As winter approaches, I wanted create a landscape that resembles the cold weather and loneliness of the season. That’s why I decided to keep a greyscale color scheme.
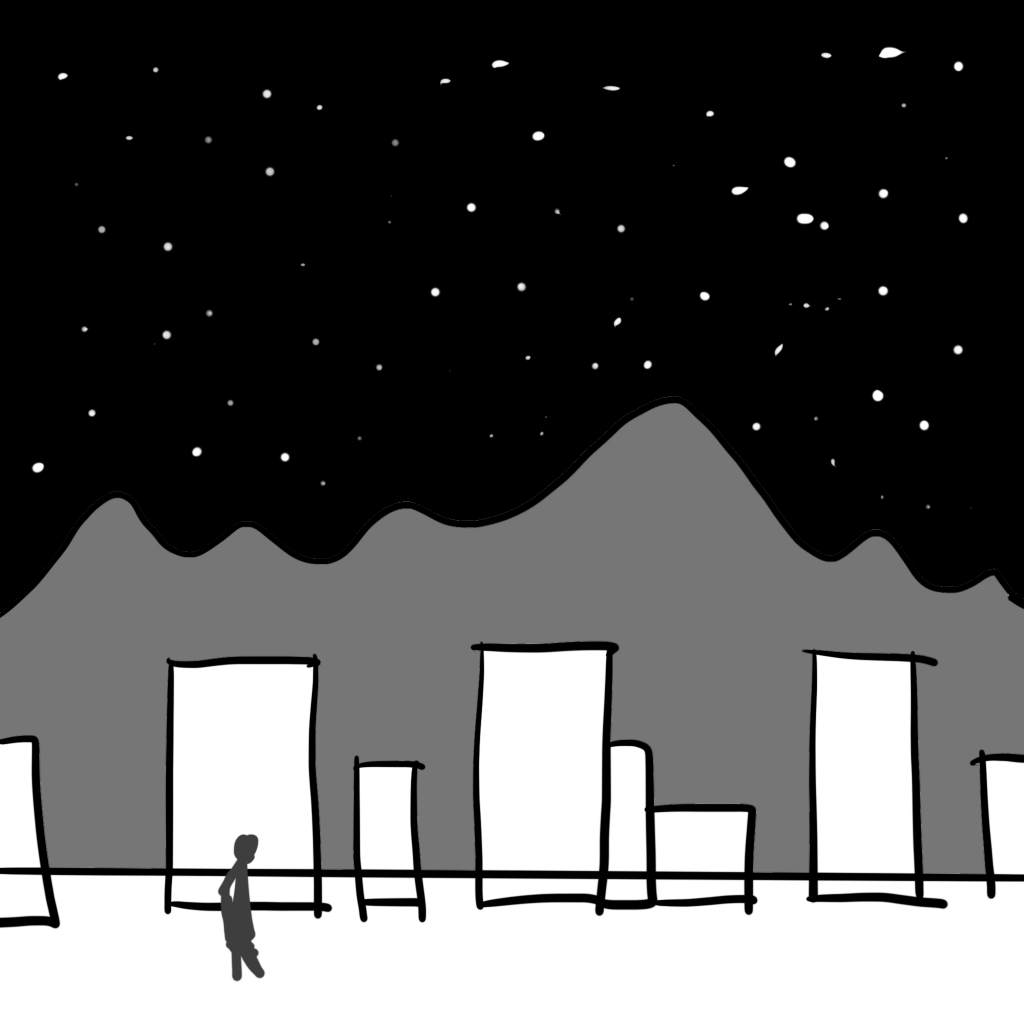
sketch
// Christy Zo
// andrew id: czo
// Section C
var hill = [];
var hill2 = [];
var noiseParam = 0;
var noiseStep = 0.05;
var noiseParam2 = 0;
var noiseStep2 = 0.05
var snowflakePerLayer = 200;
var maxSize = 3;
var gravity = 0.75;
var layerCount = 5;
var snowflakes = [];
var walkImage = [];
var newCharacter = [];
var airplane;
var newPlane = [];
var buildings = [];
function preload() {
var filenames = [];
filenames[0] = "https://i.imgur.com/Uz3ltZC.png";
filenames[1] = "https://i.imgur.com/ofNc4Wv.png";
filenames[2] = "https://i.imgur.com/7hMA7W6.png";
filenames[3] = "https://i.imgur.com/3RBTODb.png";
filenames[4] = "https://i.imgur.com/FliwTsj.png";
for (var i = 0; i < filenames.length; i++) {
walkImage[i] = loadImage(filenames[i]);
}
airplane = loadImage("https://i.imgur.com/dbPwlhp.png");
}
//airplane
function makePlane(px, pdx, py, pdy) {
var p = {x: px, dx: pdx, y:py, dy: pdy,
stepFunction: stepPlane,
drawFunction: drawPlane
}
return p;
}
function stepPlane() {
this.x += this.dx;
this.y += this.dy;
if (this.x > 480) {
this.x = 0;
this.y = 100;
}
}
function drawPlane() {
image(airplane, this.x, this.y, 20, 20);
}
//person
function makeCharacter(cx, cdx) {
var c = {x: cx, dx: cdx,
// walkingRight: true,
imageNum: 0,
stepFunction: stepCharacter,
drawFunction: drawCharacter
}
return c;
}
function stepCharacter() {
this.x += this.dx;
this.imageNum += 1;
if (this.imageNum == 4 ) {
this.imageNum = 0;
}
if (this.x >= 680) {
this.x = 200;
}
}
function drawCharacter() {
image(walkImage[this.imageNum], this.x, 430);
}
//snowflake update
function updateSnowflake(snowflake) {
var diameter = (snowflake.l * maxSize) / layerCount;
if (snowflake.y > height + diameter) {
snowflake.y = -diameter;
} else {
snowflake.y += gravity * snowflake.l * snowflake.mass;
}
}
//buildings
function updateAndDisplayBuildings(){
// Update the building's positions, and display them.
