// Alec Albright
// aalbrigh@andrew.cmu.edu
// Section B
// Project 07
var nPoints = 100; // number of points used to draw curves
function setup(){
createCanvas(480, 480);
angleMode(RADIANS);
}
function draw(){
background("lightpink");
// center the shapes
push();
translate(width / 2, height / 2);
// draw all the shapes
drawHippopede();
drawEpicycloid();
drawHypotrochoid();
pop();
}
// draws Hippopede
function drawHippopede() {
var x; // x coordinate of vertex
var y; // y coordinate of vertex
var r; // polar coordinate
var a = mouseX / 2 // main parameter of the curve
var b = map(a, 0, 480, 100, 240); // circle radius
var rotation = map(mouseY, 0, 480, 0, TWO_PI); // amount of rotation
// thickness of line proportional to the circle radius
strokeWeight(b / 5);
stroke("white");
noFill();
// rotate shape
push();
rotate(rotation);
// start drawing the shape, one point at a time
beginShape();
for(var i = 0; i < nPoints; i++){
var t = map(i, 0, nPoints, 0, TWO_PI);
// find r (polar equation)
r = sqrt(4 * b * (a - b * sinSq(t)));
// convert to x and y coordinates
x = r * cos(t);
y = r * sin(t);
// draw a point at x, y
vertex(x, y);
}
endShape();
pop();
}
// draws hypotrochoid
function drawHypotrochoid() {
var x; // x coordinate of vertex
var y; // y coordinate of vertex
var a = map(mouseX, 0, 480, 50, 150); // radius of the interior circle
var b = 3; // radius of the petals
var h = mouseX / 10; // distance from center of interior circle
var red = map((mouseX + mouseY) / 2, 0, 480, 0, 255); // how much red
var blue = map(mouseY, 0, 480, 0, 255); // how much blue
var alpha = map(mouseX, 0, 480, 50, 255); // how opaque
var rotation = map(mouseY, 100, 300, 0, TWO_PI); // amount of rotation
strokeWeight(2)
stroke("white");
// control color and opacity with mouse location
fill(red, 0, blue, alpha);
// control rotation with mouseY
push();
rotate(rotation);
// create the shape itself
beginShape();
for(var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
// use parametric euqations for hypotrochoid to find x and y
x = (a - b) * cos(t) + h * cos((a - b) / b * t);
y = (a - b) * sin(t) - h * sin((a - b) / b * t);
// draw a point at x, y
vertex(x, y)
}
endShape(CLOSE);
pop();
}
// draws an epicycloid
function drawEpicycloid() {
var x; // x coordinate of vertex
var y; // y coordinate of vertex
var a = map(mouseX, 0, 480, 50, 200); // radius of interior circle
var b = map(mouseY, 0, 480, 10, 50); // radius of petals
var blue = map((mouseX + mouseY) / 2, 0, 480, 0, 255); // how much blue
var red = map(mouseY, 0, 480, 0, 255); // how much red
var rotation = map(mouseY, 100, 300, 0, TWO_PI); // how muhc rotation
// control color with mouse location
strokeWeight(10)
stroke(red, 0, blue);
// control rotation with mouse location
push();
rotate(rotation);
// start drawing shape
beginShape();
for(var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
// find coordinates using epicycloid parametric equations
x = (a + b) * cos(t) - b * cos((a + b) / b * t);
y = (a + b) * sin(t) - b * sin((a + b) / b * t);
// draw a point at x, y
vertex(x, y);
}
endShape();
pop();
}
// defines sin^2 using trigonometric identities
function sinSq(x) {
return((1 - cos(2 * x)) / 2);
}
In order to put together this project, I started with a simple curve, the hippopede, and began to implement increasingly more complex ones. Once I was able to draw the three curves (hippopede, hypotrochoid, and epicycloid), I started mapping various features of the curves to things like color, transparency, stroke weight, and rotation until I was satisfied with the results. Particularly, the color mapping was interesting to me because I utilized the average of the mouseX and mouseY coordinates in order to determine some aspects of color like the amount of red in the hypotrochoid and the amount of blue in the epicycloid. This allowed me to have more freedom to speculate interesting relationships that could be created using the coordinate system.
