//Ghalya Alsanea
//Section B
//galsanea@andrew.cmu.edu;
//Project-05
var density = 20; //grid density
var tile;
function setup() {
createCanvas(600, 600);
noLoop();
tile = width / density; //find tile dimension
}
function draw() {
background(200);
//create a grid
for (var gridY = 0; gridY <= density; gridY++) {
for (var gridX = 0; gridX <= density; gridX++) {
//random color generator
array = ["DarkSalmon", "DarkSeaGreen", "LightCoral", "MediumAquaMarine"];
colorSeed = int(random(0, 4));
var color = array[colorSeed];
stroke (color);
strokeWeight(1);
//define the grid's X and Y positions
var posX = tile * gridX;
var posY = height / density * gridY;
//randomizer
var toggle = int(random(0, 3));
//randomize which direction the diagonal is drawn
//whether it's (\) or (/)
if (toggle == 0) {
line(posX, posY, posX + tile, posY + height / density);
}
if (toggle == 1) {
strokeWeight(2);
line(posX, posY + tile, posX + height / density, posY);
}
// if it's not / or \ then draw a dot
if (toggle == 2) {
noStroke();
fill(color);
circle(posX, posY, tile / 4)
}
// if it's not / or \ or a dot then it becomes a torus flower
else {
noFill();
stroke(color);
strokeWeight(1);
circle(posX, posY, tile / 3);
push();
translate(posX, posY);
for (i = 0; i < 6; i++) {
fill(255, 100);
circle(tile / 6, 0, tile / 3);
rotate(radians(60));
}
pop();
}
}
}
}
function mousePressed() {
//redraw the wallpaper when you click your mouse
redraw();
}
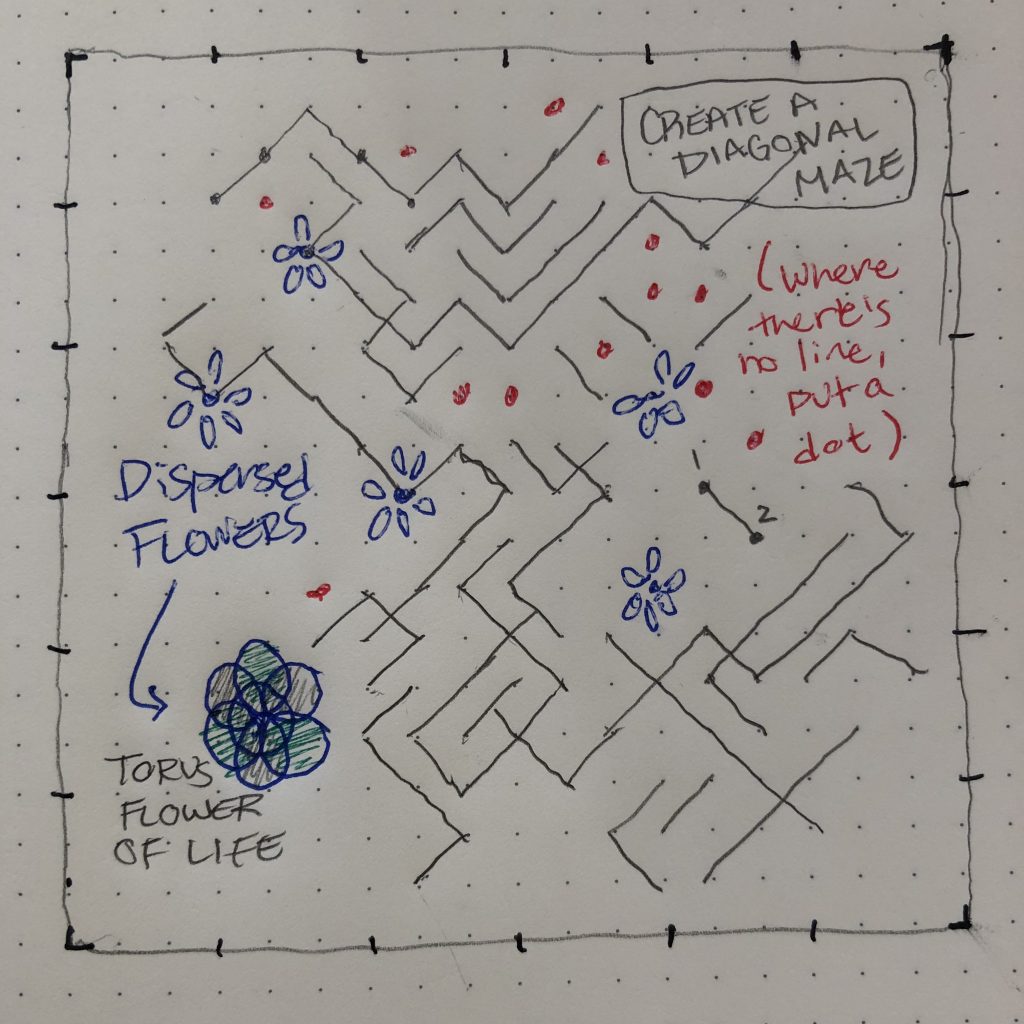
I started by creating a grid and then creating a maze like pattern based on a random toggle (i.e. if the line goes \ or /). I wanted to create a more fun pattern so I added to the conditional to add a flower or a dot in the places where there was no line. I was challenging myself to find a balance between structure and randomness. There’s also a random color associated with each graphic that is placed in the grid. Hence, every time you click your mouse a completely new wallpaper is drawn unlike the one before.