var nPoints = 100;
var x = 1;
function setup() {
createCanvas(480, 480);
textSize(20);
textAlign(CENTER, CENTER)
}
function draw() {
background(0);
noStroke();
//draws text on screen that changes with every mouse click
if (x == 1) {
fill(255, random(200), random(200), 220);
text('我爱你', width/2, height/2);
} else if (x == 2) {
fill(255, random(200), random(200), 220);
text('i love you', width/2, height/2);
} else if (x == 3) {
fill(255, random(200), random(200), 220);
text('te amo', width/2, height/2);
} else if (x == 4) {
fill(255, random(200), random(200), 220);
text('사랑해', width/2, height/2);
} else if (x == 5) {
fill(255, random(200), random(200), 220);
text('愛してる', width/2, height/2);
} else if (x == 6) {
fill(255, random(200), random(200), 220);
text('♡', width/2, height/2);
}
//the code below this line starts drawing curves
//draws first curve/heart in top left corner
fill(188, 0, 56, 200);
drawEpitrochoidCurve();
//draws curve/heart in top right corner
push();
translate(width, 0);
drawEpitrochoidCurve();
pop();
//draws curve/heart in bottom left corner
push();
translate(0, height);
drawEpitrochoidCurve();
pop();
//draws curve/heart in bottom right corner
push();
translate(480, 480);
drawEpitrochoidCurve();
pop();
}
function mousePressed(){
if (x == 1){
x = 2;
} else if (x == 2){
x = 3;
} else if (x == 3){
x = 4;
} else if (x == 4){
x = 5;
} else if (x == 5){
x = 6;
} else if (x == 6){
x = 1;
}
}
function drawEpitrochoidCurve() {
// Epicycloid:
// http://mathworld.wolfram.com/Epicycloid.html
var x;
var y;
var a = map(mouseX/3, 0, width, 0, 500);
var b = a / 8;
var h = constrain(mouseY / 8.0, 0, b);
beginShape();
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x = (a + b) * cos(t) - h * cos(((a + b) / b) * t);
y = (a + b) * sin(t) - h * sin(((a + b) / b) * t);
vertex(x, y);
}
endShape();
}
Author: meep
Looking Outwards: Unnumbered Sparks
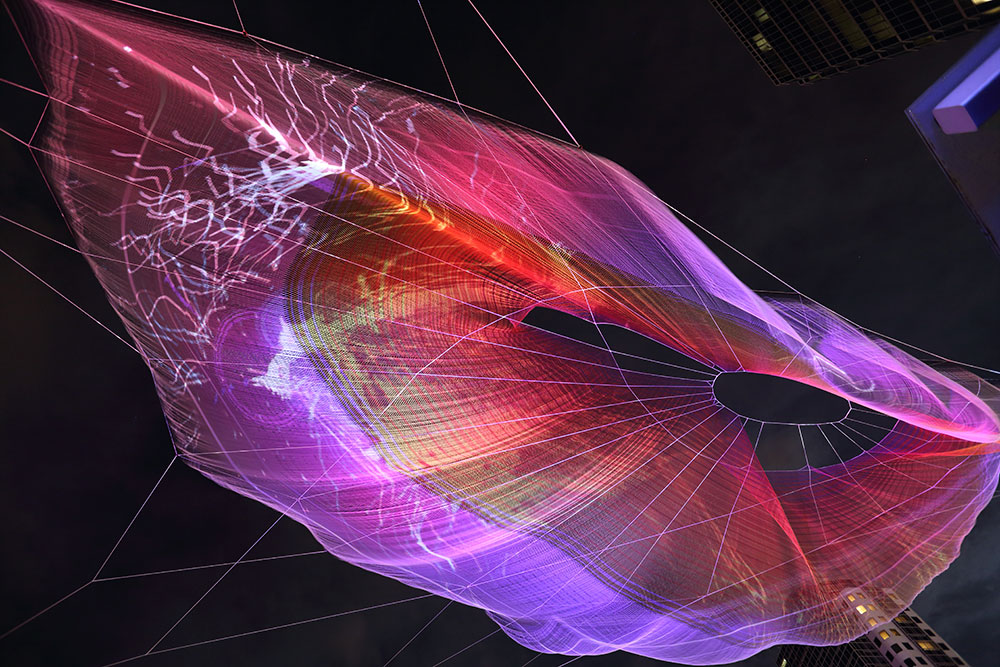
The project I chose to look at this week was Aaron Koblin’s Unnumbered Sparks, when I first visited his website I was immediately drawn to the striking visuals of the piece. On a surface level, it looks like a massive, ethereal, floating structure reminiscent of a net. But it isn’t just so, as the colors and striking visuals that are displayed across this structure are all controlled in the background by a single website. I really admire how it is an artwork that engages its viewers and promotes interactions between viewers themselves, as well as, between viewers and the artwork. By logging onto a website, people are enabled to draw their own distinct patterns or visuals which are then projected up onto this sculpture.
