var plastic = ["https://i.imgur.com/n3n2yAx.png",
"https://i.imgur.com/GO1fho6.png",
"https://i.imgur.com/HTF7LVN.png",
"https://i.imgur.com/lPCz5Kl.png",
"https://i.imgur.com/uOAHfcT.png",
"https://i.imgur.com/714d3E7.png",
"https://i.imgur.com/mxW1JT4.png",
"https://i.imgur.com/2diHO2t.png",
"https://i.imgur.com/YfCSzcQ.png",
"https://i.imgur.com/TZovsxa.png",
"https://i.imgur.com/lK07FmR.png"];
var waste1;
var waste2;
var waste3;
var waste4;
var waste5;
var waste6;
var waste7;
var waste8;
var waste9;
var waste10;
var waste11;
var humanfiles = [];
var human = [];
var vehiclefiles = [];
var vehicles = [];
var wheel;
var instruction;
var newBackground = [];
var newCharacter = [];
var c1,c2;
var mode = false;
var instructionMode = true;
function preload() {
//waste images
waste1 = loadImage(plastic[0]);
waste2 = loadImage(plastic[1]);
waste3 = loadImage(plastic[2]);
waste4 = loadImage(plastic[3]);
waste5 = loadImage(plastic[4]);
waste6 = loadImage(plastic[5]);
waste7 = loadImage(plastic[6]);
waste8 = loadImage(plastic[7]);
waste9 = loadImage(plastic[8]);
waste10 = loadImage(plastic[9]);
waste11 = loadImage(plastic[10]);
//characters
humanfiles[0] = "https://i.imgur.com/gGl600Z.png";
humanfiles[1] = "https://i.imgur.com/6yqtw3q.png";
for (var i = 0; i < humanfiles.length; i++) {
human[i] = loadImage(humanfiles[i]);
}
//vehicle
vehiclefiles[0] = "https://i.imgur.com/gnO3lJm.png";
vehiclefiles[1] = "https://i.imgur.com/S1GOMdC.png";
for (var i = 0; i < vehiclefiles.length; i++) {
vehicles[i] = loadImage(vehiclefiles[i]);
}
wheel= loadImage("https://i.imgur.com/Wkpp7B1.png");
instruction = loadImage("https://i.imgur.com/QcOMxYC.png");
}
//Factory made of waste
function generateBackground(bx, bdx) {
var b = {
x: bx,
y:height-75,
dx: bdx,
items: random([waste1, waste2, waste3, waste4, waste5, waste6, waste7, waste8, waste9, waste10, waste11]),
moveFunction: moveBackground,
drawFunction: drawBackground
}
return b;
}
function moveBackground() { //generative landscape
if (keyIsDown(RIGHT_ARROW)) {
this.x -= this.dx;
} else if (keyIsDown(LEFT_ARROW)) {
this.x += this.dx;
}
}
function drawBackground() {
var smallSize = 20;
var bigSize = 30
push();
if (this.items == waste1) {
image(waste1, this.x, 325-waste1.height);
} else if (this.items == waste2) {
image(waste2, this.x, 325-waste2.height);
} else if (this.items == waste3) {
image(waste3, this.x, 325-waste3.height);
} else if (this.items == waste4) {
image(waste4, this.x, 325-waste4.height);
} else if (this.items == waste5) {
image(waste5, this.x, 325-waste5.height);
} else if (this.items == waste6) {
image(waste6, this.x, 325-waste6.height);
} else if (this.items == waste7) {
image(waste7, this.x, 325-waste7.height);
} else if (this.items == waste8) {
image(waste8, this.x, 325-waste8.height);
} else if (this.items == waste9) {
image(waste9, this.x, 325-waste9.height);
} else if (this.items == waste10) {
image(waste10, this.x, 325-waste10.height);
} else if (this.items == waste11) {
image(waste11, this.x, 325-waste11.height);
}
pop();
}
function itemShow() {
for(var i = 0; i < 100; i++) {
newBackground[i].moveFunction();
newBackground[i].drawFunction();
}
}
//Character skating
function makeSkateCharacter (cx, cdx) {
var c = {
x: cx,
dx: cdx,
y: height-150,
movingRight: true,
stepSkateFunction: stepSkateCharacter,
drawSkateFunction: drawSkateCharacter
}
return c;
}
function stepSkateCharacter () {
if (keyIsDown(RIGHT_ARROW)) {
this.movingRight = true;
if (this.x < width/2) {
this.x += this.dx;
}
}
}
function drawSkateCharacter () {
image(vehicles[0], this.x-25, height-80, 70, 25);
image(human[0], this.x, this.y);
}
//Character riding bike
function makeBikeCharacter (mx, wmx, mdx) {
var m = {
x: mx,
wx: wmx,
dx: mdx,
y: height-150,
