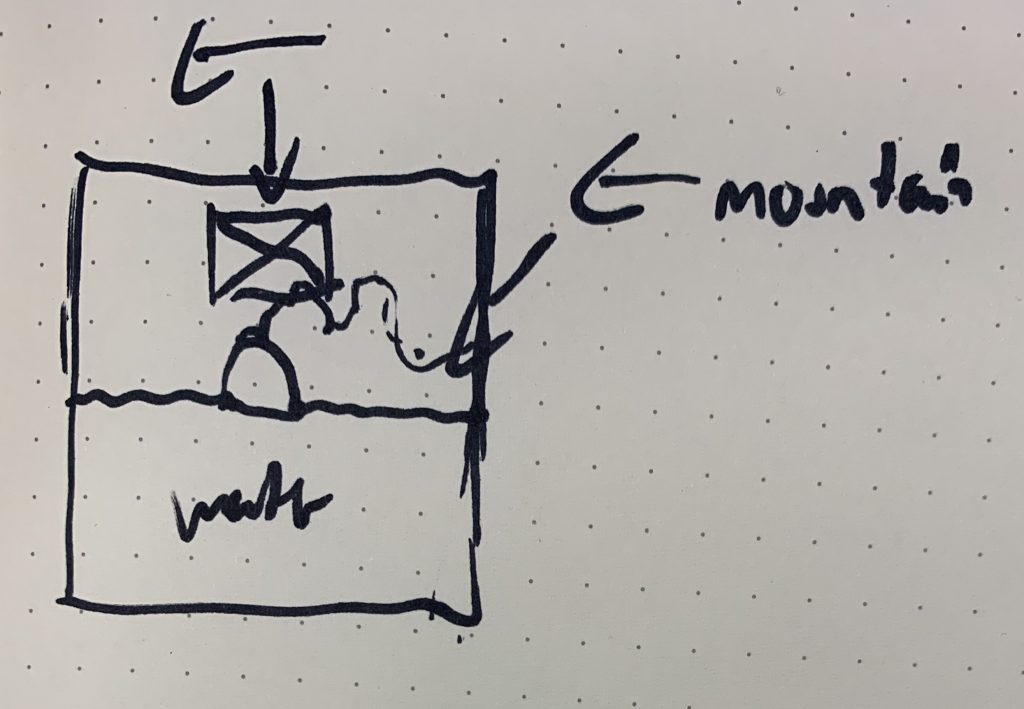
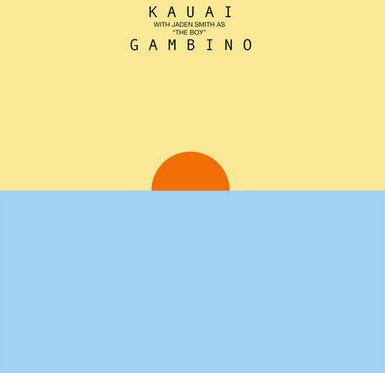