/*
Emma Nicklas-Morris
Section B
enicklas
Project-11
Generative Landscape
*/
var waterSpeed = 0.0002;
var waterDetail = 0.002;
var c2, c5; // gradient colors
var yPos = []; // water y position
var tubers = [];
var r1 = 50;
function setup() {
createCanvas(450, 300);
frameRate(10);
// colors for water gradient
c2 = color("#8cbaba");
c5 = color("#005364");
}
function draw() {
setGradient(c2, c5);
// draw water
water();
// the sun
noStroke();
fill("#FFE484");
ellipse(width - r1, r1, r1+10, r1+10);
fill("#FFCC33");
ellipse(width - r1, r1, r1, r1);
updateAndDisplayTuber();
removeTubers();
addTuber();
}
function water() {
noFill();
beginShape();
stroke("skyblue");
// draw the water that varies in height
for (var x = 0; x < width; x++) {
var t = (x * waterDetail) + (millis() * waterSpeed);
var y = map(noise(t), 0,1, height/2, 3/4*height);
line(x, y, x, -300); // illusion that the bottom water is changing height
// only keep y positions that are on the screen
if (yPos.length > width) {
yPos.shift();
}
yPos.push(y);
}
endShape();
}
function addTuber() {
var probability = 0.08;
// randomly make a new tuber as long as there are no more than
// 5 tubers on screen at once
if ((random(0,1) < probability) & (tubers.length < 5)){
var yT = yPos[width];
var t = makeTuber(width, yT - 15);
// set the color of the tube
var r = random(255);
var g = random(255);
var b = random(255);
var c = color(r, g, b);
t.setColor(c);
tubers.push(t); // add tuber into list
}
}
function removeTubers() {
for (var j = 0; j < tubers.length; j++) {
// if the tuber goes off the screen, remove from list
if (tubers[j].x + 50 < 0) {
tubers.splice(j, 1);
}
}
}
function makeTuber(x1, y1) {
var tuber = {x: x1, y: y1, hSize: 17, bWidth: 10, bH: 25,
color: color(128), move: moveTuber,
drawT: drawTuber, setColor: setTubeColor
}
return tuber;
}
// draw and update position of tuber
function updateAndDisplayTuber() {
for (var i = 0; i < tubers.length; i++) {
tubers[i].drawT();
tubers[i].move();
}
}
function moveTuber() {
this.x -= 12.0;
}
function drawTuber() {
noStroke();
fill(0);
// head
ellipse(this.x, this.y, this.hSize, this.hSize);
// torso
push();
translate(this.x + this.bWidth, this.y + this.bWidth);
rotate(radians(30));
ellipse(0, 1, this.bH + 5, this.bWidth);
pop();
// thighs (legs)
push();
translate(this.x + 3 * this.bWidth, this.y + this.bWidth);
rotate(radians(-20));
ellipse(0, 5, this.bH, this.bWidth);
pop();
// calves (legs)
push();
translate(this.x + 2 * this.bH, this.y + 2 * this.bWidth);
rotate(radians(40));
ellipse(0, 0, this.bH, this.bWidth);
pop();
// tube
fill(this.color);
ellipse(this.x + 20, this.y + 20, this.bH * 2, 1.5 * this.bWidth);
}
function setTubeColor(c) {
this.color = c;
}
// color gradient for the water
function setGradient(c1, c2) {
noFill();
for (var z = height/2; z < height; z++) {
var inter = map(z, height/2, height, 0, 1);
var c = lerpColor(c1, c2, inter);
stroke(c);
line(0, z, width, z);
}
}
I struggled a lot to get my object of Tubers to work and move with my water. There were a lot of “moving” parts to figure out and I had trouble when I tried to add lots of different elements at once. So once I slowed down and tried to get small parts, one at a time, I began to make more progress. I really like how my water looks. It was fun to experiment with gradients this project. Enjoy tubing 🙂
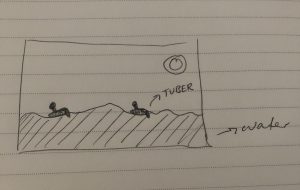