I liked this project a lot because it began to delve into more abstract realms. While the execution was difficult, I had a lot of fun in the process exploring various types of graphing functions and applications. In trying to determine how to build my proposed idea, I was able to rekindle a long held interest in computational art as well as explore the mathematical side of computing. I believe this project has a host of potential applications that will bode well for me in future projects and courses.
sketch
/* Jason Zhu
jlzhu@andrew.cmu.edu
Section E
Project 07-Curves
*/
function setup() {
createCanvas(480, 480);
}
function draw() {
// define constrain variables
var cx = constrain(mouseX, 0, 480);
var cy = constrain(mouseY, 0, 480);
// define color variables
var red = cx * .3
var green = cy * .3
var blue = 100
// define additional variables and draw shapes
background(red * .3 - 15, green * .3 - 15, blue * .3 - 15);
drawhypotrochoid();
drawhypotrochoid2();
drawflowerellipse();
drawflowerellipse2();
frameRate(999);
}
function drawhypotrochoid() {
push();
translate(width / 2, height / 2);
noFill();
// define constraint function
var cy = constrain(mouseY, 0, 480);
var cx = constrain(mouseX, 0, 480);
// define draw variables
var r1 = 360;
var a1;
var b1;
// define color variables
var red = cx * .25
var green = cy * .25
var blue = 100
// define stroke
strokeWeight(10);
stroke(red, green, blue);
// define shape parameters
// define alternation parameters
var a1 = map(cx, 0, width, 0, 25);
var b1 = map(cy, 0, height, 0, 1);
// define shape parameters
beginShape();
rotate(radians(angle));
for(var t = 0; t < 360; t += 2) {
var angle = map(t, 0, 360, 0, 360);
// equation
var x = (a1 - b1) * cos(angle) + r1 * cos((a1 - b1) * angle);
var y = (a1 - b1) * sin(angle) - r1 * sin((a1 - b1) * angle);
curveVertex(x, y);
}
endShape();
pop();
}
function drawflowerellipse(){
translate(width / 2, height / 2);
noFill();
// define constrain variables
var cx = constrain(mouseX, 0, 480);
var cy = constrain(mouseY, 0, 480);
// define draw variables
var angle = map(cx, 0, width, 0, 360);
var a2 = 100 + (.2 * cx);
var b2 = 100 + (.2 * cx);
// define color variables
var red = cx * .6
var green = cy * .6
var blue = 100
// define stroke
strokeWeight(1);
stroke(red, green, blue);
// define shape parameters
beginShape();
rotate(radians(angle));
for (var t = 0; t < 160; t +=2.8){
var x = a2 * (cos(t));
var y = b2 * (sin(t));
curveVertex(x,y);
}
endShape();
}
function drawflowerellipse2(){
noFill();
// define constrain variables
var cx = constrain(mouseX, 0, 480);
var cy = constrain(mouseY, 0, 480);
// define draw variables
var angle = map(cx, 0, width, 0, 360);
var a2 = 30 + (.2 * cx);
var b2 = 30 + (.2 * cx);
// define color variables
var red = cx * .6
var green = cy * .6
var blue = 100
// define stroke
strokeWeight(1);
stroke(red, green, blue);
// define shape parameters
beginShape();
rotate(radians(angle));
for (var t = 0; t < 160; t +=3.8){
var x = a2 * (cos(t));
var y = b2 * (sin(t));
curveVertex(x,y);
}
endShape();
}
function drawhypotrochoid2(){
push();
translate(width / 2, height / 2);
noFill();
// define constraint function
var cy = constrain(mouseY, 0, 480);
var cx = constrain(mouseX, 0, 480);
// define draw variables
var r1 = 360;
var a1;
var b1;
// define color variables
var red = cx * .3
var green = cy * .3
var blue = 100
// define stroke
strokeWeight(5);
stroke(red * .3 - 15, green * .3 - 15, blue * .3 - 15);
// define shape parameters
// define alternation parameters
var a1 = map(cx, 0, width, 0, 25);
var b1 = map(cy, 0, height, 0, 1);
// define shape parameters
beginShape();
rotate(radians(angle));
for(var t = 0; t < 360; t += 2) {
var angle = map(t, 0, 360, 0, 360);
// equation
var x = (a1 - b1) * cos(angle) + r1 * cos((a1 - b1) * angle);
var y = (a1 - b1) * sin(angle) - r1 * sin((a1 - b1) * angle);
curveVertex(x, y);
}
endShape();
pop();
}
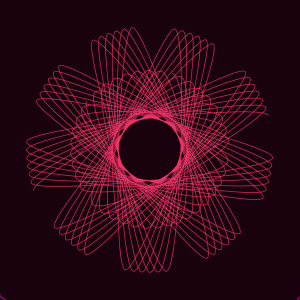
screenshot at the maximum width and minimum height of the canvas.
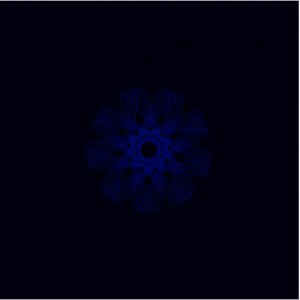
mouse X and mouse Y at the minimum width and height of the canvas.
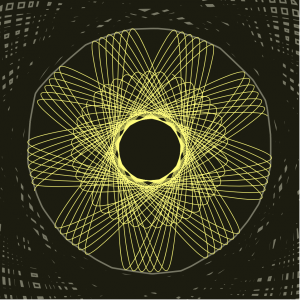
mouse X and mouse Y at the maximum width and height of the canvas.