movingRight: true,
stepBikeFunction: stepBikeCharacter,
drawBikeFunction: drawBikeCharacter,
}
return m;
}
function stepBikeCharacter () {
if (keyIsDown(RIGHT_ARROW)) {
this.movingRight = true;
push(); //bike wheel rotating
imageMode(CENTER);
translate(this.wx+18, this.y+73);
r+=0.5;
rotate(r);
image(wheel, 0, 0, 35, 35);
pop();
push();
imageMode(CENTER);
translate(this.wx+103, this.y+73);
r+=1;
rotate(r);
image(wheel, 0, 0, 35, 35);
pop();
if (this.x < width/2) {
this.x += this.dx;
}
}
}
var r = 0;
function drawBikeCharacter () {
if (this.movingRight == true) {
image(vehicles[1], this.x-40, height-120, 120, 60);
image(human[1], this.x, this.y);
} else {
push();
translate(600, 0);
scale(-1, 1);
image(vehicles[1], this.x-25, height-80, 200, 100);
image(human[1], this.x, this.y);
pop();
}
}
function setup() {
createCanvas(600, 400);
frameRate(15);
var n = 0;
for (var i = 0; i < 100; i++) {
var x = generateBackground(n, 5);
newBackground.push(x);
if (newBackground[i].items == waste1) { //placement of each waste factory
n += waste1.width-2;
} else if (newBackground[i].items == waste2) {
n += waste2.width-2;
} else if (newBackground[i].items == waste3) {
n += waste3.width-2;
} else if (newBackground[i].items == waste4) {
n += waste4.width-2;
} else if (newBackground[i].items == waste5) {
n += waste5.width-2;
} else if (newBackground[i].items == waste6) {
n += waste6.width-2;
} else if (newBackground[i].items == waste7) {
n += waste7.width-2;
} else if (newBackground[i].items == waste8) {
n += waste8.width-2;
} else if (newBackground[i].items == waste9) {
n += waste9.width-2;
} else if (newBackground[i].items == waste10) {
n += waste10.width-2;
} else if (newBackground[i].items == waste11) {
n += waste11.width-2;
}
}
var d = makeSkateCharacter(width/2, 3);
var e = makeBikeCharacter(width/2, width/2-40, 5);
newCharacter.push(d);
newCharacter.push(e);
}
function draw() {
var cAmount = 100;
c1 = color(80);
//gradient color change & fleeting effect on the factory in sustainable future
c1.setAlpha(10 + 10 * sin(millis() / 1000));
c2 = color(230);
c4 = color(225,247,255);
c3 = color(0, 171, 245);
c3.setAlpha(10 + 10 * sin(millis() / 1000));
var year = 2050;
var title = "WHICH FUTURE DO YOU CHOOSE TO LIVE IN?";
noStroke();
if (mode) {
for(let y=0; y<height; y++){ //gradient background
n = map(y,0,height,0,1);
let newc = lerpColor(c3,c4,n);
stroke(newc);
line(0,y,width, y);
}
fill(255);
textSize(270);
text(year, width/2, height-82);
textSize(25);
textAlign(CENTER);
text(title, width/2, height-300);
itemShow();
fill(100);
noStroke();
rect(0, height-76, width, 76);
newCharacter[0].stepSkateFunction();
newCharacter[0].drawSkateFunction();
} else {
background(0);
for(let y=0; y<height; y++){
n = map(y,0,height,0,1);
let newc = lerpColor(c1,c2,n);
stroke(newc);
line(0,y,width, y);
}
fill(255);
textSize(270);
text(year, width/2, height-82);
textSize(25);
textAlign(CENTER);
text(title, width/2, height-300);
itemShow();
fill(100);
noStroke();
rect(0, height-76, width, 76);
newCharacter[1].drawBikeFunction();
newCharacter[1].stepBikeFunction();
}
if (instructionMode) {
push();
imageMode(CENTER);
image(instruction, width/2, height/2);
pop(); }
if (keyIsDown(81)) { //show instruction page
push();
imageMode(CENTER);
image(instruction, width/2, height/2);
pop();
}
}
//toggle between bike and skate
function keyPressed() {
if (key == ' ') {
if (mode == false) {
mode = true;
} else if (mode == true) {
mode = false;
}
}
}
function mousePressed() {
if (instructionMode == true){
instructionMode = false;
}
}
When I heard that the topic for this project is climate change, I was instantly reminded of previous poster work on air pollution, in which I used everyday waste material to draw factories as a metaphor.
