//Garrett Rauck
//Section C
//grauck@andrew.cmu.edu
//Project-04
/////////////////////////////
// DECLARE VARS
/////////////////////////////
//canvas
var canvasWidth, canvasHeight, cx, cy, margin
//mouse
var xm, xy; //constrained mouseX and mouseY\
//color
var bgColor, nColor, hColor; //background, normal, and highlight colors
//Spiral
var r0, theta0; //initial values for recursive spiral
/////////////////////////////
// HELPER FNS
/////////////////////////////
function drawSpiralArt(r, theta) {
//mouseX controls amount by which spiral lines are decimating
//in length with each recursive call
var reductionFactor = map(xm,0,canvasWidth,0,1);
//mouseY controls rotational increment between strings in the
//spiral. Varying this by numbers which are not factors of 2*pi
//leads to different types of spirals.
var angleIncrement = int(map(xy, 0, canvasHeight, 1, 10));
//get spiral points per call
var p1x = cx + r*sin(theta);
var p1y = cy + r*cos(theta);
var p2x = cx - r*sin(theta);
var p2y = cy - r*cos(theta);
//draw grey strings
stroke(nColor);
line(0, 0, p1x, p1y); //top left to p1
line(canvasWidth, 0, p1x, p1y); //top right to p1
line(0, canvasHeight, p1x, p1y); //bottom left to p1
line(canvasWidth, canvasHeight, p1x, p1y); //bottom right to p1
line(0, 0, p2x, p2y); //top left to p2
line(canvasWidth, 0, p2x, p2y); //top right to p2
line(0, canvasHeight, p2x, p2y); //bottom left to p2
line(canvasWidth, canvasHeight, p2x, p2y); //bottom right to p2
//draw main strings;
stroke(hColor);
line(p1x, p1y, p2x, p2y); //through center (core of spiral)
//recursive call until base case is met
if (r > 1) {
drawSpiralArt(r*reductionFactor, theta + angleIncrement);
}
}
/////////////////////////////
// RUN!
/////////////////////////////
function setup() {
//init canvas vars
canvasWidth = 640;
canvasHeight = 480;
cx = canvasWidth/2;
cy = canvasHeight/2;
margin = 30;
//init color vars
bgColor = color(255); //white
nColor = color(136,136,136,35); //grey, semitransparent
hColor = color(255,0,136); //fucia
//init spiral vars
r0 = canvasWidth/2
theta0 = HALF_PI;
//init canvas
createCanvas(canvasWidth, canvasHeight);
}
function draw() {
background(bgColor);
//recalculate mouseX, mouseY with constraints
xm = constrain(mouseX, margin, canvasWidth-margin);
xy = constrain(mouseY, margin, canvasHeight-margin);
//draw spiral
drawSpiralArt(r0, theta0);
}
I wanted to use trigonometry to create a series of points predicated on circular geometry and then connect the points to various other points across the canvas. I ended up using recursion to generate a central spiral. I then used the mouse position to control the degree to which the length of strings in the spiral decimates (the rate at which the strings spiral towards the center) and to control the rotational rate of the strings in the spiral, so that spirals of various character could be created.
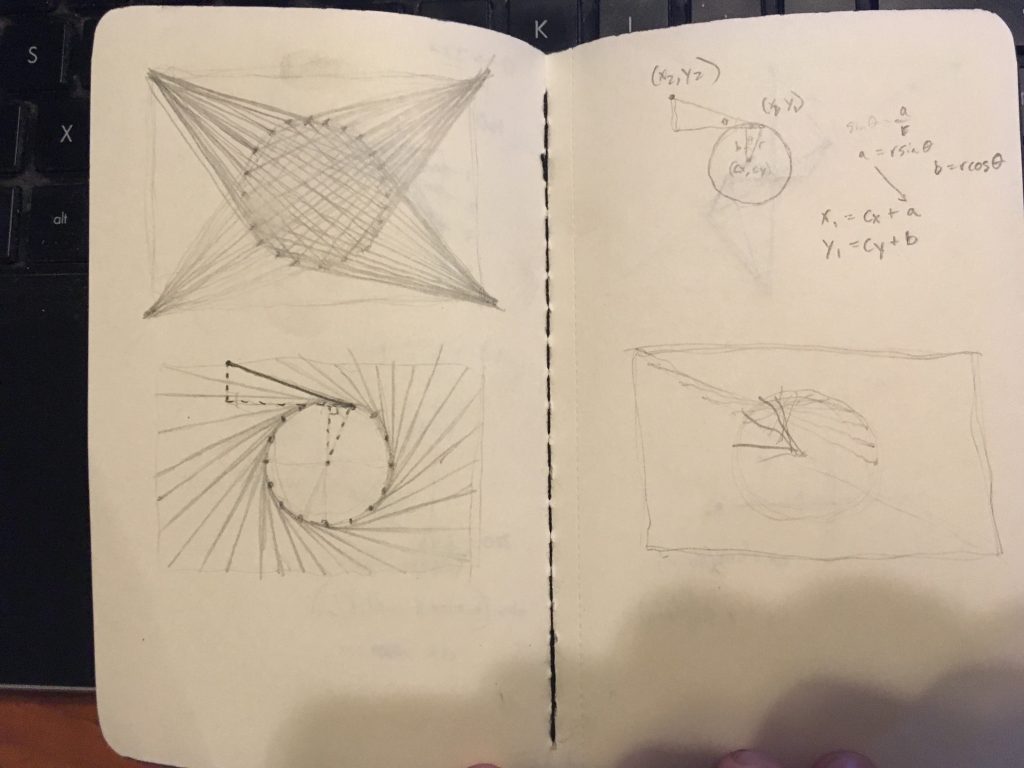
Later sketch focusing on spiral series generated from recursive rules.