It was impressive the amount of thought given into the piece, not only from a computational standpoint. There were also considerations of how the sculpture would interact with the environment and environmental factors such as the wind. It also looked at the different experiences it would create for people depending on the distance at which they view the sculpture. Behind all these considerations, is the computational aspect itself which lent itself not only to the aesthetic of the final artwork but also the ideation and planning of the artwork too. Koblin described the process in where they built the whole 3D modeling environment itself directly in Chrome through WebGL, using that as a tool to help them sample light from the virtual sculpture and project that onto the real life object.
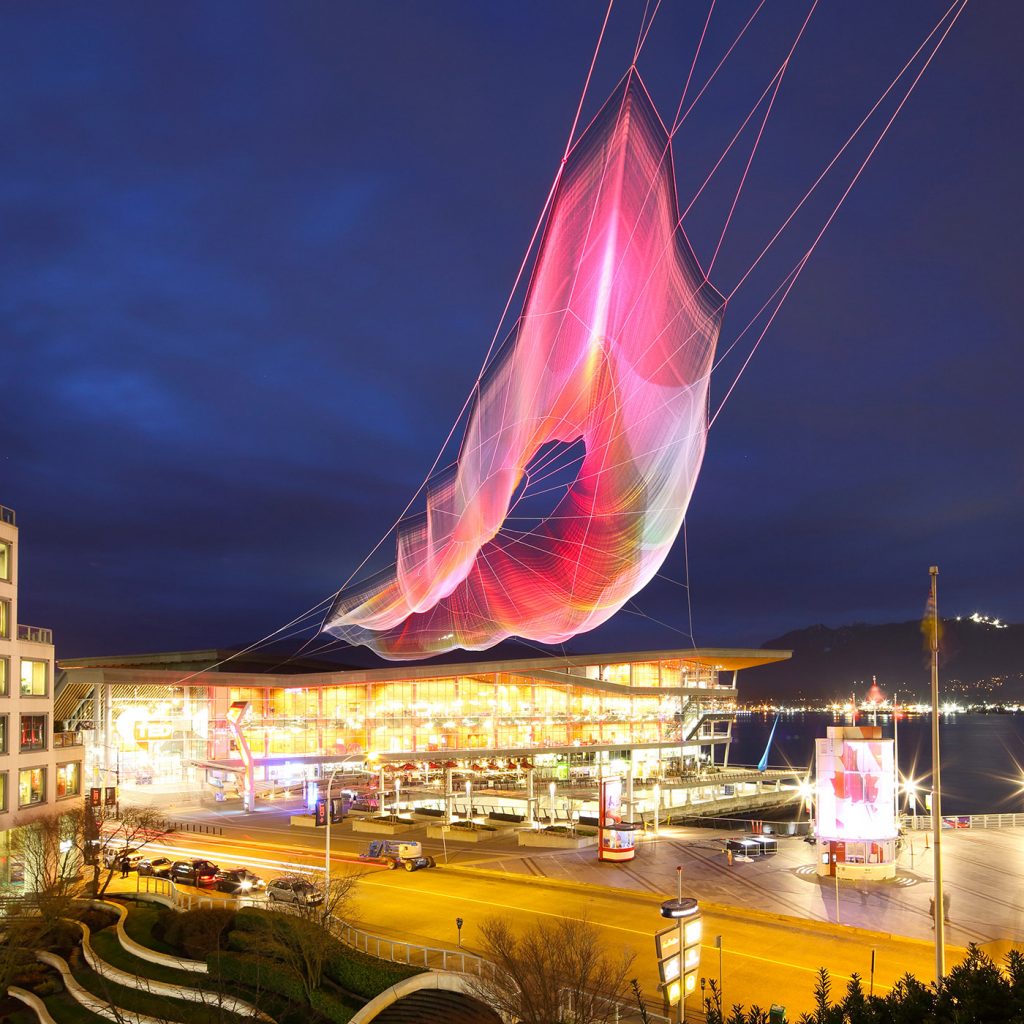
Project 6: Abstract Clock
For my project, I decided to create an abstract clock that tells the time through an animated frog. In this project, the frog slow closes its eyes following every passing second, and when a minute passes its eyes jump back to their starting position. The background itself is programmed to change subtly for every minute that passes. At the bottom of the canvas are blades of grass, one representing each hour that has already passed in the day.
