// assignment 10 sample code referenced for trees
// source: https://courses.ideate.cmu.edu/15-104/f2018/week-10-due-nov-4/#landscape
// p5.js.org example referenced for snowflakes
// source: https://p5js.org/examples/simulate-snowflakes.html
var trees = [];
var snowflakes = [];
function setup() {
createCanvas(480, 200);
for (var i = 0; i < 10; i++){
var rx = random(width);
trees[i] = makeTree(rx);
}
frameRate(15);
}
function draw() {
background(80, 100, 150);
displayGround();
// trees
updateCanvas();
eraseOld();
addNew();
// snowflakes
let t = frameCount / 60;
for (var i = 0; i < random(5); i++) {
snowflakes.push(new snowflake());
}
for (let flake of snowflakes) {
flake.update(t);
flake.display();
}
}
// tree functions
function updateCanvas(){
for (var i = 0; i < trees.length; i++){
trees[i].move();
trees[i].display();
}
}
function eraseOld(){
var treesToKeep = [];
for (var i = 0; i < trees.length; i++){
if (trees[i].x + trees[i].width > 0) {
treesToKeep.push(trees[i]);
}
}
trees = treesToKeep;
}
function addNew() {
var newTreeLikelihood = 0.02;
if (random(0,1) < newTreeLikelihood) {
trees.push(makeTree(width));
}
}
function treeMove() {
this.x += this.speed;
}
function treeDisplay() {
var treeH = this.height;
fill(10, 60, 20);
noStroke();
push();
translate(this.x, height - 40);
triangle(0, treeH - 17, this.width, treeH - 17, this.width / 2, -treeH - 17);
pop();
}
function makeTree(birthLocationX) {
var tr = {x: birthLocationX,
width: random(10, 20),
speed: -1.0,
height: random(10, 20),
move: treeMove,
display: treeDisplay}
return tr;
}
function displayGround(){
fill(255);
noStroke();
rect(0, height - 50, width, 50);
// frame
stroke(200);
noFill();
rect(0, 0, width - 1, height - 1);
}
// snowflakes
function snowflake() {
this.posX = 0;
this.posY = random(-50, 0);
this.initialangle = random(0, 2 * PI);
this.size = random(1, 3);
this.radius = sqrt(random(pow(width / 2, 2)));
this.update = function(time) {
let w = 0.6;
let angle = w * time + this.initialangle;
this.posX = width / 2 + this.radius * sin(angle);
this.posY += pow(this.size, 0.5);
if (this.posY > height) {
let index = snowflakes.indexOf(this);
snowflakes.splice(index, 1);
}
}
this.display = function() {
fill(255);
noStroke();
ellipse(this.posX, this.posY, this.size);
}
}
It snowed in my hometown this past week so I was inspired to do a snowy winter landscape.
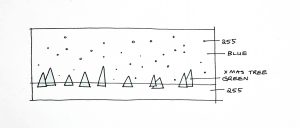
I started with a blue sky and white ground. Then, I referenced the buildings sample code to generate trees of different height and width triangles. Finally, I looked on the p5.js website to find an example showing how to make falling snowflakes (source: https://p5js.org/examples/simulate-snowflakes.html). I studied the code and adapted it to my landscape which required smaller snowflake particles.
I am really happy with the end result and it reminds me a lot of what Ottawa looks like in the winter. Also, I had some trouble understanding the lectures on particles last week so studying and learning from the snowflake example really helped.