/*
Alice Fang
acfang@andrew.cmu.edu
Section E
Project-06-AbstractClock
*/
function setup() {
createCanvas(400, 400);
noStroke();
}
function draw() {
background(146, 211, 231);
//current time
var H = hour();
var M = minute();
var S = second();
// sun
var arc = 140; // radius of sun trajectory path
var angle = H;
push();
translate(width/2, height/2);
rotate(radians(7.5*H)); // rotate based on time of day, with highest point at 12:00 pm
fill(239, 244, 214);
ellipse(0 - arc, 0, 36, 36);
pop();
//static landscape
fill(145, 168, 113); // farthest mountain
beginShape();
vertex(0, 166);
vertex(67, 186);
vertex(115, 175);
vertex(150, 182);
vertex(240, 152);
vertex(270, 160);
vertex(328, 144);
vertex(352, 153);
vertex(369, 150);
vertex(400, 157);
vertex(400, 400);
vertex(0, 400);
endShape(CLOSE);
fill(125, 147, 96); //third mountain
beginShape();
vertex(0, 226);
vertex(40, 208);
vertex(95, 224);
vertex(183, 205);
vertex(288, 218);
vertex(400, 186);
vertex(400, 400);
vertex(0, 400);
endShape(CLOSE);
fill(81, 110, 81, 80); // second mountain
beginShape();
vertex(0, 266);
vertex(20, 258);
vertex(40, 265);
vertex(110, 244);
vertex(200, 275);
vertex(260, 312);
vertex(400, 323);
vertex(400, 400);
vertex(0, 400);
endShape(CLOSE);
fill(81, 110, 81, 80); // closest mountain
beginShape();
vertex(0, 280);
vertex(47, 298);
vertex(77, 289);
vertex(119, 330);
vertex(175, 348);
vertex(211, 326);
vertex(288, 337);
vertex(325, 370);
vertex(400, 400);
vertex(0, 400);
endShape(CLOSE);
// hot air balloon!
var mapY1 = map(S, 0, 60, height, -175); // map y position of turquoise balloon to seconds
var mapY2 = map(M, 0, 60, height * 2, -175); // map y position of magenta balloon to minutes
var mapY3 = map(H, 0, 24, height * 2.857, -175); // map y position of orange balloon to hours
var balX = 300; // X position of balloon
var balY1 = mapY1;
var balY2 = mapY2;
var balY3 = mapY3;
drawBalloon(balX, balY1 + 60, 120, 1, 26, 120, 142); // turquoise balloon
drawBalloon(balX, balY2 + 60, 120, 0.5, 137, 62, 101); // magenta balloon
drawBalloon(balX - 100, balY3 + 60, 120, 0.35, 233, 153, 95); // orange balloon
if (H <= 11 & H >= 6) {
fill(252, 217, 192, 75) // morning color
rect(0, 0, width, height);
} else if (H >= 14 & H <= 20) {
fill(228, 77, 46, 75); // sunset color
rect(0, 0, width, height);
} else if (H > 20 || H < 6) {
fill(5, 6, 90, 99); // dusk color
rect(0, 0, width, height);
}
}
function drawBalloon(balX, balY, balSize, balScale, R, G, B) {
push();
scale(balScale);
fill(R, G, B);
ellipse(balX, balY, balSize, balSize); // balloon shape
quad(balX - 44, balY + 40, balX + 44, balY + 40, balX + 14, balY + 77, balX - 13, balY + 77);
stroke(0); // basket lines
line(balX - 12, balY + 77, balX - 12, balY + 90);
line(balX + 12, balY + 77, balX + 12, balY + 90);
noStroke();
fill(104, 81, 32); // basket
rect(balX - 12, balY + 90, 25, 25);
pop();
}
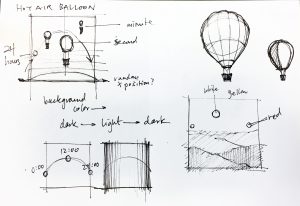
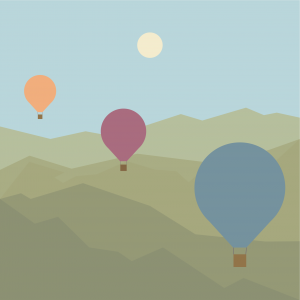
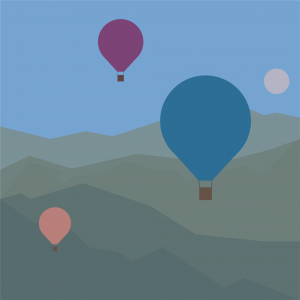
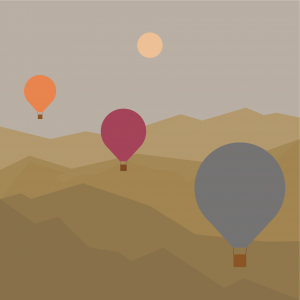
I was inspired by some photographs that I saw of hot air balloons, and I really wanted to play with the different colors of sky at different times of day. The trickiest parts for me were setting up the function drawBalloon() to create the balloons, because once I scaled them, I forgot what variables would be affected in terms of animation.
The largest, turquoise balloon represents seconds, with the magenta balloon as minutes and the orange balloon as hours. The sun also rises and sets based on the hour, with the highest point at noon.