//Kristine Kim
//Section D
//younsook@andrew.cmu.edu
//Project- 07- Curves
var nPoints = 70
function setup() {
createCanvas(480, 480);
frameRate(10);
}
function draw() {
background(67, 42, 135);
//calling all the functions
//drawing all of them on the center of the canvas
push();
translate(width/2, height/2);
drawMovinglines();
drawHypotrochoid();
drawEpitrochoidCurve();
pop();
}
/*drawing the EpitrochoidCurve filled with
colors that are determined by mouseX and mouseY */
//the image that is drawing on top of everything
function drawEpitrochoidCurve(){
var x;
var y;
var r1 = 100
var r2 = r1 / mouseX;
var h = constrain(mouseX, r1 , r2)
noStroke();
fill(mouseY, 245, mouseX);
beginShape();
//for loop to draw the epitrochoidcurve
for (var i = 0; i < nPoints; i++){
var t = map(i, 0, nPoints, 0, TWO_PI);
x = (r1 + r2) * cos(t) - h * cos(t * (r1 + r2) / r2);
y = (r1 + r2) * sin(t) - h * sin(t * (r1 + r2) / r2);
vertex (x,y);
}
endShape(CLOSE);
}
/*drawing the hypotrochoid with vertexs with
colors determined by mouseY */
//the first layer, drawing behind the epitrochoidcurve and movinglines
function drawHypotrochoid(){
var x;
var y;
var r1 = 250
var r2 = r1/ mouseX;
var h = constrain(mouseY, r1, r2)
stroke(mouseY, 200, 73);
noFill();
beginShape();
//for loop for drawing the hypotrochoid form
for (var i = 0; i < nPoints; i ++){
var t = map(i, 0, nPoints, 0 , TWO_PI);
x = (r1- r2) * cos(t) + h * cos(t * (r1 - r2) / r2);
