/* Youie Cho
Section E
minyounc@andrew.cmu.edu
Project-11-Landscape*/
var terrainSpeed = 0.0001;
var terrainDetail = 0.005;
var houses = [];
var tY = 130;
function setup() {
createCanvas(400, 300);
frameRate(10);
// initial collection of houses
for (var i = 0; i < 10; i++){
var rx = random(width);
houses[i] = makehouse(rx);
}
}
function draw() {
background(219, 110, 163);
snowyTerrain();
snow();
house();
// Happy November text moving upwards
fill(196, 71, 132);
textSize(12);
textFont('Helvetica');
text('Happy November', 150, tY);
tY -= 0.05;
// moon
fill(255, 238, 194);
ellipse(330, 40, 40, 40);
}
function snow() {
for (var i = 0; i < 50; i++) {
ellipse(random(0, width), random(0, height), 1, 1);
}
}
function snowyTerrain() {
noStroke();
fill(252, 230, 240);
beginShape();
for (var x = 0; x < width + 1; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0,1, height * 0.5, height * 0.8);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
function house() {
push();
translate(0.1 * width, 0.1 * height);
scale(0.8);
stroke(0);
noFill();
updateAndDisplayhouses();
removehousesThatHaveSlippedOutOfView();
addNewhousesWithSomeRandomProbability();
pop();
}
// houses' positions are updated and displayed
function updateAndDisplayhouses(){
for (var i = 0; i < houses.length; i++) {
houses[i].move();
houses[i].display();
}
}
// houses outside of the canvas are removed
function removehousesThatHaveSlippedOutOfView() {
var housesToKeep = [];
for (var i = 0; i < houses.length; i++){
if (houses[i].x + houses[i].breadth + 70 > 0) {
housesToKeep.push(houses[i]);
}
}
houses = housesToKeep;
}
// new house is added at the end with small probability
function addNewhousesWithSomeRandomProbability() {
var newhouseLikelihood = 0.01;
if (random(0, .5) < newhouseLikelihood) {
houses.push(makehouse(width + 70));
}
}
// method to update position of house every frame
function houseMove() {
this.x += this.speed;
}
// draw the houses
function houseDisplay() {
var floorHeight = 15;
var bHeight = this.nFloors * floorHeight;
// house block
fill(145, 55, 26);
noStroke();
push();
translate(this.x, height - 40);
rect(0, -bHeight + 20, this.breadth, bHeight);
// roof
fill(61, 27, 18);
triangle(-10, -bHeight + 20, this.breadth/2, -bHeight * 2, this.breadth + 10, -bHeight + 20)
// snow on the roof
stroke(255);
strokeWeight(6);
strokeJoin(ROUND);
fill(255);
triangle(0, -bHeight, this.breadth/2, -bHeight * 2, this.breadth, -bHeight)
//window that flickers
strokeWeight(0.5);
stroke(0);
fill("yellow")
noStroke();
var wL = this.nFloors * 6 // variable to determine location
var wW = random(25, 27); //variable to determine width and height
rect(wL, -bHeight + wL * 2, wW, wW - 10);
// windowsill
stroke(0);
line(wL + wW / 2, -bHeight + wL * 2, wL + wW / 2, -bHeight + wL * 2 + wW - 10);
line(wL, -bHeight + wL * 2 + wW / 2 - 5, wL + wW, -bHeight + wL * 2 + wW / 2 - 5);
pop();
}
function makehouse(birthLocationX) {
var hs = {x: birthLocationX,
breadth: 70,
speed: -4,
nFloors: round(random(2,5)),
move: houseMove,
display: houseDisplay}
return hs;
}
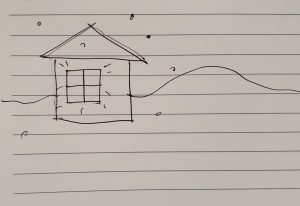
I wanted to create a scene that represented the Christmas season, which I like a lot. I made the terrains become snowy hills, and added colors that made the scenery seem pleasant. It was fun to play around with different factors of my drawings, especially to control different things in a way they depend on other elements. For instance, I made my window and windowsills randomly move together to create an effect of the yellow light flickering from each house. It was also fun to add snow on the roofs using rounded joining corners. If I were to continue to work on this, I would want to do something more interesting with the text movement.