/* Rachel Lee
Section E
rwlee@andrew.cmu.edu
Project 19: Generative Landscape
*/
var sailboats = [];
function setup() {
createCanvas(480, 480);
noStroke();
background('blue');
for(var i = 0; i < 3; i ++) {
var rndmX = random(width);
sailboats[i] = drawSailboats(rndmX);
}
frameRate(50);
}
function draw() {
// Background color changes according to hour time bracket
// Draw sun and moon according to hour time bracket
if (hour() >= 0 & hour() <= 8) {
fill(210, 195, 135);
rect(0, 0, width, height);
strokeWeight(15);
fill(240, 100, 70);
fill(225, 145, 90);
ellipse(390, 90, 135);
} else if (hour() > 8 & hour() <= 16) {
fill(190, 230, 250);
rect(0, 0, width, height);
stroke(255, 231, 101, 80);
strokeWeight(15);
fill(230, 210, 120);
ellipse(390, 90, 135);
} else if (hour() > 16 & hour() <= 24) {
fill(10, 50, 70);
rect(0, 0, width, height);
strokeWeight(15);
fill(205, 205, 200);
fill(225, 225, 210);
ellipse(390, 90, 135);
}
// Calls function to draw waves
waves1();
waves2();
updateandDisplaySailboats();
addSailboats();
}
// Draws wave layer 1 via Perlin Noise
function waves1() {
noStroke();
var wavesSpeed = 0.0002;
var wavesDetail = 0.0004;
push();
beginShape();
fill(0, 100, 140);
vertex(0, height);
for (var x = 0; x < width; x++) {
var w = (x * wavesDetail) + (millis() * wavesSpeed);
var y = map(noise(w), 0, 1.2, height /2 + 70, height);
vertex(x, y);
}
vertex(x, height);
vertex(0, height);
vertex(0, y);
endShape();
pop();
}
// Draws wave layer 2 via Perlin Noise
function waves2() {
noStroke();
wavesSpeed = 0.0004;
wavesDetail = 0.0008;
push();
beginShape();
fill(80, 135, 155);
vertex(0, height);
for (var x = 0; x < width; x++) {
var w = (x * wavesDetail) + (millis() * wavesSpeed);
var y = map(noise(w), 0, 1, height / 1.5 + 50, height);
vertex(x, y);
}
vertex(x, height);
vertex(0, height);
vertex(0, y);
endShape();
pop();
}
// Updates and displays the position of sailboats
function updateandDisplaySailboats() {
for(var i = 0; i < sailboats.length; i++) {
sailboats[i].move();
sailboats[i].display();
}
}
// Adds a new sailboat to the canvas according to a marginal probability
function addSailboats() {
var sailboatProbability = 0.008;
if (random(0, 1) < sailboatProbability) {
sailboats.push(drawSailboats(width));
}
}
// Updates the position of the sailboat with every frame
function moveSailboats() {
this.x += this.speed;
}
// Draws the sailboats
function displaySailboats() {
noStroke();
push();
translate(0, 220);
fill(190, 115, 75);
rect(width / 2 + this.x - 5, height / 2 - 100, 5, 100);
fill(230, 225, 225);
quad(width / 2 + this.x - 60, height / 2 - 30,
width / 2 + this.x + 50, height / 2 - 30,
width / 2 + this.x + 30, height / 2,
width / 2 + this.x - 40, height / 2);
fill(230, 85, 60);
triangle(width / 2 + this.x, height / 2 - 60,
width / 2 + this.x, height / 2 - 150,
width / 2 + this.x - 60, height / 2 - 40);
fill(225, 120, 110);
triangle(width / 2 + this.x, height / 2 - 60,
width / 2 + this.x, height / 2 - 150,
width / 2 + this.x + 40, height / 2 - 60);
pop();
}
function drawSailboats(birthLocationX, birthLocationY) {
var boat = {x: birthLocationX,
speed: -1,
r: random(0.1, 0.3),
move: moveSailboats,
display: displaySailboats,
}
return boat;
}
For this week’s assignment, I decided to depict some boats sailing across the water. When I was a kid, I would sit by the pier and watch junk boats and ferries glide across the harbour, so this scene really resonated with me. This project was a little challenging for me, but I challenged myself to experiment with Perlin noise, and create a landscape that changes over time (beyond the immediate shifting waves and number of boats). If I had more time, I would try to space the boats out a bit more, so that they don’t overlap, and also add clouds.
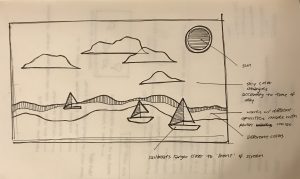