var sheep = [];
var terrainSpeed = 0.0005;
var terrainSpeedB = 0.0002;
var terrainSpeedS = 0.0001;
var terrainDetail = 0.02;
var terrainDetailB = 0.01;
var terrainDetailS = 0.03;
function setup() {
createCanvas(480, 480);
for (var i = 0; i < 10; i++){ //fills the array with sheep
var rx = random(width);
sheep[i] = makeSHEEPS(rx);
}
frameRate(10);
}
function draw() {
background("PaleTurquoise"); //the sky
noStroke();
fill("coral"); //sun
ellipse(130, 100, 30, 30)
//darkest green hills
beginShape();
stroke("darkgreen");
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeedS); //creates detail in terrain
//based on milliseconds that pass
var y = map(noise(t), 0,1, 170, height/2);
line(x, y, x, height); //I used this in order to fill my hills with color
}
endShape();
//darker green hills
beginShape();
stroke("forestgreen");
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeedB); //terrain speeds differ as object appear closer
//or further away
var y = map(noise(t), 0,1, 210, height/2);
line(x, y, x, height);
}
endShape();
//green hills
beginShape();
stroke("darkseagreen");
for (var x = 0; x < width; x++) {
var t = (x * terrainDetailB) + (millis() * terrainSpeedB);
var y = map(noise(t), 0,1, 280, height/2);
line(x, y, x, height);
}
endShape();
showSHEEP();
byeOLDsheep(); //removes old sheep when they leave the frame
addNewSHEEPS(); //adds more sheep
//road bushes
beginShape();
stroke("olivedrab");
for (var x = 0; x < width; x++) {
var t = (x * terrainDetailB) + (millis() * terrainSpeed);
var y = map(noise(t), 0,1, 430, 400);
line(x, y, x, height);
}
endShape();
noStroke();
displayROAD();
}
function showSHEEP() {
// prints and moves the sheep
for (var i = 0; i < sheep.length; i++){
sheep[i].move();
sheep[i].display();
}
}
function byeOLDsheep(){
var sheepToKeep = [];
for (var i = 0; i < sheep.length; i++){
if (sheep[i].x + sheep[i].breadth > 0) {
sheepToKeep.push(sheep[i]);
}
}
sheep = sheepToKeep; //fills with remaining sheeps so they continue to print
}
function addNewSHEEPS() {
var newSHEEPLikelihood = 0.009;
if (random(0,1) < newSHEEPLikelihood) {
sheep.push(makeSHEEPS(width));
}
}
//makes the sheep move forward with each frame, depending on var speed
function sheepMove() {
this.x += this.speed;
}
// the physical build of each sheep
function SheepDisplay() {
var floorHeight = 20;
var bHeight = this.nFloors * floorHeight;
push();
noStroke();
translate(this.x, height - 40);
//legs of sheep
fill("wheat");
rect(-7, -bHeight+3, 3, 16);
rect(-1, -bHeight+5, 3, 16);
rect(7, -bHeight+5, 3, 16);
rect(12, -bHeight+3, 3, 16);
//body of sheep
fill(255);
ellipse(0, -bHeight, 10, 10);
ellipse(10, -bHeight+10, 10, 10);
ellipse(5, -bHeight+10, 10, 10);
ellipse(10, -bHeight+5, 10, 10);
ellipse(10, -bHeight+5, 15, 15);
ellipse(-5, -bHeight+5, 15, 15);
ellipse(-5, -bHeight+10, 10, 10);
ellipse(0, -bHeight+12, 10, 10);
ellipse(10, -bHeight, 10, 10);
fill("wheat");
ellipse(-10, -bHeight+7, 12, 8);
ellipse(-7, -bHeight+3, 2, 5);
ellipse(-10, -bHeight+3, 2, 5);
fill("black");
ellipse(-10, -bHeight+7, 2, 2);
pop();
}
function makeSHEEPS(birthLocationX) { //creates sheeep at their randomized locations within reason
var lilsheep = {x: birthLocationX,
breadth: 10,
speed: -2.5,
nFloors: round(random(2,8)),
move: sheepMove,
display: SheepDisplay}
return lilsheep;
}
function displayROAD(){ //function for printing the road in the foreground
noStroke();
fill("silver");
rect(0,height-50, width, height-50);
fill("dimgray");
rect(0,height-45, width, height-50);
}
For this landscape, I wanted to make something that was idellic and nice to look at. When I was little, I would always try to draw the landscape outside when I was sitting in the car on road trips (especially when there are livestock to admire!) but I never could capture it accurately since it’s moving by so quickly. This is hardly an accurate description of what I would remember, but I like that this medium allows me to create moving animations that at least capture that aspect of my memory. I also had fun choosing colors.
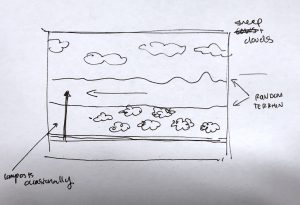