//Lanna Lang
//Section D
//lannal@andrew.cmu.edu
//Project 09 - Computational Portrait
var px = [];
var py = [];
//load the underlying image
function preload() {
var imgURL = "https://i.imgur.com/mSgPk6o.jpg";
underlyingImage = loadImage(imgURL);
}
function setup() {
createCanvas(480, 480);
background(0);
underlyingImage.loadPixels();
frameRate(10);
}
function draw() {
//for loop to draw the random lines that draw
//when the new frame is displayed
for (var i = 0; i < frameCount; i++) {
//the x and y array is
//drawn randomly
px[i] = random(width);
py[i] = random(height);
//get the color of the underlying image
//at the specific x, y location
var ix = constrain(floor(px[i]), 0, width-1);
var iy = constrain(floor(py[i]), 0, height-1);
var theColorAtLocationXY = underlyingImage.get(ix, iy);
//the person is drawn with smaller lines = more detail
//this if statement is for the head
if (px[i] >= 230 & px[i] <= 400 &&
py[i] >= 70 && py[i] <= 300) {
strokeWeight(1);
//the line drawn is the same color as the
//underlying image at its location
stroke(theColorAtLocationXY);
//the lines drawn is at a random angle
line(px[i] + random(5), py[i] + random(5),
px[i] + random(10), py[i] + random(10));
//this if statement is for the chest
} else if (px[i] >= 30 & px[i] <= width &&
py[i] >= 270 && py[i] <= 450) {
