//Minjae Jeong
//Section B
//minjaej@andrew.cmu.edu
//Project-11
var trees = [];
function setup() {
createCanvas(480, 400);
// collection of trees
for (var i = 0; i < 10; i++){
var rx = random(width);
trees[i] = makeTrees(rx);
}
frameRate(100);
}
function draw() {
background('lightblue');
drawRoad();
updateTrees();
removeTrees();
addNewTrees();
drawRedcar(180, 230);
drawYellowcar(260, 330);
drawSun();
}
function drawSun() {
fill(255, 102, 0);
ellipse(440,20, 130);
}
function drawRoad() {
//grey road
fill(163);
rect(0, 220, 480, 180);
//white line
fill('white');
rect(0, 290, 480, 5);
}
function drawRedcar(x , y) {
strokeWeight(0);
//body
fill('red');
rect(x, y, 100, 20);
rect(x + 20, y - 20, 50, 20);
//tires
fill('black');
ellipse(x + 20, y + 20, 21, 21);
ellipse(x + 80, y + 20, 21, 21);
//wheels
fill('white');
ellipse(x + 20, y + 20, 15, 15);
ellipse(x + 80, y + 20, 15, 15);
fill('red');
ellipse(x + 20, y + 20, 3, 3);
ellipse(x + 80, y + 20, 3, 3);
//windows
fill(102, 204, 255);
rect(x + 28, y - 17, 35, 15);
}
function drawYellowcar(x , y) {
strokeWeight(0);
//body
fill('yellow');
rect(x, y, 100, 20);
rect(x + 20, y - 20, 50, 20);
//tires
fill('black');
ellipse(x + 20, y + 20, 21, 21);
ellipse(x + 80, y + 20, 21, 21);
//wheels
fill('white');
ellipse(x + 20, y + 20, 15, 15);
ellipse(x + 80, y + 20, 15, 15);
fill('blue');
ellipse(x + 20, y + 20, 3, 3);
ellipse(x + 80, y + 20, 3, 3);
//windows
fill(102, 204, 255);
rect(x + 28, y - 17, 35, 15);
}
function updateTrees(){
for (var i = 0; i < trees.length; i++){
trees[i].move();
trees[i].display();
}
}
function removeTrees(){
var treesToKeep = [];
for (var i = 0; i < trees.length; i++){
if (trees[i].x + trees[i].breadth > 0) {
treesToKeep.push(trees[i]);
}
}
trees = treesToKeep; // remember the surviving buildings
}
function addNewTrees() {
//probability of new trees
var newTreeLikelihood = 0.01;
if (random(0,1) < newTreeLikelihood) {
trees.push(makeTrees(width));
}
}
// method to update position of tree every frame
function treeMove() {
this.x += this.speed;
}
// draw the trees
function treeDisplay() {
fill(119, 83, 0);
noStroke();
push();
translate(this.x, height - 230);
noStroke();
rect(0, 0, 15, 50);
pop();
fill(0, 153, 0);
noStroke();
push();
translate(this.x, height - 230);
noStroke();
ellipse(0, 20, 40);
ellipse(10, 3, 40);
ellipse(20, 20, 40);
pop();
}
function makeTrees(birthLocationX) {
var tree = {x: birthLocationX,
y: 200,
breadth: 50,
speed: -1.0,
move: treeMove,
display: treeDisplay}
return tree;
}
For this project, I wanted to create a project that displays cars and the surroundings, which are sky, trees, road, and a sun. It was more challenging than my expectation but will be fun and useful once I absorb it.
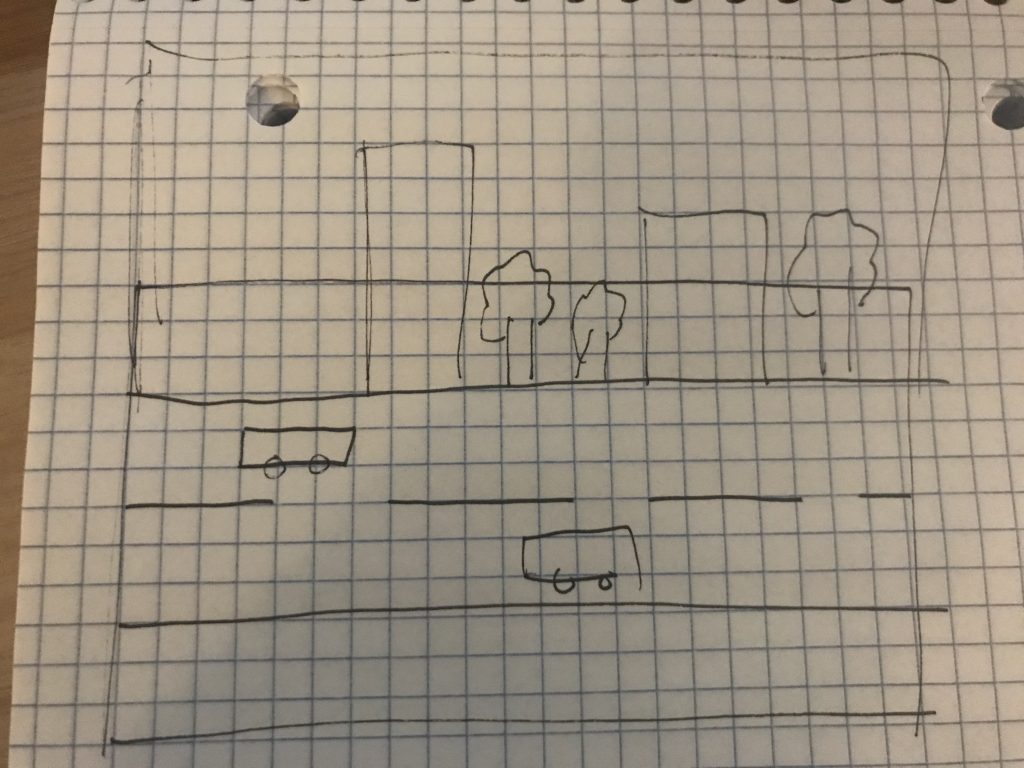