var flyX = 225;
var flyY = 0;
function setup() {
createCanvas(480, 480);
background(255, 220, 220);
ellipseMode(CENTER);
rectMode(CENTER);
}
//function that draws the frog on canvas
function frog(){
//frog body
stroke(163, 207, 144);
fill(163, 207, 144);
quad(20, 480, 460, 480, 340, 308, 140, 308);
//frog head
stroke(176, 217, 159);
fill(176, 217, 159);
ellipse(width/2, height/2, 360, 302);
ellipse(130, 130, 126, 120);
ellipse(350, 130, 126, 120);
//frog blush
stroke(240, 142, 117)
fill(240, 142, 117);
ellipse(70, 218, 83, 46);
ellipse(410, 218, 83, 46);
}
//function for the flies that surround the frog
function flies(x, y){
translate(width/2, height/2)
for(var i = 0; i < 24; i++){
push();
rotate(TWO_PI*i/24);
fill(0);
rect(x, y, 1, 1);
pop();
}
for(var i = 0; i < 12; i++){
push();
noStroke();
fill(0);
rotate(TWO_PI*i/12);
rect(x, y, 5, 5)
pop();
}
}
function grass(){
var x = 0;
var hr = hour();
for(var x = 0; x <= 20*(hr-1); x += 20){
fill(61, 118, 37);
triangle(x, 480, x+20, 480, x+10, 460);
}
}
function draw() {
//initializaing variables for time
var sec = second();
var min = minute();
var hr = hour();
var eyeX = 56;
var eyeY = 66;
background(min*5, min*3.5, min*3);
//draws the frog on to the canvas
frog();
//draws blades of grass on canvas that represent the hour of the day
noStroke();
grass();
//frog mouth and eyes
stroke(0);
strokeWeight(2);
fill(0);
ellipse(350, 140, eyeX, eyeY-sec**1);
ellipse(130, 140, eyeX, eyeY-sec**1);
line(210, 168, 270, 168);
//calling function to draw flies
flies(flyX, flyY);
flyX += random(-1, 1);
flyY += random(-1, 1);
//draws blades of grass
}
Looking Outwards-06
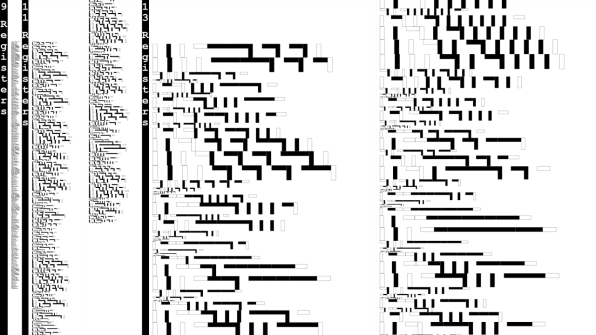
In looking at the application of randomness in generative art this week, I looked at Rami Hammour’s work on A Text of Random Meaning. It’s a visualization mapping out 18 columns of a “Register and Taps” random number generator in action. I found it interesting the way the final visualization simulates the look of long columns of text, but once viewers zoom in they find that the randomly generated lines are not actually representative of anything. I found the irony in this interaction very interesting. However, one of the interesting points I came across in my research was that technically in it’s simulated nature, there is no such thing as true randomness. In descriptions of Rami Hammour’s project, it is described as a “systematic cycle for producing randomness”.
LO: 3D Computer Graphics
The project I was particularly struck by while looking through 3D artists is called “Transit” and was created by Michael Kim, or maikoolk, as his social media handles read. It’s a render of what is supposed to look like the inside of a train car, with certain labels of the train car being changed to represent details relevant to his personal life, or simply to take on personal meaning to him. Across the walls of the car are writings in graffiti, that also seem to carry personal meanings to him.
