//Crystal Xue
//15104-section B
//luyaox@andrew.cmu.edu
//Project_07
var nPoints = 100;
function setup() {
createCanvas(480, 480);
}
function draw() {
background(0);
translate(width/2, height/2);
drawEpitrochoid2();
drawHeart();
drawEpitrochoid();
}
function drawEpitrochoid2() {
//geometry rotation
rotate(map(mouseX, height, 0, TWO_PI, 0));
//color gradiant
var from = color(208, 16, 76);
var to = color(0, 137, 167);
var cH = lerpColor(from, to, map(mouseY, 0, width, 0 , 1));
stroke(255);
strokeWeight(0.2);
fill(cH);
//geometry parameters
var a = map(mouseX, 0 , width, 50, 200);
var b = a/30;
var h = map(mouseY,0, height, 100, 0);
//plotting geometry
beginShape();
for (var i = -1; i < 100; i ++) {
var t = map(i, 0, 100, 0, TWO_PI);
x = (a+b)*cos(t)-h*cos(((a+b)/b)*t);
y = (a+b)*sin(t)-h*sin(((a+b)/b)*t);
vertex(x, y)
}
endShape();
}
function drawHeart(){
//stop heart shapes from rotation
rotate(map(mouseX, height, 0, 0, TWO_PI));
push();
noStroke();
fill(255,50);
//size
var h = map(mouseY, 0, height, 0, 5);
//for how many layers of heart shapes
for (var z = 1; z < 8; z++) {
//plotting geometry
beginShape();
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
//heart equation
x = 16 * pow(sin(t),3);
y = -13 * cos(t)+ 5 * cos(2 * t) + 2 * cos(3 * t) + cos(4 * t);
vertex(x*z*h,y*z*h);
}
endShape(CLOSE);
}
}
function drawEpitrochoid() {
//geometry rotation
rotate(map(mouseY, 0, height, 0, TWO_PI));
//color gradiant
var from = color(178, 43, 206);
var to = color(247, 217, 76);
var cH = lerpColor(from, to, map(mouseX, 0, width, 0 , 1),100);
stroke(cH);
strokeWeight(1);
noFill();
//geometry parameters
var a = map(mouseX, 0 , width, 50, 200);
var b = a/20;
var h = map(mouseY,0, height, 50, 200);
//plotting the geometry
beginShape();
for (var i = -1; i < 100; i ++) {
var t = map(i, 0, 100, 0, TWO_PI);
x = (a+b)*cos(t)-h*cos(((a+b)/b)*t);
y = (a+b)*sin(t)-h*sin(((a+b)/b)*t);
vertex(x, y)
}
endShape();
}
This project is very intriguing to me because I got to explore the artistic value of math and geometry in a larger span.
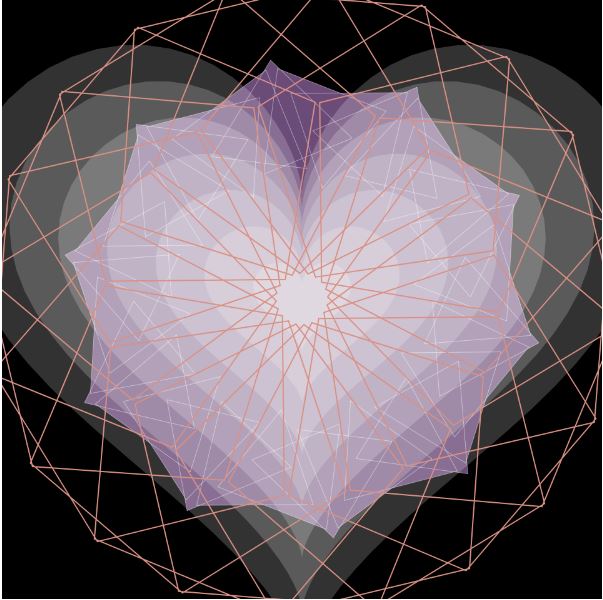
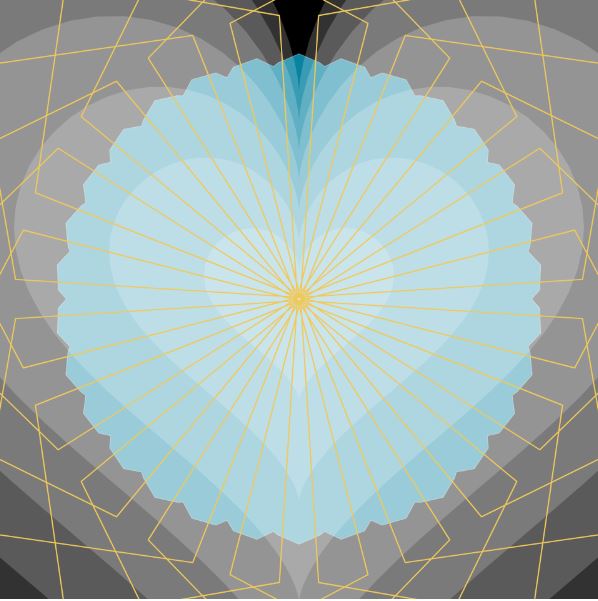