/* Mari Kubota
49-104 Section 1
mkubota@andrew.cmu.edu
Project 11
*/
var lamps = [];
var stars = [];
var buildings = [];
function setup() {
createCanvas(480, 280);
// create an initial collection of objects
for (var i = 0; i < 10; i++){
var rx = random(width);
lamps[i] = makeLamp(rx);
stars[i] = makeStar(rx);
buildings[i] = makeBuilding(rx);
}
frameRate(10);
}
function draw() {
//creates gradient sky
c1 = color(0, 28, 84);
c2 = color(222, 241, 255);
setGradient(c1, c2);
//creates roads
noStroke();
fill(100);
rect(0, height*.75, width, height/4);
fill(200);
stroke(50);
rect(0,height*.9, width, height/9);
//calls functions with objects
updateAndDisplayStars();
removeStarsThatHaveSlippedOutOfView();
addNewStarsWithSomeRandomProbability();
updateAndDisplayBuildings();
removeBuildingsThatHaveSlippedOutOfView();
addNewBuildingsWithSomeRandomProbability();
updateAndDisplayLamps();
removeLampsThatHaveSlippedOutOfView();
addNewLampsWithSomeRandomProbability();
}
function updateAndDisplayLamps(){
// Update the building's positions, and display them.
for (var i = 0; i < lamps.length; i++){
lamps[i].move();
lamps[i].display();
}
}
function updateAndDisplayStars(){
// Update the building's positions, and display them.
for (var i = 0; i < stars.length; i++){
stars[i].move();
stars[i].display();
}
}
function updateAndDisplayBuildings(){
// Update the building's positions, and display them.
for (var i = 0; i < buildings.length; i++){
buildings[i].move();
buildings[i].display();
}
}
//////////////////////////////////////////////////////
function removeLampsThatHaveSlippedOutOfView(){
// takes away lamps once they leave the frame
var lampsToKeep = [];
for (var i = 0; i < lamps.length; i++){
if (lamps[i].x + lamps[i].breadth > 0) {
lampsToKeep.push(lamps[i]);
}
}
lamps = lampsToKeep; // remember the surviving buildings
}
////////////////////////////////////////////////////////
function removeStarsThatHaveSlippedOutOfView(){
//removes stars from array stars and puts them in new array
//once they've moved off the edge of frame
var starsToKeep = [];
for (var i = 0; i < stars.length; i++){
if (stars[i].x + stars[i].breadth > 0) {
starsToKeep.push(stars[i]);
}
}
stars = starsToKeep; // remember the surviving stars
}
function removeBuildingsThatHaveSlippedOutOfView(){
var buildingsToKeep = [];
for (var i = 0; i < buildings.length; i++){
if (buildings[i].x + buildings[i].breadth > 0) {
buildingsToKeep.push(buildings[i]);
}
}
buildings = buildingsToKeep; // remember the surviving buildings
}
////////////////////////////////////////////////////////////////////////
function addNewLampsWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newLampLikelihood = 0.03;
if (random(0,1) < newLampLikelihood) {
lamps.push(makeLamp(width));
}
}
function addNewStarsWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newStarLikelihood = 0.02;
if (random(0,1) < newStarLikelihood) {
stars.push(makeStar(width));
}
}
function addNewBuildingsWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newBuildingLikelihood = 0.007;
if (random(0,1) < newBuildingLikelihood) {
buildings.push(makeBuilding(width));