y = (r1- r2) * sin(t) + h * sin(t * (r1 - r2) / r2);
vertex(x,y)
}
endShape(CLOSE);
}
/*drawing the constiently moving/wiggling circle
the radius and fill all controlled by mouseX and mouseY
the second layer, between the epitrochoidcurve and hypotrochoid */
function drawMovinglines(){
var x;
var y;
var r1 = mouseX
fill(mouseX, mouseY, mouseX)
beginShape();
//for loop for drawing the wiggling circle
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
var x = r1 * cos(t);
var y = r1 * sin(t);
vertex(x + random(-5, 5), y + random(-5, 5));
}
endShape(CLOSE);
}
I was first very intimidated by this project because it looked so complicated and intricate, but I studying the website provided to us was very helpful. The little explanations and gif helped me understand the concept a lot. There are just so many ways we can execute this project so I struggled with starting the project, but once I drew the Hypotrochoid function, I knew how to approach this project. I played around with the quantity of nPoints, fill(), stroke(), and a lot with mouseX and mouseY until I ended up with the product that I have right now . I enjoyed creating this a lot because I love discovering new images and findings so I was pleasantly surprised every single time I changed something in my code and found different drawings.


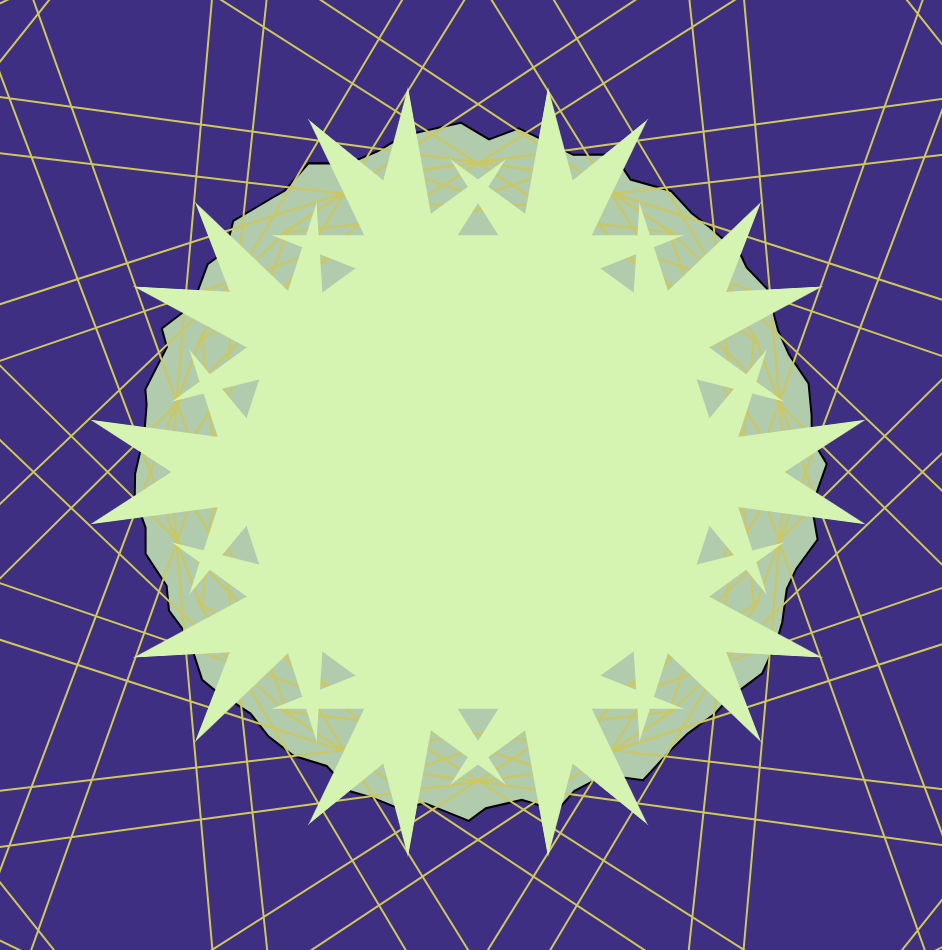
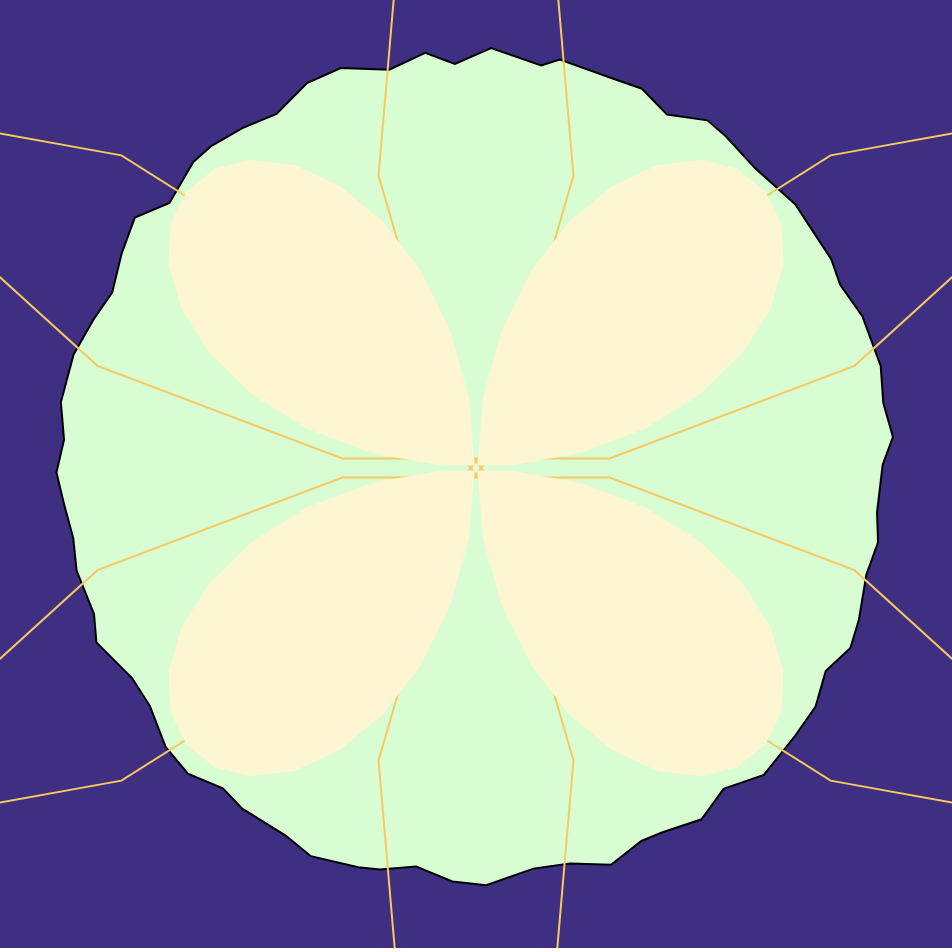