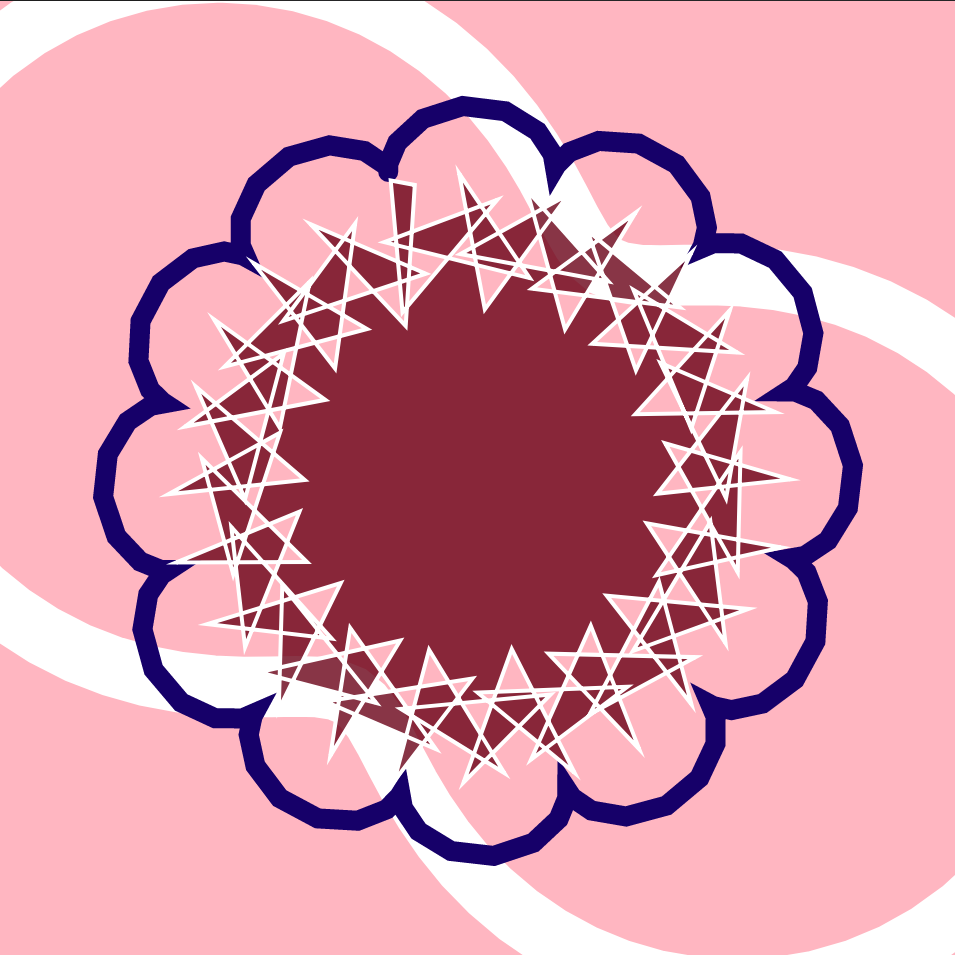
