let flowers = [];
let grass = [];
let clouds = [];
function setup() {
createCanvas(600,600);
//inital placement for flowers
for (let i = 0; i < 50; i++) {
let firstX = random(width);
let firstY = random(height);
flowers[i] = makingFlower(firstX,firstY)
}
// inital placement for grass
for (let gx = 0; gx < 400; gx++) {
let grassX = random(width);
let grassY = random(height);
grass[gx] = makingGrass(grassX,grassY);
}
// initial placement for clouds
for (let j = 0; j < 7; j++) {
let cloudx = random(width);
let cloudy = random(height);
clouds[j] = makingCloud(cloudx, cloudy);
}
}
function draw() {
background(105,130,80);
//drawing the grass
updateAndDisplayGrass();
addingGrass();
removingGrass();
//drawing the flowers
updateAndDisplayflowers();
addingFlowers();
removingFlowers();
// drawing the clouds
updateAndDisplayClouds();
addingClouds();
removingClouds();
}
////////////////////////////////////flowers//////////////////////////
///removing flowers from the array once they go off screen
function removingFlowers() {
var keeping = [];
for (var i = 0; i < flowers.length; i++) {
if (flowers[i].x + flowers[i].r > 0) {
keeping.push(flowers[i]);
}
}
flowers = keeping; //keeping certain flowers in the flowers array
}
//update all the flowers in the array
function updateAndDisplayflowers(){
for (var i = 0; i < flowers.length; i++){
flowers[i].move();
flowers[i].display();
}
}
//adding new flowers to the array according to a certain liklihood
function addingFlowers() {
var newflowerliklihood = 0.05;
var initialX = random(width);
var initialY = 0;
if (random(0,1) < newflowerliklihood) {
flowers.push(makingFlower(initialX,initialY));
}
}
//the flower object
function makingFlower(firstX,firstY) {
var flr = {x: firstX,
y: firstY,
r: round(random(10,40)),
move: moveflowers,
display: showflowers}
return flr;
}
//specifying the drawing of the flower
function showflowers() {
noStroke();
fill(230, 80, 50); //red petals
ellipse(this.x+3, this.y, 3, 4);
ellipse(this.x-3, this.y, 3, 4);
ellipse(this.x, this.y-3, 3, 4);
ellipse(this.x, this.y+3, 3, 4);
fill(230, 130, 50); // orange center
ellipse(this.x, this.y, 5, 5);
fill(90, 40, 30); // red inner center
ellipse(this.x, this.y, 1, 1);
}
//speciftying the movement of the flower
