/* Dani Delgado
Section E
ddelgad1@andrew.cmu.edu
Project-04
*/
function setup() {
createCanvas(400, 300);
background(10);
}
function draw() {
//draw the sixth layer of lines (brownish black lines)
//these lines are on the "inside" of all other line layers (its the lowest layer)
for(var g = 0; g < 1.5 ; g += 0.09) {
//set the stroke style
strokeWeight(0.2);
stroke(35, 19, 0);
//determine the lerp values
x1 = lerp(0, width / 2.15, g);
y1 = lerp(0, height * 2.15, g);
x2 = lerp(width * 2.15, 0, g);
y2 = lerp(height / 2.15, 0, g);
//draw the curves
line(x1, 0, width, y1);
line(x2, height, 0, y2);
line(x1, height, width, y2);
line(x2, 0, 0, y1);
}
//draw the fifth layer of lines (brown lines)
//these lines are on the "inside" the above layers of lines
for(var f = 0; f < 1.4 ; f += 0.08) {
//set the stroke style
strokeWeight(0.3);
stroke(51, 34, 0);
//determine the lerp values
x1 = lerp(0, width / 1.9, f);
y1 = lerp(0, height * 1.9, f);
x2 = lerp(width * 1.9, 0, f);
y2 = lerp(height / 1.9, 0, f);
//draw the curves
line(x1, 0, width, y1);
line(x2, height, 0, y2);
line(x1, height, width, y2);
line(x2, 0, 0, y1);
}
//draw the fourth layer of line (dark orange lines)
//these lines are "inside" the above layer of lines
for(var e = 0; e < 1.3; e += 0.07) {
//set the stroke style
strokeWeight(0.4);
stroke(102, 68, 0);
//determine the lerp values
x1 = lerp(0, width / 1.75, e);
y1 = lerp(0, height * 1.75, e);
x2 = lerp(width * 1.75, 0, e);
y2 = lerp(height / 1.75, 0, e);
//draw the curves
line(x1, 0, width, y1);
line(x2, height, 0, y2);
line(x1, height, width, y2);
line(x2, 0, 0, y1);
}
//draw the third layer of lines (medium orange lines)
//these lines are "inside" the above layer of lines
for(var d = 0; d < 1.2; d += 0.06) {
//set the stroke style
strokeWeight(0.6);
stroke(204, 136, 0);
//determine the lerp values
x1 = lerp(0, width / 1.5, d);
y1 = lerp(0, height * 1.5, d);
x2 = lerp(width * 1.5, 0, d);
y2 = lerp(height / 1.5, 0, d);
//draw the curves
line(x1, 0, width, y1);
line(x2, height, 0, y2);
line(x1, height, width, y2);
line(x2, 0, 0, y1);
}
//draw the second layer of lines (light orange lines)
//these lines are "inside" of the abvoe layer of lines
for(var c = 0; c < 1.1; c += 0.05) {
//set the stroke style
strokeWeight(0.8);
stroke(255, 204, 102);
//determine the lerp values
x1 = lerp(0, width / 1.5, c);
y1 = lerp(0, height, c);
x2 = lerp(width * 1.5, 0, c);
y2 = lerp(height, 0, c);
//draw the curves
line(x1, 0, width, y1);
line(x2, height, 0, y2);
line(x1, height, width, y2);
line(x2, 0, 0, y1);
}
//draw the uppermost line set (creme colored lines)
//the first line set will curve the corners
for(var i = 0; i < 1; i += 0.04) {
//set the stroke style
strokeWeight(1);
stroke(250, 240, 220);
//determine the lerp variables
x1 = lerp(0, width, i);
y1 = lerp(0, height, i);
x2 = lerp(width, 0, i);
y2 = lerp(height, 0, i);
//draw the curves
line(x1, 0, width, y1);
line(x2, height, 0, y2);
line(x1, height, width, y2);
line(x2, 0, 0, y1);
}
}
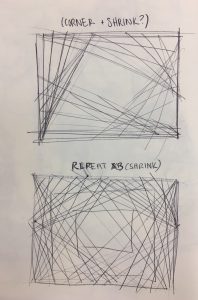
Since this is my first experience with for loops (outside of the other class assignments), I found this to be very challenging since I first had to solidify the conceptual aspect of the code before I could begin to work on the physical coding. Once I got into the groove of it though I had fun with the assignment!