for (var i = 0; i < buildings.length; i++){
buildings[i].move();
buildings[i].display();
}
}
function removeBuildingsThatHaveSlippedOutOfView(){
var buildingsToKeep = [];
for (var i = 0; i < buildings.length; i++){
if (buildings[i].x + buildings[i].breadth > 0) {
buildingsToKeep.push(buildings[i]);
}
}
buildings = buildingsToKeep; // remember the surviving buildings
}
function addNewBuildingsWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newBuildingLikelihood = 0.007;
if (random(0,1) < newBuildingLikelihood) {
buildings.push(makeBuilding(width));
}
}
function buildingMove() {
this.x += this.speed;
}
// draw the building and some windows
function buildingDisplay() {
var floorHeight = 20;
var bHeight = this.nFloors * floorHeight;
fill(255);
noStroke;
push();
translate(this.x, height - 40);
rect(0, -bHeight, this.breadth, bHeight);
stroke(200);
fill(200);
for (var i = 0; i < this.nFloors; i++) {
rect(5, -15 - (i * floorHeight), this.breadth - 10, 10);
}
pop();
}
function makeBuilding(birthLocationX) {
var bldg = {x: birthLocationX,
breadth: 50,
speed: -1.0,
nFloors: round(random(2,8)),
move: buildingMove,
display: buildingDisplay}
return bldg;
}
function setup() {
createCanvas(480, 480);
noStroke();
frameRate(20);
for (i=0; i<width/5+1; i++) {
var n = noise(noiseParam);
var value = map(n, 0, 1, 100, height);
hill.push(value);
noiseParam += noiseStep;
}
// snowflakes
for (let l = 0; l < layerCount; l++) {
snowflakes.push([]);
for (let i = 0; i < snowflakePerLayer; i++) {
snowflakes[l].push({
x: random(width),
y: random(height),
mass: random(0.75, 1.25),
l: l + 1
});
}
}
for (var i = 0; i < 10; i++){
var rx = random(width);
buildings[i] = makeBuilding(rx);
}
imageMode(CENTER);
var d = makeCharacter(50, 1);
newCharacter.push(d);
var p = makePlane(10, 5, 100, -1);
newPlane.push(p);
}
function draw() {
background(0);
for (let l = 0; l < snowflakes.length; l++) {
var layer = snowflakes[l];
for (let i = 0; i < layer.length; i++) {
var snowflake = layer[i];
fill(255);
circle(snowflake.x, snowflake.y, (snowflake.l * maxSize) / layerCount);
updateSnowflake(snowflake);
}
}
beginShape();
vertex(0, height);
for (i=0; i<width/5+1; i++) {
noStroke();
fill(200);
vertex(i*5, hill[i]);
}
vertex(width, height);
endShape();
var n = noise(noiseParam);
var value = map(n, 0, 1, 100, height);
hill.shift();
// append(hill, value);
hill.push(value);
noiseParam += noiseStep;
fill(150);
rect(0, 430, width, height);
updateAndDisplayBuildings();
removeBuildingsThatHaveSlippedOutOfView();
addNewBuildingsWithSomeRandomProbability();
newCharacter[0].stepFunction();
newCharacter[0].drawFunction();
newPlane[0].stepFunction();
newPlane[0].drawFunction();
// image(walkImage[1], 100,100);
}
*Due to the way I saved my png drawing of the person, you have to wait a little long for the person to appear!*
After I created this work, I realized that the mountain behind moves significantly faster than everything else, making it look like this space is not on earth. Maybe this could be what winter looks like in a different planet.