// Nawon Choi
// nawonc@andrew.cmu.edu
// Section C
// Project 07 Composition with Curves
// number of points
var np = 100;
function setup() {
createCanvas(400, 400);
}
function draw() {
background("black");
stroke("#f7fad2");
noFill();
strokeWeight(2);
// center the curves
push();
translate(200, 200);
drawHypocycloid(100);
// color of second curve depends on mouseX
fill(255, 200, mouseX);
drawHypocycloid(-200);
pop();
}
// http://mathworld.wolfram.com/HypocycloidEvolute.html
function drawHypocycloid(xy) {
var x;
var y;
var a = xy;
var b = mouseY;
beginShape();
for (var i = 0; i < np; i++) {
var t = map(i, 50, np, 50, TWO_PI);
var one = a / (a - 2 * b);
x = one * ((a - b) * cos(t) - b * cos(((a - b) / b) * t));
y = one * ((a - b) * sin(t) + b * sin(((a - b) / b) * t));
vertex(x, y);
}
endShape();
}
For this project, I chose to draw a curve called Hypocycloid Evolute. It was fun playing around by plugging in different variables at different points and seeing how it affects the shape. I eventually decided on having one of the variables, b, be dependent on the y-position of the mouse. I originally drew one curve, but decided to add another one to create depth. I drew another, larger curve and filled in the shape to create different flower-esque shapes as a result. I really enjoyed seeing how the changing colors and varying number of edges and angles created significantly different images with a single curve.
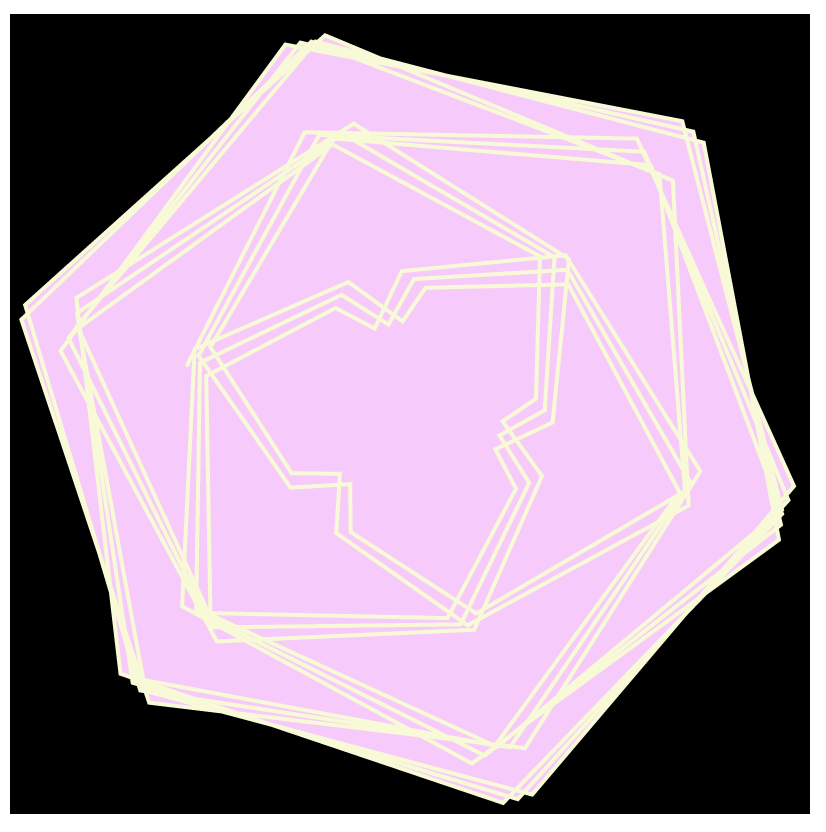