}
}
///////////////////////////////////////////////////////////////////////////
// method to update position of building every frame
function lampMove() {
this.x += this.speed;
}
function starMove() {
this.x += this.speed;
}
function buildingMove() {
this.x += this.speed;
}
//////////////////////////////////////////////////////////////////////////////
// draws lamps
function lampDisplay() {
fill(255,255,0,45);
noStroke();
ellipse(this.x, height -25 - this.h,this.r,this.r); //halo of light from lamp
fill(102,166,218);
rect(this.x-1.5, height - 25-this.h, this.breadth, this.h); //lamp post
fill(255);
ellipse(this.x, height -25 - this.h, this.r2, this.r2); //lightbulb
stroke(255);
strokeWeight(0.5);
noFill();
quad(this.x - 1.5, height - 20 - this.h,
this.x - 5, height - 35 - this.h,
this.x + 5, height - 35 - this.h,
this.x + this.breadth - 1.5, height - 20 - this.h); //draws the lamp box
}
function starDisplay(){
//halo of light around star
noStroke();
fill(255, 255, 0, 30);
ellipse(this.x + this.breadth/2, this.h, this.breadth * 5, this.breadth * 5);
stroke(255,255,0);
strokeWeight(0.5);
noFill();
//draws diamonds that make up star
quad(this.x, this.h,
this.x + this.breadth / 2,this.h - this.tall / 2,
this.x + this.breadth, this.h,
this.x + this.breadth / 2,this.h + this.tall / 2);
}
function buildingDisplay() {
var floorHeight = 20;
var bHeight = height;
noStroke();
fill(0);
push();
translate(this.x, height - 40);
rect(0, -bHeight, this.breadth, bHeight);
for (var i = 0; i < this.nFloors; i+=2) {
fill("yellow");
rect(5, -15 - (i * floorHeight), this.breadth - 10, 15);
}
pop();
}
//////////////////////////////////////////////////////////////////////////
function makeLamp(posX) {
var lamp = {x: posX,
breadth: 3,
speed: -2.5,
h: random(40,60),
r: random(20,35),
r2: 4,
move: lampMove,
display: lampDisplay}
return lamp;
}
function makeStar(posX) {
var star = {x: posX,
breadth: 3,
speed: -0.5,
tall: random(5,10),
h: random(20, 100),
move: starMove,
display: starDisplay}
return star;
}
function makeBuilding(birthLocationX) {
var bldg = {x: birthLocationX,
breadth: 60,
speed: -1.0,
nFloors: round(random(10,20)),
move: buildingMove,
display: buildingDisplay}
return bldg;
}
//////////////////////////////////////////////////////////////////////////////
//gradient sky function
function setGradient(c1, c2) {
noFill();
for (var y = 0; y < height; y++) {
var inter = map(y, 0, height, 0, 1);
var c = lerpColor(c1, c2, inter);
stroke(c);
line(0, y, width, y);
}
}
For this project, I created a landscape of a city during the night time. The three objects in the landscape are the buildings, lamps, and stars. All of the objects appear randomly in random quantities. The only consistency is the height at which the buildings and lamps appear. The lamps’s colors are randomized and made transparent in order to create a halo effect. The objects all move across the canvas at different speeds in order to create a sense of depth. The stars move slowest, followed by the buildings, and the lamp posts move the fastest.
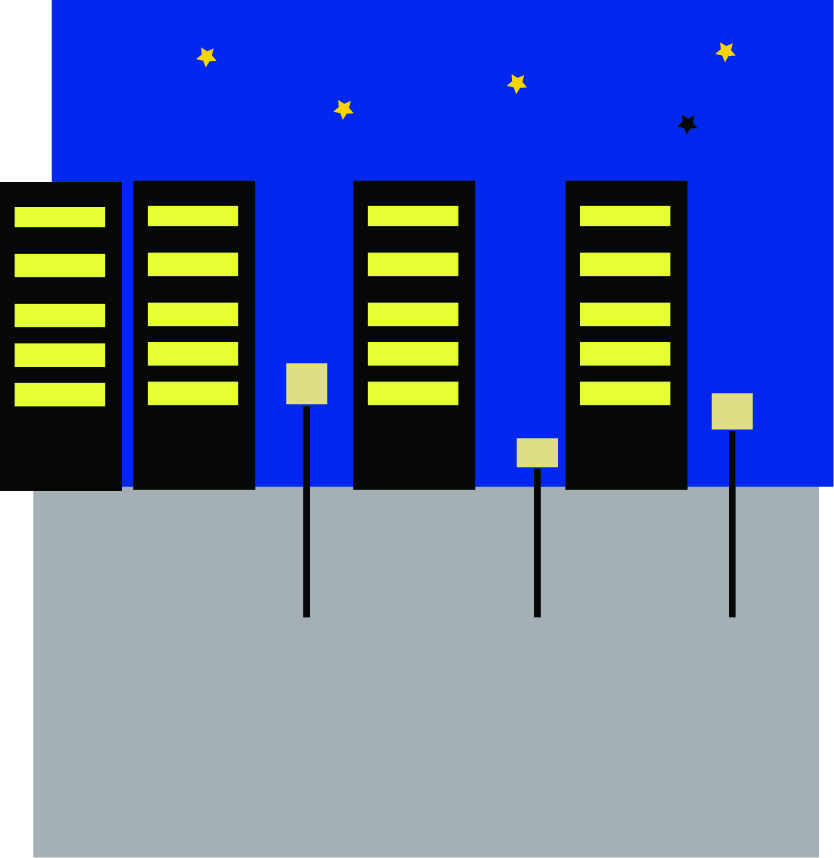