/* Cathy Dong
Section D
yinhuid@andrew.cmu.edu
Project 07 - Composition with Curves
*/
var density = 200;
function setup() {
createCanvas(480, 480);
}
function draw() {
background(0);
stroke(255);
noFill();
//call devil's curve
strokeWeight(1);
push();
translate(width/ 2, height / 2);
devilDraw()
pop();
//call curve and make its center move with mouse
strokeWeight(.5);
push();
var xDraw = constrain(mouseX, width / 5, width / 5 * 4);
var yDraw = constrain(mouseY, width / 8, height / 8 * 7);
translate(xDraw, yDraw);
hypocycloidDraw();
pop();
}
//draw hypocycloid move with mouse
function hypocycloidDraw() {
var xA;
var yA;
var aA = map(mouseX, 0, width, 0, width / 5);
var bA = map(mouseY, 0, height, 0, height / 8);
beginShape();
for (var i = 0; i < density; i++) {
var t = map(i, 0, density, 0 ,PI * 2);
xA = (aA - bA) * cos(t) - bA * cos((aA - bA) * t);
yA = (aA - bA) * sin(t) - bA * sin((aA - bA) * t);
vertex(xA, yA);
}
endShape();
}
// draw devil's curve function
function devilDraw() {
var xD;
var yD;
var aD = map(mouseX, 0, width, 10, width/ 8);
var bD = map(mouseY, 0, height, 10, height / 10);
beginShape();
for (var j = 0; j < density; j++) {
var t = map(j, 0 , density, 0, PI * 2);
xD = cos(t) * sqrt((pow(aD, 2) * pow(sin(t), 2) - pow(bD, 2) * pow(cos(t), 2)) / (pow(sin(t), 2) - pow(cos(t), 2)));
yD = sin(t) * sqrt((pow(aD, 2) * pow(sin(t), 2) - pow(bD, 2) * pow(cos(t), 2)) / (pow(sin(t), 2) - pow(cos(t), 2)));
vertex(xD, yD);
}
endShape();
}
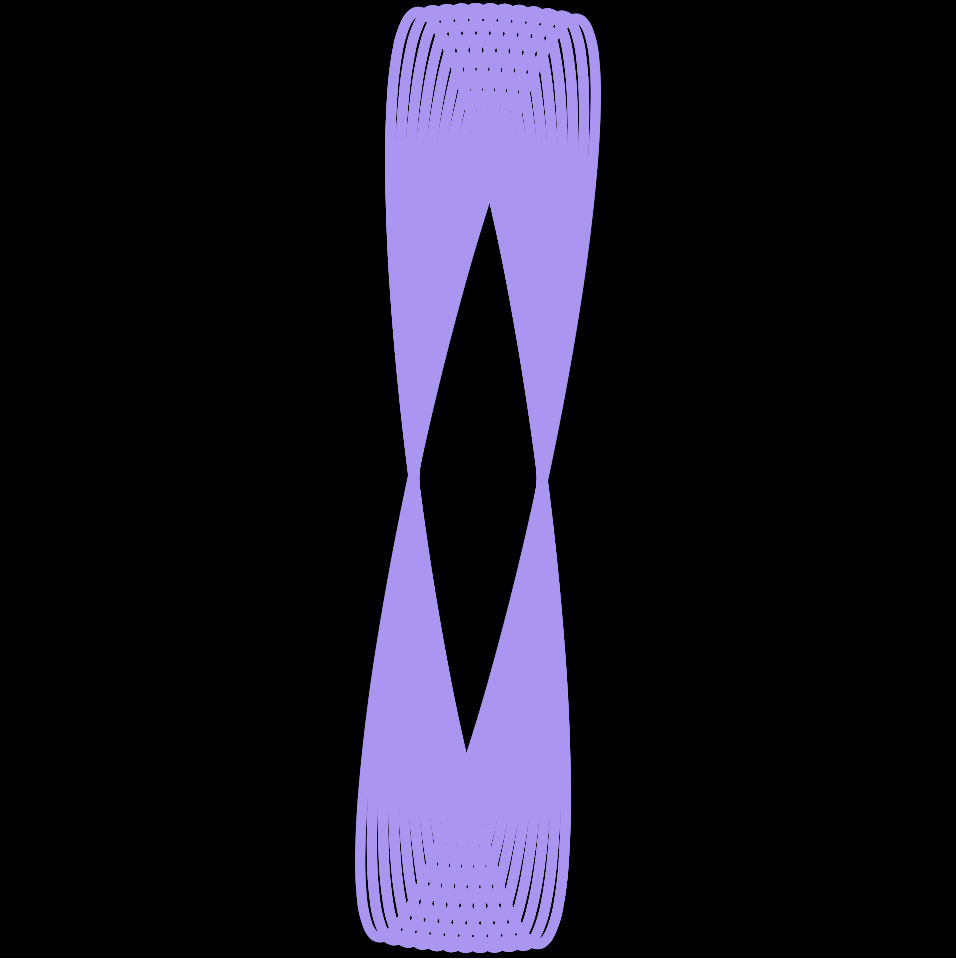
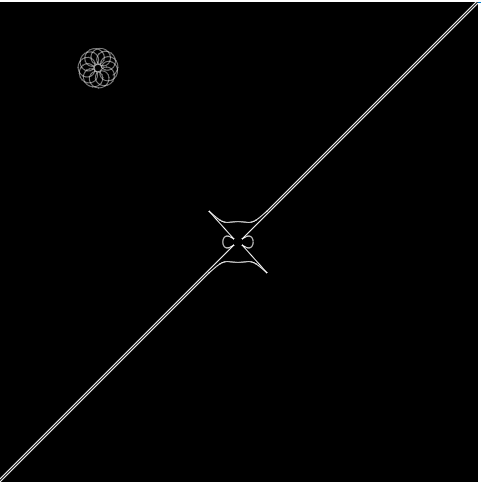
I started the project with browsing Mathworld Curves and found the curves that I am interested in. I used devil’s curve and hypocycloid. Devil’s curve remains at canvas center and its long diagonal edges change side when mouse moves, whereas hypocyloid itself flies with mouseX and mouseY. Hypocyloid is the smallest when it’s at upper left corner and largest at lower left. It is less complex and mouse moves to left of the canvas. The longest devil curve edges and the hypocyloid would only appear to be at the same edge when mouse is at upper right.