For this project, I tried to create variations of sizes, colors, and positions utilizing the original self portrait I created for our last deliverable. Different sizes, colors, shapes, and positions make changes to the mood of the portrait that was originally adorable.
// Stefanie Suk
// 15-104 Section D
var bgColorR = 208;
var bgColorG = 233;
var tiecolorR = 0;
var tiecolorG = 0;
var tiecolorB = 0;
var buttonD= 259;
var hatColorR = 32;
var hatColorG = 69;
var mouthposition = 345;
var eyeSize = 17;
var blushW = 30;
var blushH = 30;
function setup() {
createCanvas(480, 640);
// text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(bgColorR, bgColorG, 255);
noFill();
// head and body
noStroke();
fill(255);
ellipse(240, 680, 400, 600); //body
fill(240);
ellipse(245, 332, 305, 250); //shadow on face
fill(255);
ellipse(240, 300, 300, 300); //main face ellipse
ellipse(285, 350, 250, 200); //cheek
ellipse(140, 200, 120, 120); //left ear
ellipse(340, 205, 120, 120); //right ear
// eyes
stroke(0);
strokeWeight(eyeSize);
point(205, 320); //left eye
point(310, 320); //right eye
// mouth
strokeWeight(5);
fill(255, 0, 0);
arc(260, 345, 50, 50, 0, PI, CHORD);
// necktie and button
fill(tiecolorR, tiecolorG, tiecolorB);
triangle(216, 465, 216, 503, 259, 484.5); //left part of tie
triangle(301, 465, 301, 503, 259, 484.5); //right part of tie
fill(0);
ellipse(buttonD, 510, 5, 5); //top button
ellipse(buttonD, 525, 5, 5); //middle button
ellipse(buttonD, 540, 5, 5); //bottom button
// blush
noStroke();
fill(249, 203, 203);
ellipse(140, 350, blushW, blushH); //left blush
ellipse(360, 350, blushW, blushH); //right blush
// hat
fill(hatColorR, hatColorG, 140);
quad(204, 173, 220, 130, 264, 130, 283, 173);
}
function mousePressed() {
// changes color of background
bgColorR = random(50, 200);
bgColorG = random(50, 200);
// changes position of button
buttonD = random(240, 280);
// changes color of necktie
tiecolorR = random(0, 255);
tiecolorG = random(0, 255);
tiecolorB = random(0, 255);
// changes color of hat
hatColorR = random(20, 230);
hatColorG = random(20, 230);
// changes eye size
eyeSize = random(10, 30);
// changes shape of blush on cheek
blushW = random(30, 45);
blushH = random(30, 45);
}
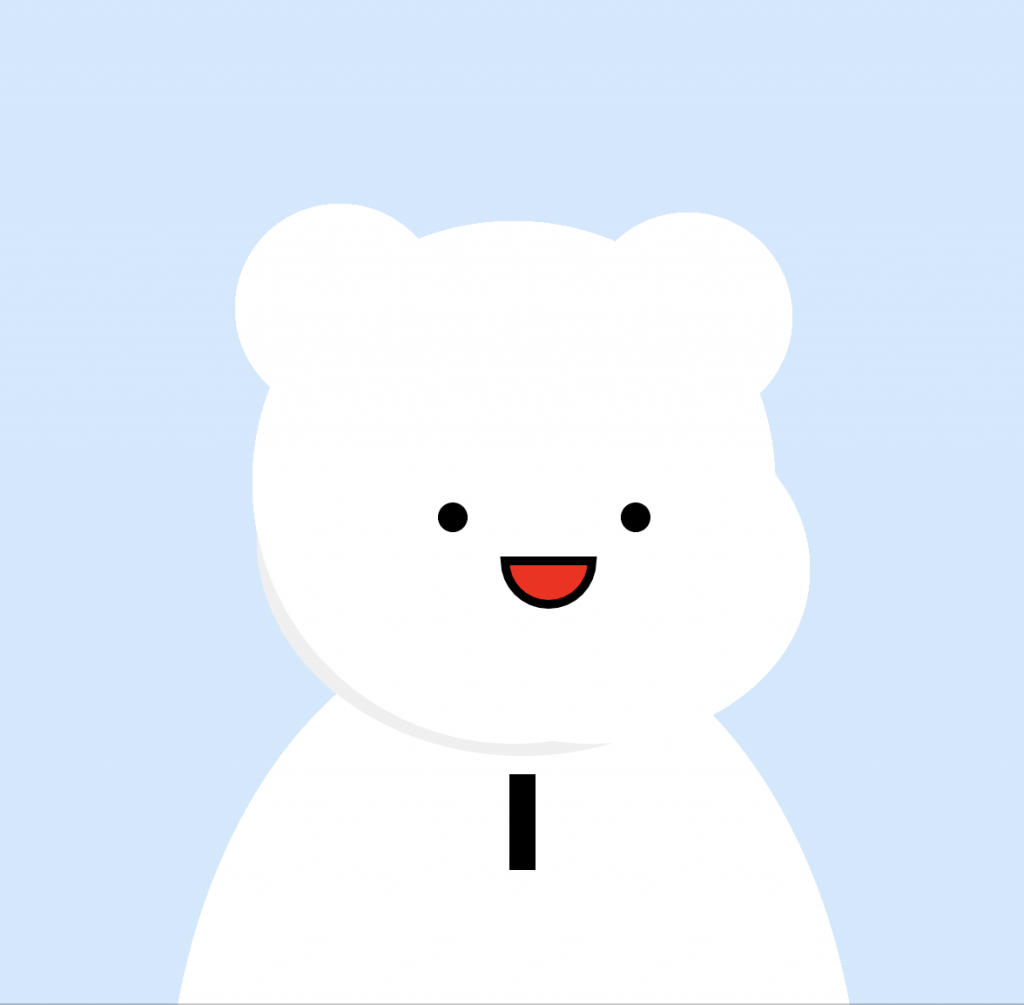