For this project I’m interested in the dynamic shapes that the turtle graphic is able to produced. I selected a spiral base shape and users can click on the canvas to generate the geometry in different sizes.
//Shan Wang
//Section A
//shanw1@andrew.cmu.edu
//11-Project
function setup(){
createCanvas(600,600);
}
function mousePressed(){
alpha += 50;
//randomly select the number of rotates the basic geometry will go
var numRotate = random(30,60);
//randomly choose the initial strokeWeight for turtle
var strokeWei = random(0.2,1);
//base the size of the graph on the number of rotation
var size = map(numRotate,30,60,80,200);
//base the initial gradient of stroke on the position of x
var color = round(map(mouseX, 0, width, 150,200));
//create a turtle at the position where the mouse is pressed
var turtle1 = makeTurtle(mouseX,mouseY,color,strokeWei);
//set the decrement unit for color and stroke weight
var intervStro = turtle1.weight/numRotate;
var intervCol = turtle1.color/numRotate;
//generate the spiral
for (var counts = 0; counts < numRotate; counts++){
turtle1.penDown();
turtle1.color -= intervCol;
turtle1.weight -= intervStro;
//draw square
for (var j = 0; j<4; j++){
turtle1.forward(size-counts*2);
turtle1.right(90);
}
turtle1.left(10);
}
}
function turtleLeft(d) {
this.angle -= d;
}
function turtleRight(d) {
this.angle += d;
}
function turtleForward(p) {
var rad = radians(this.angle);
var newx = this.x + cos(rad) * p;
var newy = this.y + sin(rad) * p;
this.goto(newx, newy);
}
function turtleBack(p) {
this.forward(-p);
}
function turtlePenDown() {
this.penIsDown = true;
}
function turtlePenUp() {
this.penIsDown = false;
}
function turtleGoTo(x, y) {
if (this.penIsDown) {
stroke(this.color);
strokeWeight(this.weight);
line(this.x, this.y, x, y);
}
this.x = x;
this.y = y;
}
function turtleDistTo(x, y) {
return sqrt(sq(this.x - x) + sq(this.y - y));
}
function turtleAngleTo(x, y) {
var absAngle = degrees(atan2(y - this.y, x - this.x));
var angle = ((absAngle - this.angle) + 360) % 360.0;
return angle;
}
function turtleTurnToward(x, y, d) {
var angle = this.angleTo(x, y);
if (angle < 180) {
this.angle += d;
} else {
this.angle -= d;
}
}
function turtleSetColor(c) {
this.color = c;
}
function turtleSetWeight(w) {
this.weight = w;
}
function turtleFace(angle) {
this.angle = angle;
}
function makeTurtle(tx, ty, tColor,tStroke) {
var turtle = {x: tx, y: ty,
angle: 0.0,
penIsDown: true,
color: tColor,
weight: tStroke,
left: turtleLeft, right: turtleRight,
forward: turtleForward, back: turtleBack,
penDown: turtlePenDown, penUp: turtlePenUp,
goto: turtleGoTo, angleto: turtleAngleTo,
turnToward: turtleTurnToward,
distanceTo: turtleDistTo, angleTo: turtleAngleTo,
setColor: turtleSetColor, setWeight: turtleSetWeight,
face: turtleFace};
return turtle;
}
This is one of the image I created.
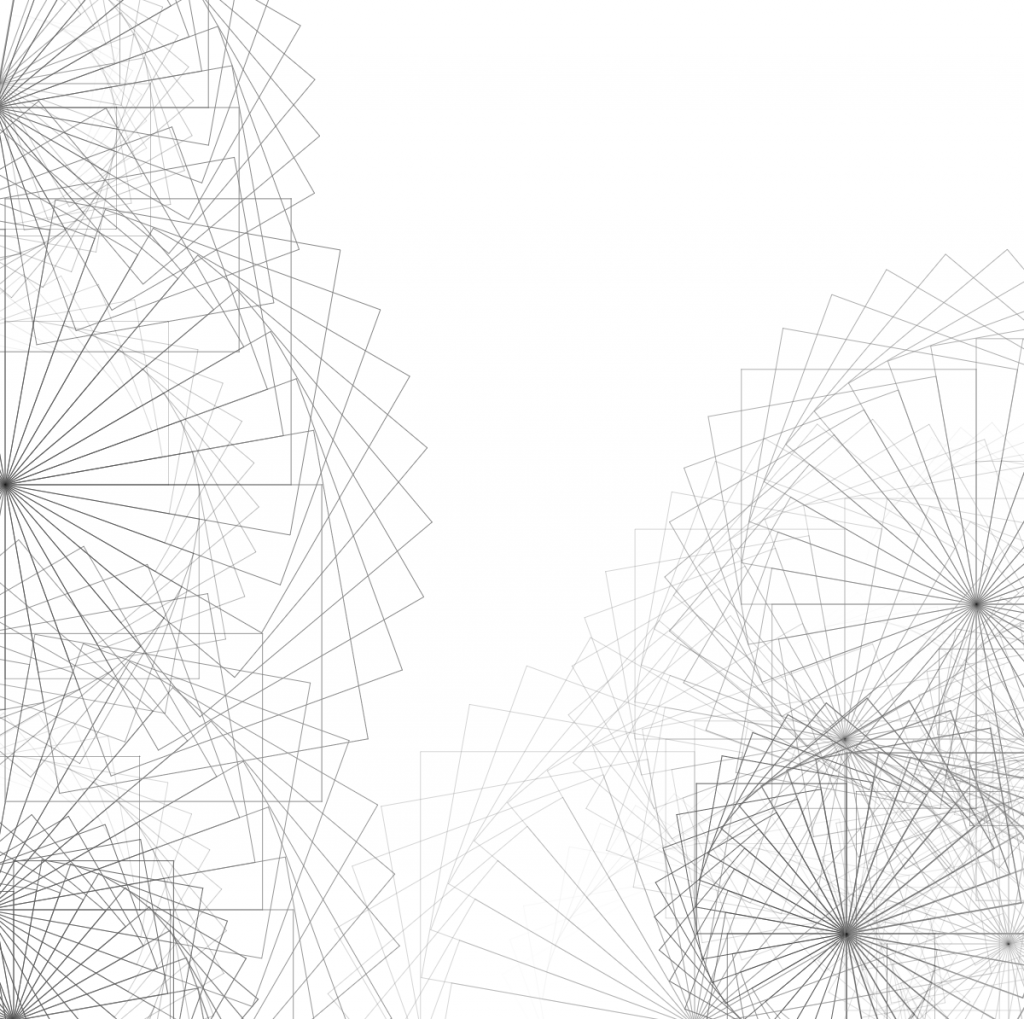