strokeWeight(1);
stroke(theColorAtLocationXY);
line(px[i] + random(5), py[i] + random(5),
px[i] + random(10), py[i] + random(10));
//this if statement is for the left hand & the sun
} else if (px[i] >= 50 && px[i] <= 200 &&
py[i] >= 90 && py[i] <= 220) {
strokeWeight(1);
stroke(theColorAtLocationXY);
line(px[i] + random(5), py[i] + random(5),
px[i] + random(10), py[i] + random(10));
//this if statement is for the left arm
} else if (px[i] >= 10 & px[i] <= 120 &&
py[i] >= 220 && py[i] <= 350) {
strokeWeight(1);
stroke(theColorAtLocationXY);
line(px[i] + random(5), py[i] + random(5),
px[i] + random(10), py[i] + random(10));
//the background is drawn with thicker lines = less detail
} else {
strokeWeight(4);
stroke(theColorAtLocationXY);
line(px[i] + random(10), py[i] + random(10),
px[i] + random(50), py[i] + random(50));
}
}
}
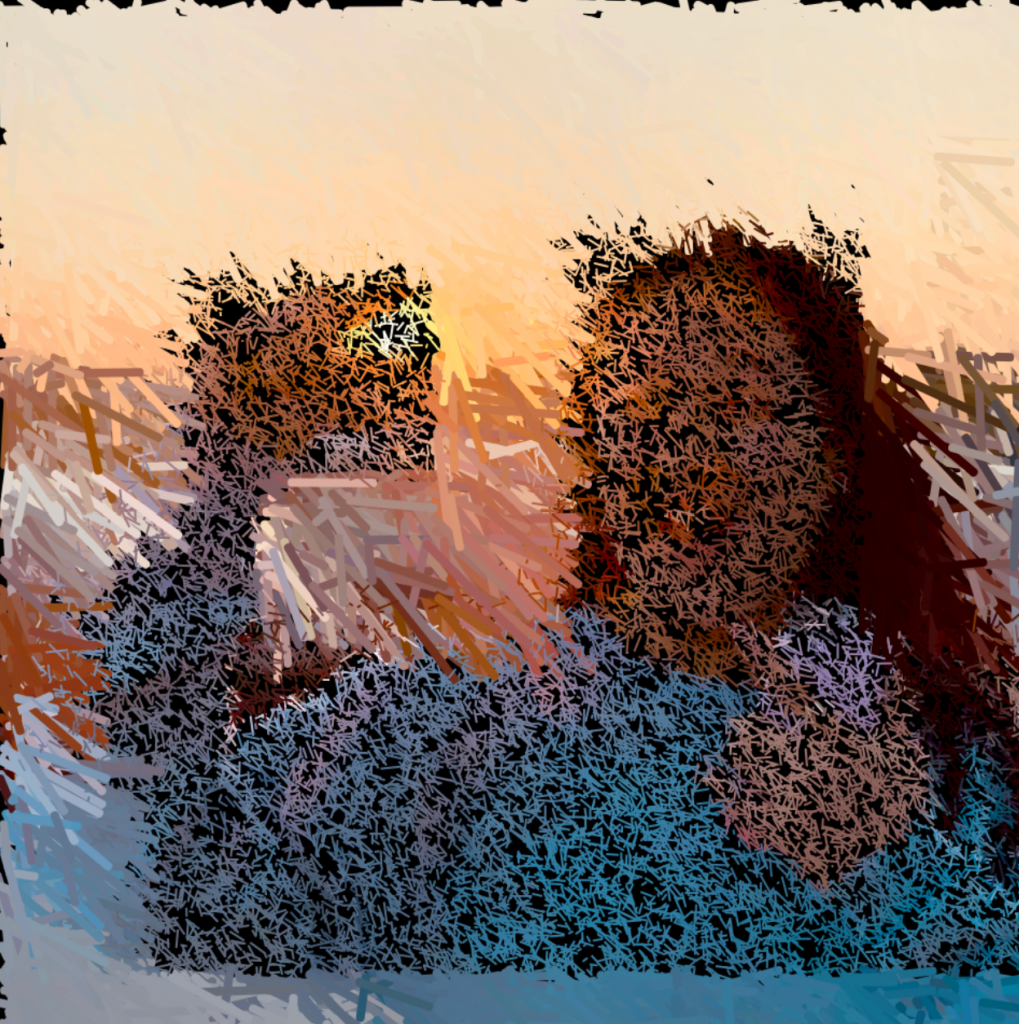
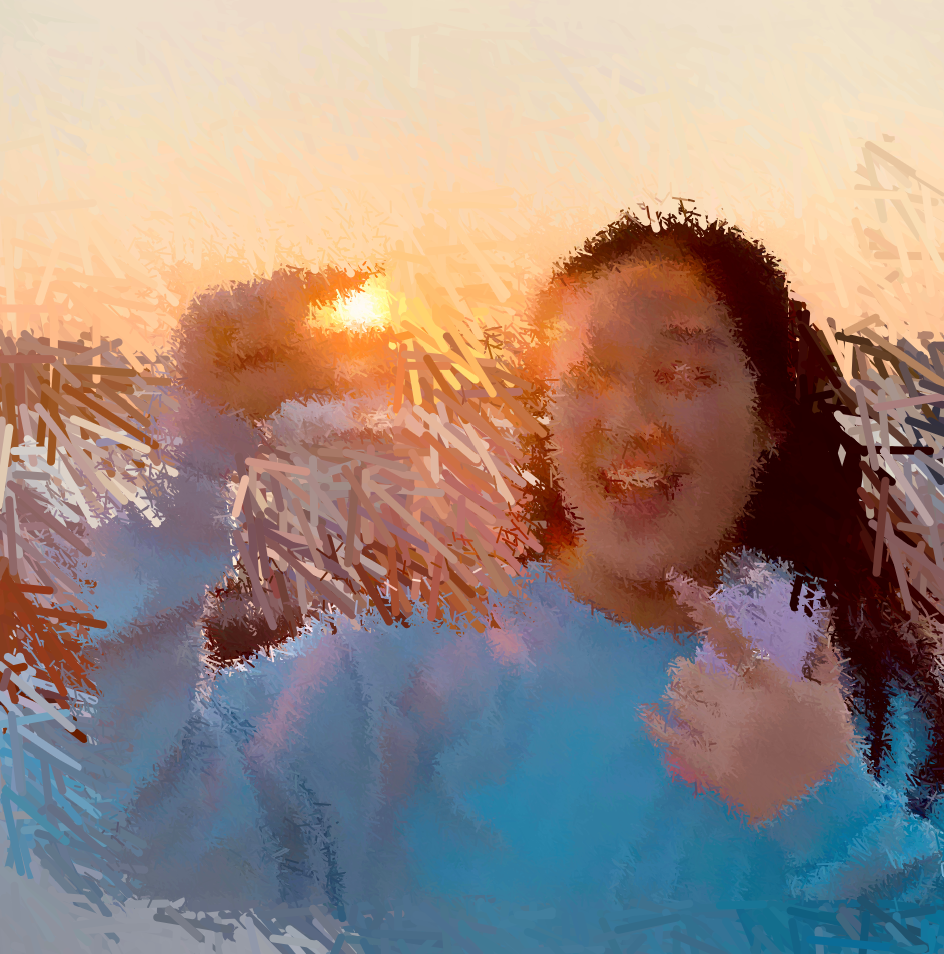
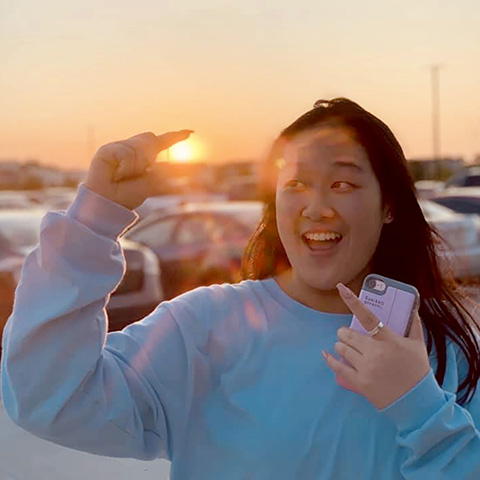
I struggled creating this code because I was very adamant about using random lines as my custom pixel and I couldn’t find out how to exactly execute it, but after using frameCount, I finally got it. I had a lot of fun writing my code, and this is one of my favorite photos I’ve taken so I am very satisfied with my final result.