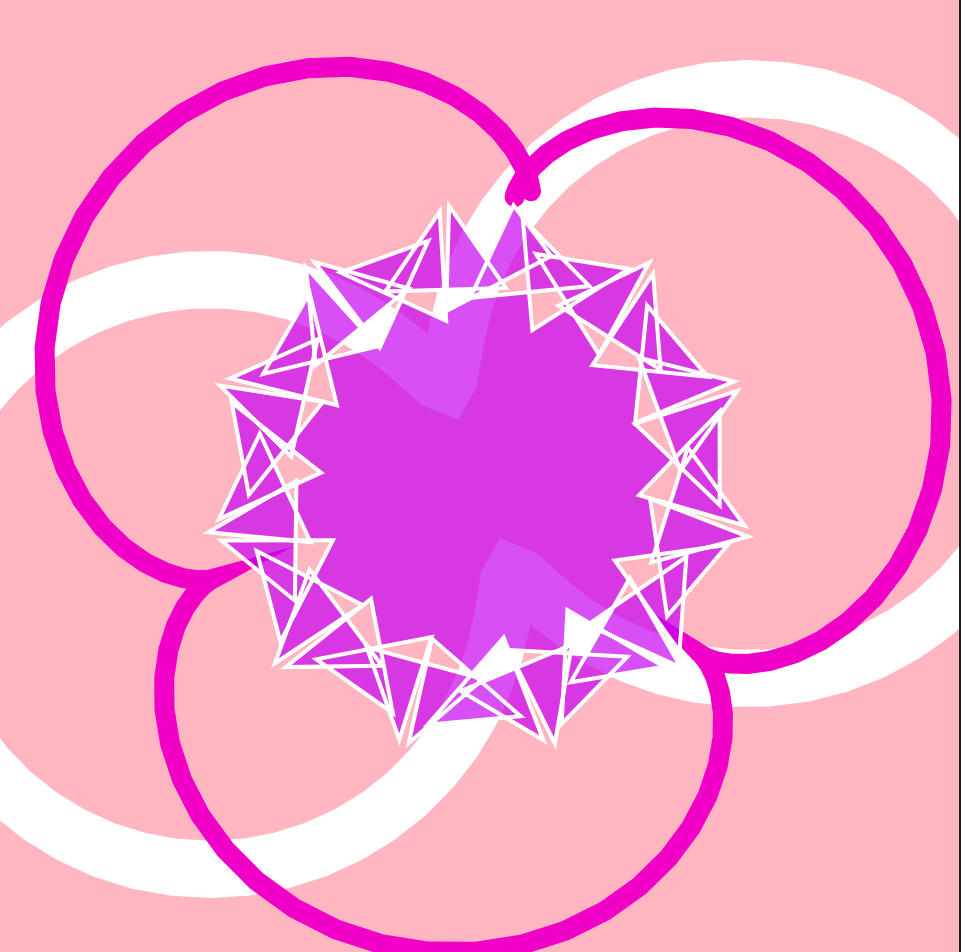
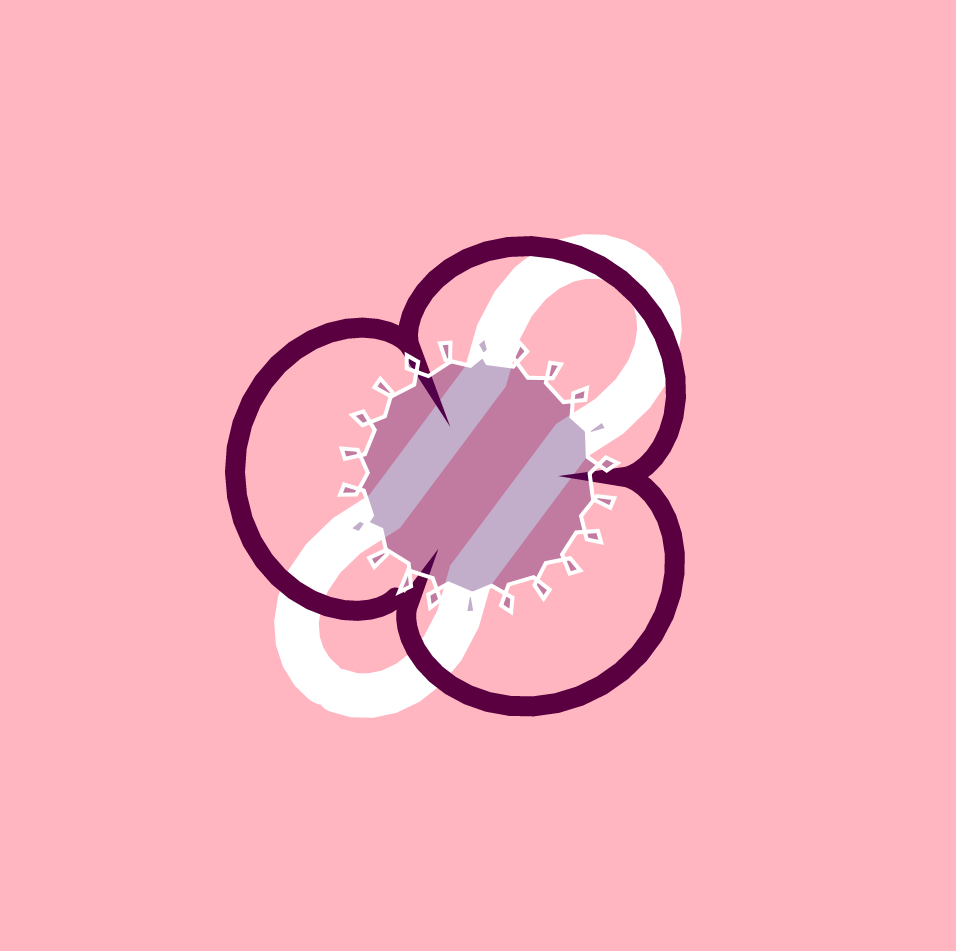