function moveflowers() {
this.y = this.y + 1
}
/////////////////////////////////// grass////////////////////////////////
function removingGrass() {
var keepingGrass = [];
for (var i = 0; i < grass.length; i++) {
if (grass[i].x > -10) {
keepingGrass.push(grass[i]);
}
}
grass = keepingGrass;
}
function updateAndDisplayGrass(){
for (var i = 0; i < grass.length; i++){
grass[i].move();
grass[i].display();
}
}
function addingGrass() {
var newgrassliklihood = 0.5;
var initialX = random(width);
var initialY = 0;
if (random(0,1) < newgrassliklihood) {
grass.push(makingGrass(initialX,initialY));
}
}
function makingGrass(grassX,grassY) {
var gss = {x: grassX,
y: grassY,
move: moveGrass,
display: showGrass}
return gss;
}
function showGrass() {
strokeWeight(random(1,2));
stroke(130,160,140);
line(this.x, this.y, this.x-2, this.y+2);
}
function moveGrass() {
this.y = this.y + 1
}
/////////////////////clouds///////////////////////////////////////////////
function removingClouds() {
var keepingClouds = [];
for (var i = 0; i < clouds.length; i++) {
if (clouds[i].x > -10) {
keepingClouds.push(clouds[i]);
}
}
clouds = keepingClouds;
}
function updateAndDisplayClouds(){
for (var i = 0; i < clouds.length; i++){
clouds[i].move();
clouds[i].display();
}
}
function addingClouds() {
var newcloudliklihood = 0.02;
var initialX = random(width);
var initialY = 0;
if (random(0,1) < newcloudliklihood) {
clouds.push(makingCloud(initialX,initialY));
}
}
function makingCloud(cloudX,cloudY) {
var cld = {x: cloudX,
y: cloudY,
move: moveCloud,
display: showCloud}
return cld;
}
function showCloud() {
noStroke();
fill(150,180,190,20); // light blue
beginShape();
vertex(this.x , this.y);
quadraticVertex(this.x - 236, this.y - 351 , this.x + 7 , this.y - 357);
bezierVertex(this.x -17, this.y -215, this.x + 132, this.y + 180, this.x, this.y);
endShape(CLOSE)
}
function moveCloud() {
this.y = this.y + 3
}
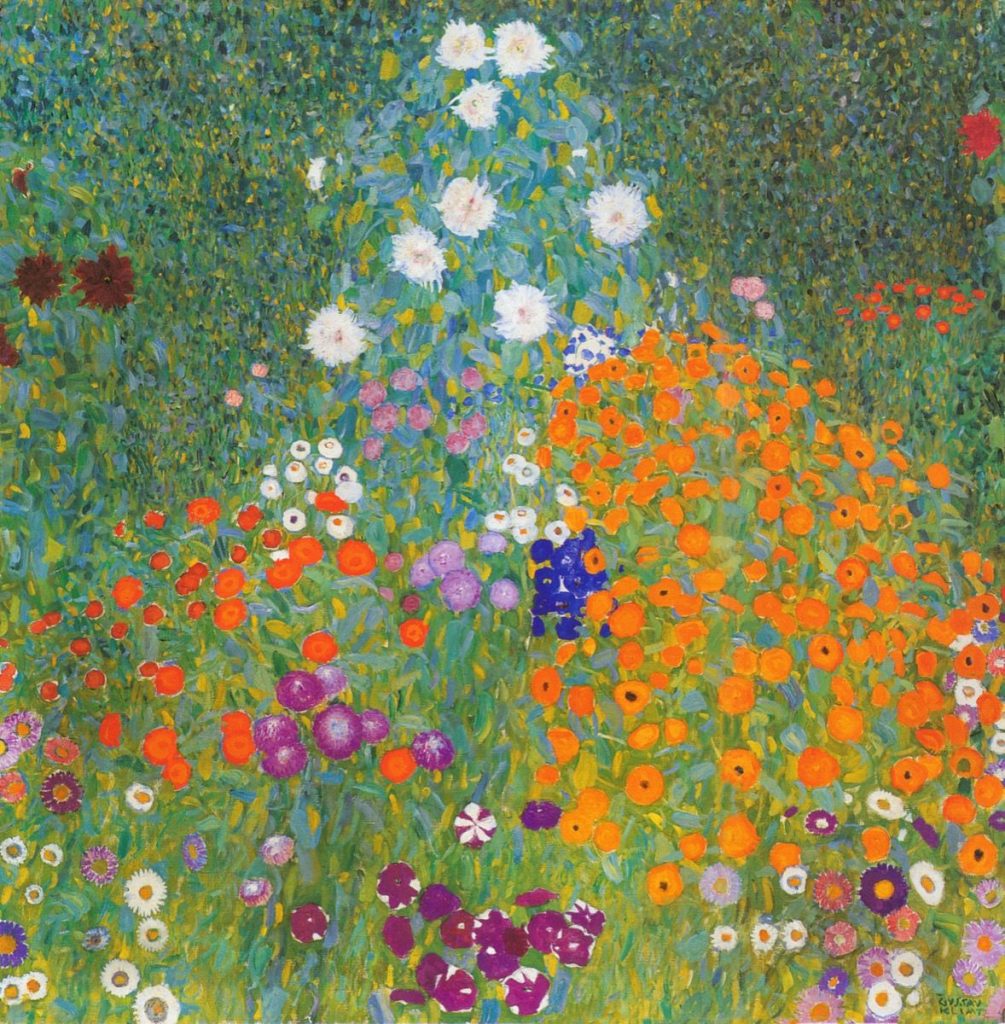
For this project, I was really inspired by the prompt of looking outside of a window. I love flowers and the peacefulness of watching clouds pass. I also love the colors in Gustav Klimpt’s landscapes, so I really wanted to incorporate this into my project, as well as get some practice drawing curves.