// Timothy Liu
// 15-104 Section C
// tcliu@andrew.cmu.edu
// OpenEnded-06
// variables defining the sapling + base characteristics
var leaf = 12;
var stem = 50;
var stemW = 4;
var base = 30;
var leafMax = 16;
var leafMin = 11;
var leafChange = 0.1;
var leafH;
function setup() {
createCanvas(480, 200);
frameRate(20);
}
function draw() {
noStroke();
// time variables; militaryH uses a 24 hr scale, and H uses a 12 hr scale with the modular
var militaryH = hour();
var H = hour() % 12;
var M = minute();
var S = second();
var mM = map(M, 0, 59, 0, 50); // mapping mM so it's constrained between 0 and 50, the height of each sapling
// these variables are used later when determining sky color and when it should change
var mH1 = militaryH + 1;
var H3 = H - 3;
// defining where leafH is
leafH = height / 2;
// the following if statements make the sky change color based on the time, indicating am vs. pm:
// ex: if there are 2.5 saplings grown, and the sky is dark blue, it must be 2.5am because it's still dark outside.
// if it's from 12am - 8am, the sky slowly gets brighter every hour
if (militaryH < 8) {
fill(0 + (15 * mH1), 15 + (20 * mH1), 100 + (15 * mH1));
rect(0, 0, width, 2 * height / 3);
// if it's 8am - 4pm, the sky stays consistently bright out
} else if (militaryH >= 8 & militaryH < 16) {
fill(120, 175, 220);
rect(0, 0, width, 2 * height / 3);
// if it's 4pm - 12pm, the sky slowly gets darker every hour
} else {
fill(120 - (15 * H3), 171 - (20 * H3), 220 - (15 * H3));
rect(0, 0, width, 2 * height / 3);
}
// base ground color
fill(191, 148, 115);
rect(0, 2 * height / 3, width, height / 3);
// this for loop draws all of the 12 saplings and the mounds of dirt they grow out of
for (var a = 0; a < 12; a++) {
// dirt mounds around base of saplings
fill(171, 125, 91);
ellipse((a + 1) * width / 13 + (stemW / 2), height / 2 + stem, base, base / 2);
// this if statement draws all the saplings that are already fully grown
if (a < H) {
fill(84, 61, 40);
ellipse((a + 1) * width / 13 + (stemW / 2), height / 2 + stem, 8, 4); // shadow at base of sapling
fill(63, 209, 0); // sapling color
rect((a + 1) * width / 13, height / 2, stemW, stem);
arc(((a + 1) * width / 13) - (stemW), leafH, leaf, leaf / 2, 0, 5 * PI / 4, CHORD);
arc(((a + 1) * width / 13) + (2 * stemW), leafH, leaf, leaf / 2, 7 * PI / 4, PI, CHORD);
}
// this if statement draws the sapling that is currently growing
if (a == H) {
fill(84, 61, 40);
ellipse((a + 1) * width / 13 + (stemW / 2), height / 2 + stem, 8, 4); // shadow at base of sapling
fill(63, 209, 0); // sapling color
rect((a + 1) * width / 13, leafH + stem, stemW, -mM);
arc(((a + 1) * width / 13) - (stemW), leafH + stem - mM, leaf, leaf / 2, 0, 5 * PI / 4, CHORD);
arc(((a + 1) * width / 13) + (2 * stemW), leafH + stem - mM, leaf, leaf / 2, 7 * PI / 4, PI, CHORD);
}
// this if statement makes the leaves flutter/pulsate once every second, serving as a measure of S (seconds)
if (S % 2 == 0) {
leaf = min(leaf + leafChange, leafMax);
} else {
leaf = max(leaf - leafChange, leafMin);
}
}
}
I really enjoyed the concept of envisioning an abstract clock. Time in itself is such an abstract construct, so it was a fun challenge trying to represent it in an unconventional way. One of my first ideas about showing time was through plants, like bamboo, that grow at a rapid rate. I realized that I could play with this concept using plant saplings, so my abstract clock portrays a series of 12 saplings that grow and sway in the wind with the seconds, minutes, and hours in the day.
A few key things to note about my clock:
- I decided to only show 12 saplings to represent the two 12-hour halves in the day because I felt that 24 saplings would cause my clock to lose meaning and groundedness. To help represent AM vs. PM time, I made the sky change color as a function of what hour is. From 12AM — 8AM, the sky slowly changes from dark blue to light blue each hour. At 8AM, the sky is light blue, and it stays that way until 4PM. Then, from 4PM — 12AM, the sky slowly gets darker again every hour. Using this logic, the user can deduce what time it is in the day; for example, if I see that there are 3.5 saplings and the sky is dark, that means it must be 3:30AM and it’s not light out yet. If there are 3.5 saplings and it’s light out, it must be 3:30PM in the early afternoon.
- My program has the leaves of the saplings pulsating/fluttering once every second. They serve as a good way to track the number of seconds in my clock, as even though there’s no counter, it’s an easy metric to count and follow.
Below are some sketches from my ideation phase.
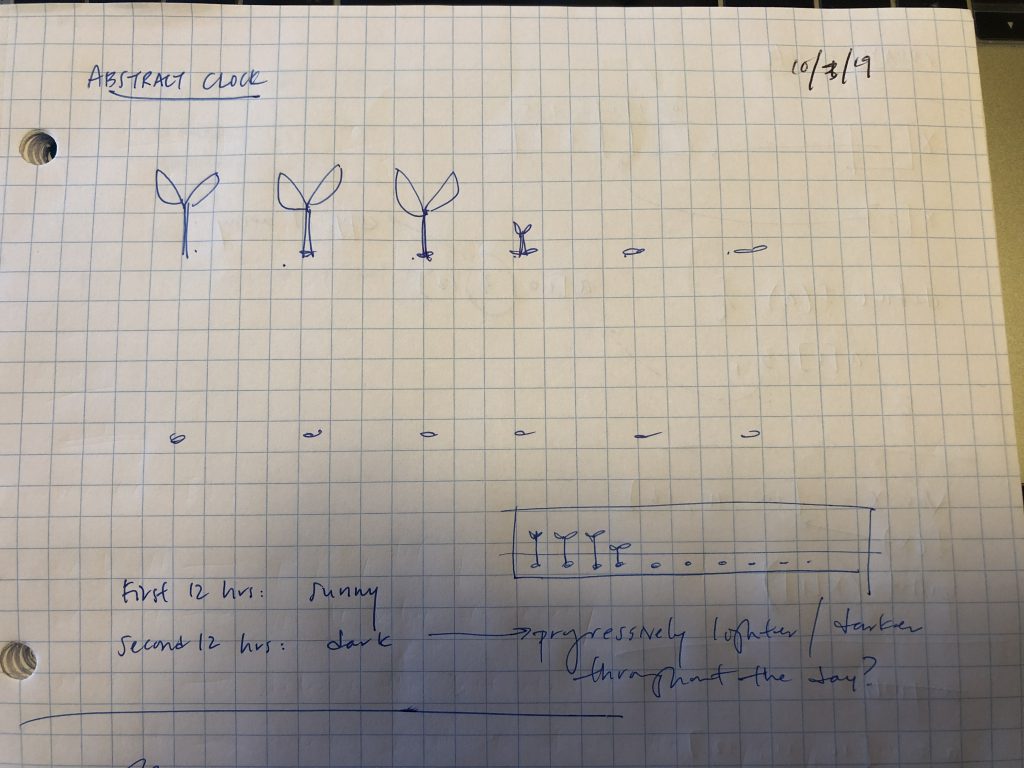