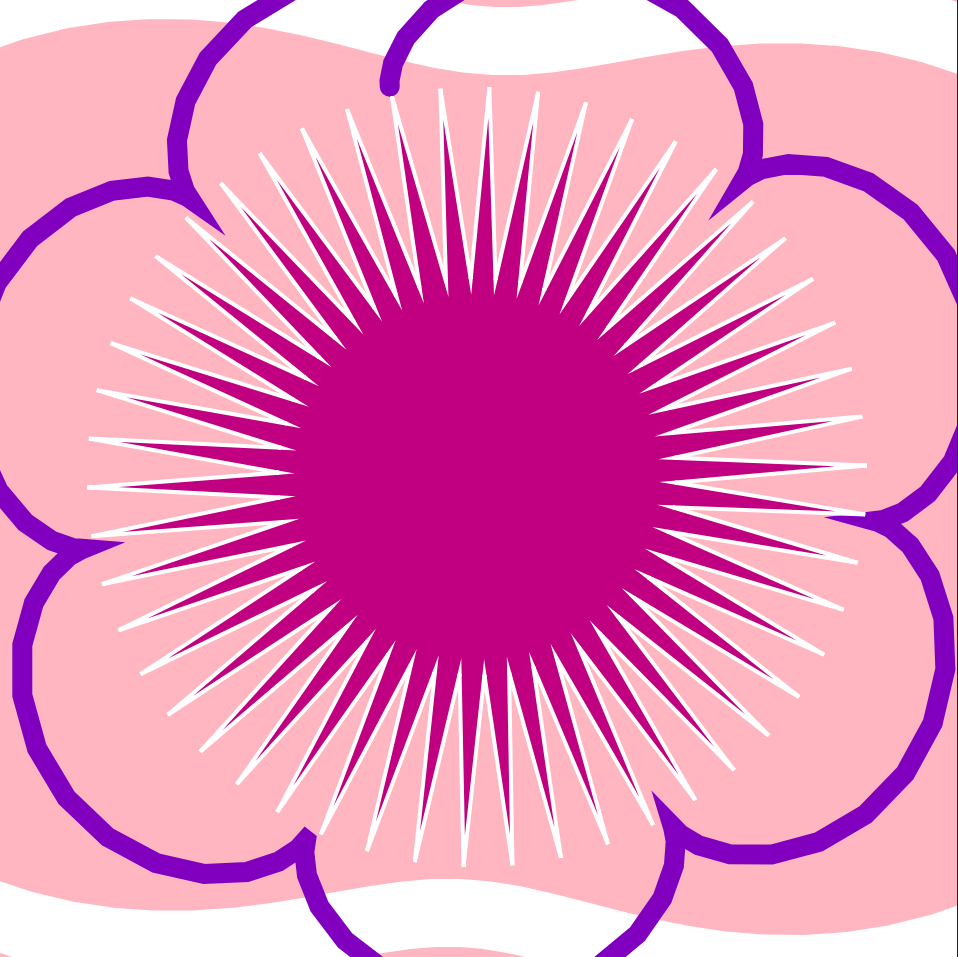