All in all I found it admirable that he took his talent in creating 3D graphics and
used it to create a piece of artwork that held personal meaning to him, in a way
creating this unique and personable artifact for himself. Besides that, it was very cool to see the amount of detail that he was putting into his work. Everything from the detail in the lighting to the texture of the walls and seats. There seemed to be a very particular grainy and moody look he was going for in his work and it all came out very nicely.
LO 4: Sound Art
The Cotodama lyric speaker was produced to act as a canvas that would digitally display the lyrics to any song playing through its speakers. Although this doesn’t exactly fall under the classification of computationally generated music, I feel like this is a product that works under very similar mechanics.
I like the product itself due to its elegant look, though it looks as if it comes as two separate pieces, it is actually one solid unit designed to look like vinyl record jackets leaned up against the wall. I also think it’s a great example of sound data being used in coordination with databases to create visuals. When connected to an audio source, its display panel sources lyrics from databases
and displays the lyrics in sync with the song. It also is programmed to analyze a song’s mood to pick corresponding fonts and animations. In cases where no lyrics can be found for a song, the speaker will display AI-generated animations that move in sync with the music instead.
https://lyric-speaker.com/en/history/
Looking Outwardss-03
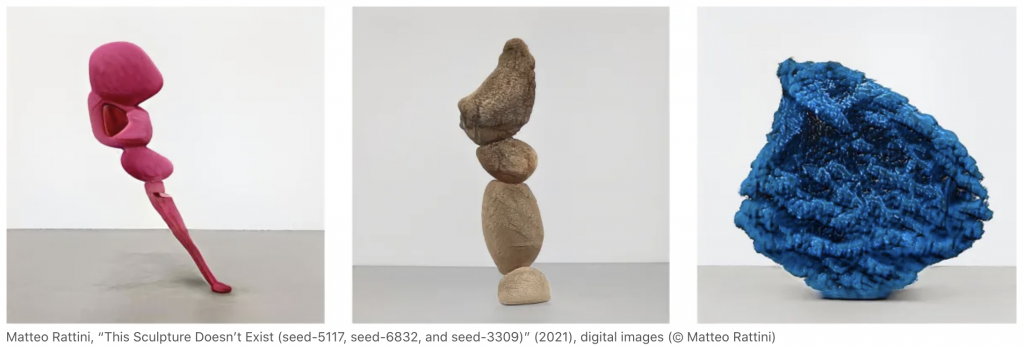
One of the things I’ve found very interesting is the use of AI in generative art,
whether in the 2D or 3D sense. I recently came across DALL-E a neural network
that was programmed to be able to generate images from user-provided text
captions. These captions could be as arbitrary as possible, used to create 2D or
even 3D images.
Following my curiosity in similar computational programs, I found artist Matteo Rattini, who similarly trained a neural network to create images of contemporary sculptures based on the Instagram algorithm. The sculptures themselves were all very interesting, taking on forms that seemed organic or close to those seen in nature.
One of the most intriguing points of this program was how it related to
current society’s behavioral patterns and trends. Because sculptures were generated based on a user’s Instagram algorithm, what Rattini found was that while Instagram suggestions would initially be wide and diverse they would always eventually reduce down to a very narrow range of things, causing later sculptures to eventually all look the same. In many ways, I find this interesting, in that while creating sculptures the neural network is also subliminally reflecting the ways users interact with social media as well as patterns in current trends.
