//Sammie Kim
//Section D
//sammiek@andrew.cmu.edu
//Project 11
var xPos = 100;
var yPos = 50;
var size = 70;
var backgroundPic;
var clouds = [];
function preload() {
//background gradient photo
var backgroundURL = "https://i.imgur.com/EMQBV3U.jpg"
backgroundPic = loadImage(backgroundURL);
}
function setup() {
createCanvas(480, 480);
frameRate(10);
//initial clouds first appears anywhere across the canvas
for (var i = 0; i < 1; i++) {
var startX = random(0, width/3);
clouds[i] = createClouds(startX);
}
}
function draw() {
background(0);
image(backgroundPic, 0, 0);
noStroke();
//call the functions below
sun();
mountain();
darkOcean();
ocean();
//show clouds
addNewClouds();
updateClouds();
removeClouds();
}
function sun() {
fill('#f29849');
circle(xPos, yPos, size);
xPos = xPos + 0.5;
if (xPos > width + size/2){
xPos = 0;
}
}
function updateClouds() {
//constantly calling the clouds
for (var x = 0; x < clouds.length; x++){
clouds[x].move();
clouds[x].draw();
}
}
function removeClouds() {
//create an array for keeping clouds on screen
//if x location is greater than 0, then push x values into the cloud array
var cloudsOnScreen = [];
for (var i = 0; i < clouds.length; i++) {
if (clouds[i].x > 0) {
cloudsOnScreen.push(clouds[i]);
}
}
clouds = cloudsOnScreen;
}
function addNewClouds() {
var probability = 0.004;
//a new cloud is drawn at the width when it is greater than a certain probability
if (random(0, 1) < probability) {
clouds.push(createClouds(width));
}
}
function moveClouds() {
//making clouds move to the left
this.x -= this.speed;
}
function makeClouds() {
//drawing the cloud with ellipses overlapping one another
noStroke();
fill('#7297a6');
ellipse(this.x - 10, this.y, this.width, this.height);
ellipse(this.x + 30, this.y, this.width, this.height);
ellipse(this.x, this.y + 5, this.width, this.height);
ellipse(this.x - 30, this.y + 5, this.width, this.height);
}
function createClouds(cloudX) {
//creating object for cloud's characteristics
var cloud = {x: cloudX,
y: random(25, 75),
width: 50,
height: 50,
speed: 0.7,
move: moveClouds,
draw: makeClouds}
return cloud;
}
function ocean() {
var oceanSpeed = 0.0003; //speed of terrain
var oceanDetail = 0.001; //smoothness
fill('#50abbf');
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * oceanDetail) + (millis() * oceanSpeed);
var y = map(noise(t), 0, 1, height/2, height);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
function mountain() {
var oceanSpeed = 0.0004; //speed of terrain
var oceanDetail = 0.007; //smoothness
fill('#6C671F');
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * oceanDetail) + (millis() * oceanSpeed);
var y = map(noise(t), 0, 1, 0, height);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
function darkOcean() {
var oceanSpeed = 0.0004; //speed of terrain
var oceanDetail = 0.001; //smoothness
fill('#254f6a');
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * oceanDetail) + (millis() * oceanSpeed);
var y = map(noise(t), 0, 1, height/3, height);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
This project was challenging as I had to keep in mind about the different objects that are shown on canvas and making them move. Imagining a space with ocean and mountain, I wanted to bring out a happy, peaceful mood through the overall warm colors. Overlapping the ocean terrains, I attempted to make an image of waves flowing past. The biggest challenge for me was creating the clouds to appear in the sky using objects. It was difficult having to think about multiple factors and constraints all at once, so I did it in a step by step manner—creating the cloud shape first with function, then calling it inside the object, and finally using other functions to add more properties.
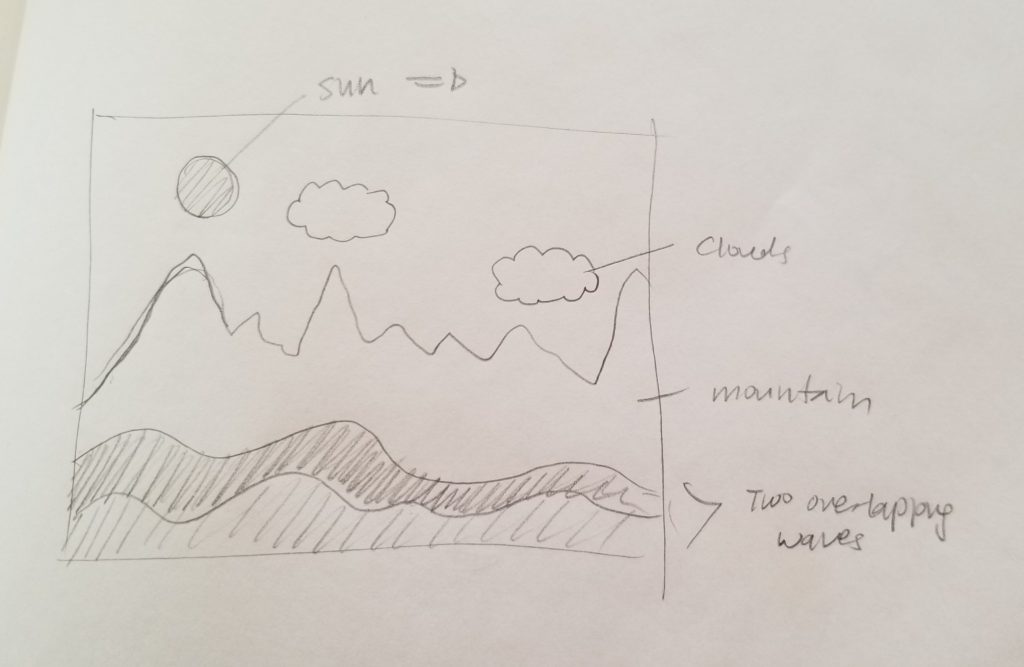