clock
var x = [];
var y = [];
var sec; // second
var minutes; // minute
var hr; // hour
// every second, coffee fills up a little bit in a cup and coffee mug gets larger
// every minute, coffee completely fills up a cup
// every hour, the background color darkens
function setup() {
createCanvas(450, 300);
}
// each cup of coffee represents one minute
function draw() {
var hr = hour();
background(255-(hr*5), 255-(hr*5), 255-(hr*3)); // background color gets darker by the hour
minutes = minute();
sec = second();
for(var row = 0; row < 6; row +=1 ) {
for(var col = 0; col < 10; col += 1) {
fill(250, 247, 222); // cream color for mug
var current = row*10+col; // current mug
mug(40+(40*col), 43+(40*row), current); // draw the mug
}
}
}
function mug(x, y, cur) { // function to draw the mug
// body of the mug
var frac = 1;
if (cur < minutes) {
fill(117, 72, 50); // brown
} else if (cur == minutes) {
frac = sec/60;
fill(250+(117-250)*frac, 247+(72-247)*frac, 222+(50-222)*frac); // cream -> brown
} else {
return;
}
push();
translate(x, y);
scale(frac); // every second, the mug gets bigger
ellipse(0, 0, 30, 7); // top of mug
beginShape();
curveVertex(-15, 0);
curveVertex(-15, 0);
curveVertex(-14, 10);
curveVertex(-10, 25);
curveVertex(-5, 28);
curveVertex(0, 28);
curveVertex(5, 28);
curveVertex(10, 25);
curveVertex(14, 10);
curveVertex(15, 0);
curveVertex(15, 0);
endShape();
// mug handle
noFill();
strokeWeight(1.5);
beginShape();
curveVertex(12, 20);
curveVertex(12, 20);
curveVertex(18, 13);
curveVertex(15, 5);
curveVertex(14, 8);
curveVertex(14, 8);
endShape();
strokeWeight(1);
pop();
}
The idea behind this project stemmed from my love for coffee. My time is basically measured by cups of coffee, which inspired me to create a canvas full of coffee mugs. The hours of each day are represented by the darkening of the background. The minutes of each hour are represented by the sixty mugs. The seconds of each minute are represented by the size and color of the mugs (filling up the mugs).
My initial sketch:
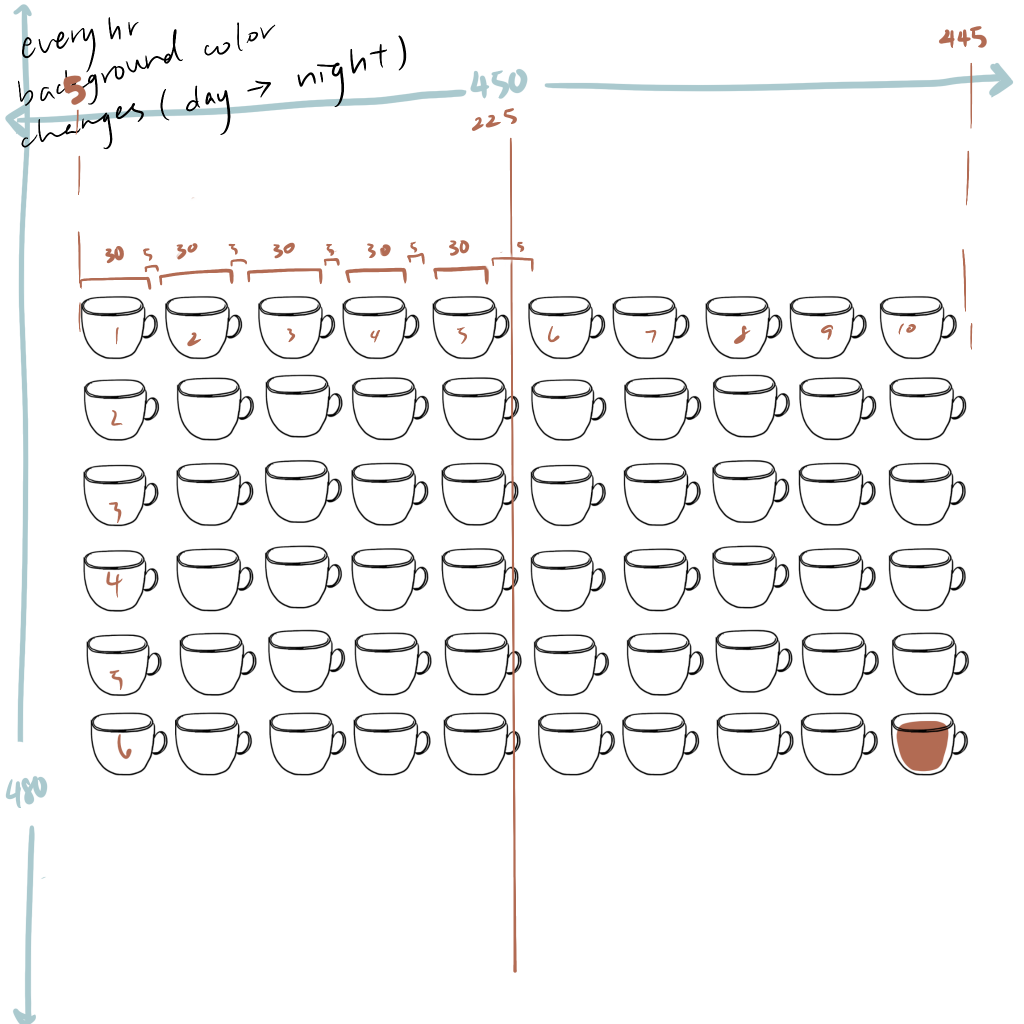
I found this project quite challenging. I realized that executing a concept in code can be a lot more difficult than expected.