More about Rattini’s work: https://hyperallergic.com/667011/art-of-ai-matteo-rattini-eerily-real-computer-generated-sculptures/
Project 3: Dynamic Drawing
var r = 161;
var g = 242;
var b = 228;
var eyesX = 5;
var eyesY =7;
s
function setup() {
createCanvas(600, 450);
background(r, g, b);
fill(255);
text("ribbit", 550, 430);
}
function draw() {
//setup for color of the water
background(r, g, b, 100);
noFill();
stroke(255);
strokeWeight(0.5);
ellipse(mouseX, mouseY, 50, 50);
if(mouseX<200){
background(17, 38, 92, 100);
} else if (mouseX<400){
background(26, 147, 139, 100);
}
//this draws water ripples
noFill();
stroke(255);
//left most ripple
ellipse(5, height/2, 200*mouseX*0.01, 200*mouseX*0.01);
ellipse(5, height/2, 200*mouseX*0.004, 200*mouseX*0.004);
//bottom ripple
ellipse(300, 360, 200*mouseX*0.01, 200*mouseX*0.01);
ellipse(300, 360, 200*mouseX*0.004, 200*mouseX*0.004);
//right ripple
ellipse(580, 160, 200*mouseX*0.01, 200*mouseX*0.01);
ellipse(580, 160, 200*mouseX*0.004, 200*mouseX*0.004);
//draws lilypads above the water
noStroke();
fill(112, 221, 85, 255);
if(mouseX<200){
fill(21, 57, 42, 255);
} else if (mouseX<400) {
fill(25, 131, 36, 255);
}
ellipse(70, 90, 150, 95);
ellipse(190, 50, 190, 110);
ellipse(300, 280, 165, 110);
ellipse(80, 400, 170, 140);
ellipse(550, 90, 230, 120);
ellipse(430, 135, 160, 95);
ellipse(530, 430, 220, 130);
//draws shadow of lilypads
fill(112, 221, 85, 70);
if(mouseX<200){
fill(21, 57, 42, 70);
} else if (mouseX<400) {
fill(25, 131, 36, 70);
}
ellipse(60, 90, 130, 95);
ellipse(180, 50, 190, 110);
ellipse(290, 280, 165, 110);
ellipse(70, 400, 170, 140);
ellipse(540, 90, 230, 120);
ellipse(420, 135, 160, 95);
ellipse(520, 430, 220, 130);
//draws frog figure
fill(63, 190, 31, 255);
if(mouseX<200){
fill(11, 129, 80);
} else if(mouseX<400){
fill(9, 101, 18);
}
rect(40, 60, 50, 50);
rect(40, 50, 20, 20);
rect(70, 50, 20, 20);
rect(77, 89, 21, 21);
rect(30, 89, 21, 21);
//frog tummy
fill(119, 202, 137);
rect(51, 85, 28, 25);
//frog eyes
fill(255);
rect(45, 52, 10, 10);
rect(75, 52, 10, 10);
//frog pupils
fill(0);
rect(47, 55, eyesX, eyesY);
rect(77, 55, eyesX, eyesY);
rect(50, 70, 29, 2);
rect(39, 96, 1, 14);
rect(89, 96, 1, 14);
//draws frog under water
fill(63, 190, 31, 255);
if(mouseX<200){
fill(11, 129, 80);
} else if(mouseX<400){
fill(9, 101, 18);
}
rect(40+mouseX*0.5, 190, 60, 20);
rect(40+mouseX*0.5, 180, 20, 20);
rect(80+mouseX*0.5, 180, 20, 20);
//frog eyes
fill(255);
rect(45+mouseX*0.5, 182, 10, 10);
rect(85+mouseX*0.5, 182, 10, 10);
//frog pupils
fill(0);
rect(47+mouseX*0.5, 185, eyesX, eyesY);
rect(87+mouseX*0.5, 185, eyesX, eyesY);
rect(55+mouseX*0.5, 200, 29, 2);
}
Project 1: My Self Portrait
LO: My Inspiration
As a student in the School of Design, one of the crucial pieces of works
most students end up creating portfolios of their work as a way to showcase
their abilities and projects. For the one opportunity to program something
purely “yourself” and put your work on display to potential employers, I’ve
seen many examples of student portfolios created in Javascript where its
combination with HTML and CSS create beautiful, personalized and responsive
portfolios.
I admire the work mostly because with each individual they are enabled through
code to create something that is unique and personalized towards them, but at
the same time something delightful for users/site visitors to experience as well.
Although there isn’t a single website on its own I am speaking for in this
writing, there are definitely memorable websites I have seen while browsing
through portfolios. Some students implement elements such as interactive interfaces
that imitate text exchanges, keeping users entertained while they navigate their
sites. Other examples, implement animation to bring static sites to life and guide
users through their content.
These portfolios range in how they use code in their creative practices and also
vary based on complexity. To my knowledge, they’re generally self-directed and
self-led projects that stem from someone’s creative side, and I find it a very
interesting harmony between coding and creative arts.
Some of the portfolios I’ve found especially interesting include:
https://dorcas.cargo.site/
https://www.iamonlyfranklin.com/
https://www.tayaras.com/