//Sarah Choi
//sychoi
//Section D
//Project-11
var buildings = [];
var birds = [];
var terrainSpeed = 0.0001;
var terrainDetail = 0.005;
function setup() {
createCanvas(480, 480);
frameRate(10);
for (var i = 0; i < 10; i++){
var rx = random(width);
var ry = random(height);
buildings[i] = makeBuilding(rx);
birds[i] = birdsFlying(rx, ry);
}
}
function draw() {
background(200);
displayHorizon();
drawMountain();
updateAndDisplayBuildings();
removeBuildingsThatHaveSlippedOutOfView();
addNewBuildingsWithSomeRandomProbability();
birdsFlying();
birdMovement();
displayBird();
updateBird();
addBird();
removeBird();
}
function drawMountain() {
fill(150, 60, 50);
noStroke();
beginShape();
for (x = 0; x < width; x++) {
var m = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 1, 0, height);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
function updateAndDisplayBuildings(){
// Update the building's positions, and display them.
for (var i = 0; i < buildings.length; i++){
buildings[i].move();
buildings[i].display();
}
}
function removeBuildingsThatHaveSlippedOutOfView(){
var buildingsToKeep = [];
for (var i = 0; i < buildings.length; i++){
if (buildings[i].x + buildings[i].breadth > 0) {
buildingsToKeep.push(buildings[i]);
}
}
buildings = buildingsToKeep; // remember the surviving buildings
}
function addNewBuildingsWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newBuildingLikelihood = 0.007;
if (random(0,1) < newBuildingLikelihood) {
buildings.push(makeBuilding(width));
}
}
// method to update position of building every frame
function buildingMove() {
this.x += this.speed;
}
// draw the building and some windows
function buildingDisplay() {
var floorHeight = 20;
var bHeight = this.nFloors * floorHeight;
fill(255);
stroke(0);
push();
translate(this.x, height - 40);
rect(0, -bHeight, this.breadth, bHeight);
stroke(200);
for (var i = 0; i < this.nFloors; i++) {
rect(5, -15 - (i * floorHeight), this.breadth - 10, 10);
}
pop();
}
function makeBuilding(birthLocationX) {
var bldg = {x: birthLocationX,
breadth: 50,
speed: -1.0,
nFloors: round(random(2,8)),
move: buildingMove,
display: buildingDisplay}
return bldg;
}
function displayHorizon(){
stroke(0);
line (0, height - 50, width, height - 50);
}
function birdsFlying(birdX, birdY) {
var bird = {x: birdX,
y: birdY,
speed: random(5, 20),
size: random(5, 20),
color: random(0, 100),
move: birdMovement,
display: displayBird}
return bird;
}
function birdMovement() {
this.x += this.speed;
this.y += this.speed / 5;
}
function displayBird() {
strokeWeight(1);
stroke(this.color);
noFill();
push();
translate(this.x, this.y);
arc(0, 0, this.size, this.size, PI + QUARTER_PI, 0);
arc(this.size, 0, this.size, this.size, PI, - QUARTER_PI);
pop();
}
function updateBird() {
for (i = 0; i < birds.length; i++) {
birds[i].move();
birds[i].display();
}
}
function addBird() {
var newBird = random(1);
if (newBird < 0.2) {
var birdX = 0;
var birdY = random(height);
birds.push(displayBird(birdX, birdY));
}
}
function removeBird() {
var stayBird = [];
for (i = 0; i < birds.length; i++) {
if (birds[i].x + birds[i].size > 0) {
stayBird.push(birds[i]);
}
}
birds = stayBird;
}
I wanted to show birds with the buildings in the background. Growing up in a city, there were always tall skyscrapers and birds flying everywhere especially next to really big parks. I love walking around in the city where you can breathe in the fresh air and feel so free in an area where you grew up.
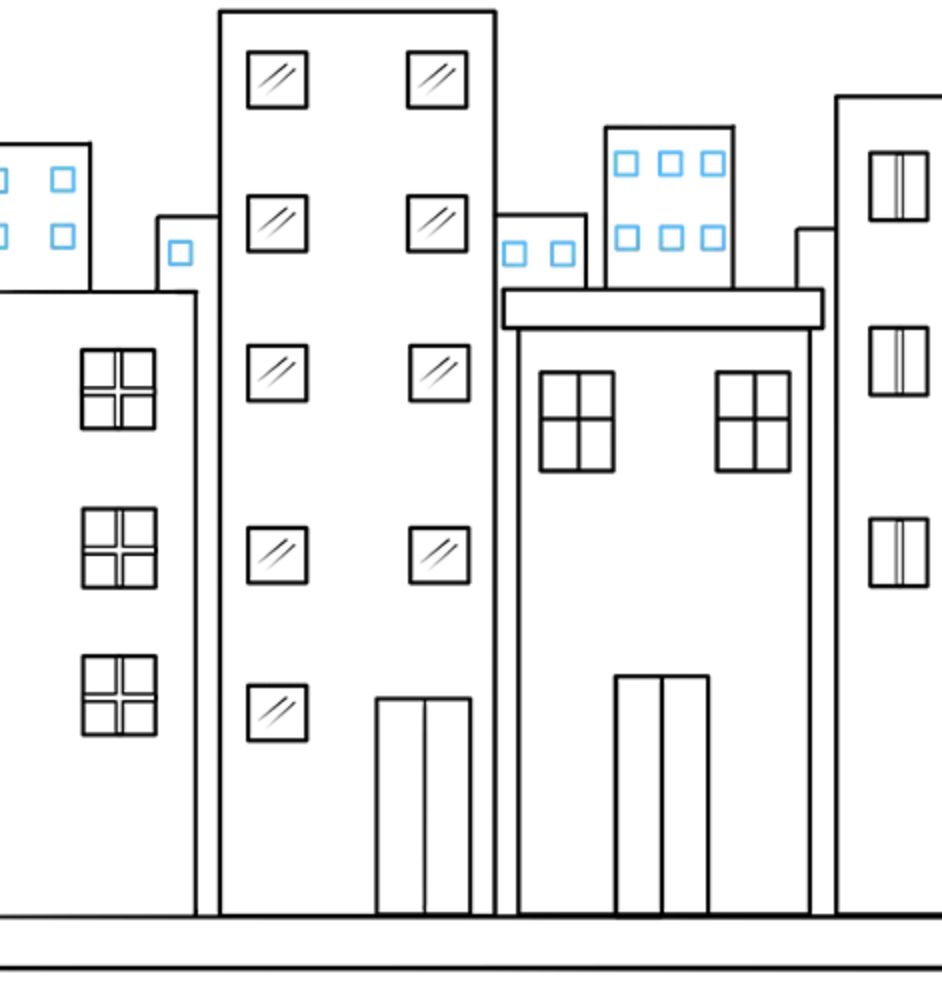
I was unable to figure out how to make both the birds and the buildings to appear in my code. Although in the end, I was still unsure how to make my code work, I hope the code is enough to show my efforts.