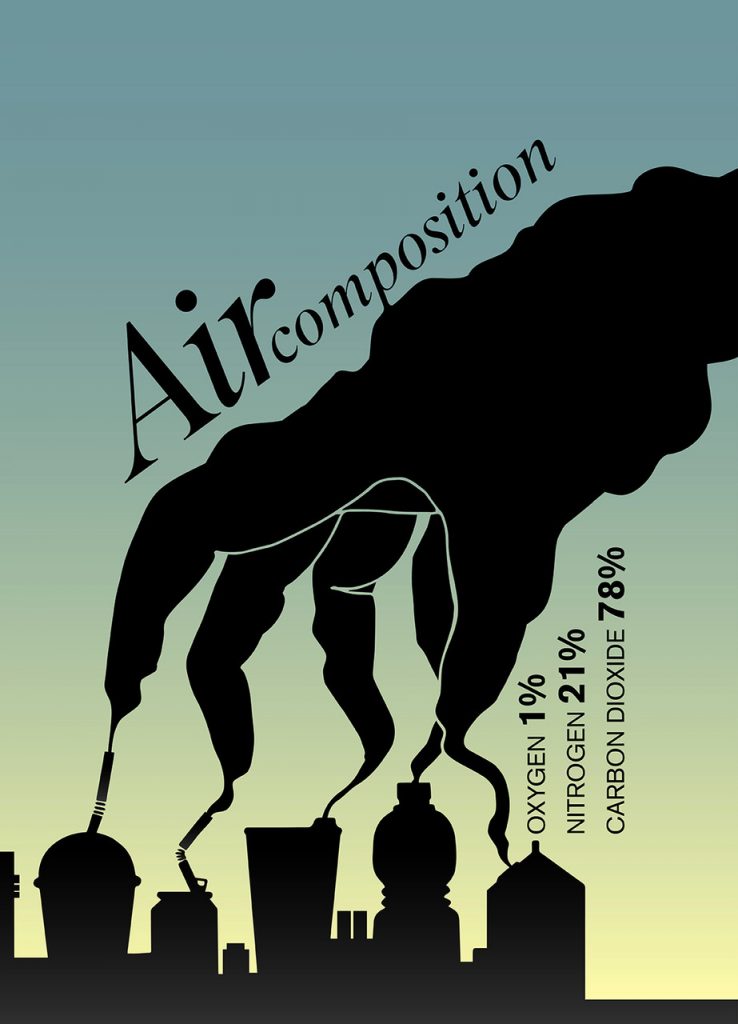
This program is a future simulator that shows two alternative versions of 2050’s air quality based how sustainable our lifestyle is. I wanted to continue this use of symbols, so I decided to create a character that interacts with this environment by creating a character that rides a vehicle that is also a metaphor for wasteful lifestyle and a sustainable one. The user of this program can toggle between a character that rides a skateboard made out of a reusable water bottle and a character that rides a motorcycle made of waste like aluminum can wheels. As the user presses down the right arrow key, they can see that the character is moving in the generative landscape. As they toggle the space bar, they can change the vehicle, and see how the air quality changes depending on how much single use products we use. In the sustainable future factories made out of trash starts to wither away, in contrast to how static they are in the polluted version. The main goal of this program is to alert people of the drastic difference our everyday behavior can make to our environment and future and provoke action.in goal of this program is to alert people of the drastic difference our everyday behavior can make to our environment and future and provoke action.