/* Chelsea Fan
Section 1B
chelseaf@andrew.cmu.edu
Project-07
*/
function setup() {
createCanvas(480, 480);
}
function draw() {
background(mouseX/2, width/2, 255);
drawHypocycloid1(); //draw hypocloid 1
drawHypocycloid2(); //draw hypocycloid 2
}
function drawHypocycloid1() {
//constrain mouse values to only affect when on canvas
var x = constrain(mouseX, 0, width);
var y = constrain(mouseY, 0, width);
noFill(); //no fill color
strokeWeight(2); //line thickness
stroke(width-mouseX); //stoke color changes based on mouseX
//Hypocycloid 1
push();
translate(width/2, height/2); //begin at center of canvas
var a = map(mouseX, 0, width, 0, 100);
var b = map(mouseY, 0, height, 0, 100);
beginShape();
for (var i = 0; i<=100; i++) {
var t = map(i, 0, 100, 0, TWO_PI); //angle variable
//hypocycloid1 equations
x = (a+b)*cos(t) + b*cos((a+b)*t);
y = (a+b)*sin(t) - b*sin((a+b)*t);
vertex(x, y);
}
endShape();
pop()
}
function drawHypocycloid2() {
//Hypocycloid 2
push();
translate(width/2, height/2); //begin at center of canvas
var a = map(width-mouseX, 0, width, 0, 100);
var b = map(width-mouseY, 0, height, 0, 100);
beginShape();
for (var i = 0; i<=150; i++) {
var t = map(i, 0, 100, 0, 360); //angle variable
//hypocycloid2 equations
x = (a+b)*cos(t) + b*cos((a+b)*t);
y = (a+b)*sin(t) - b*sin((a+b)*t);
vertex(x, y);
}
endShape();
pop()
}
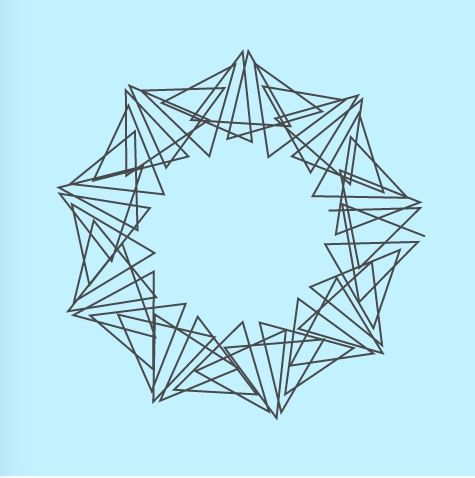
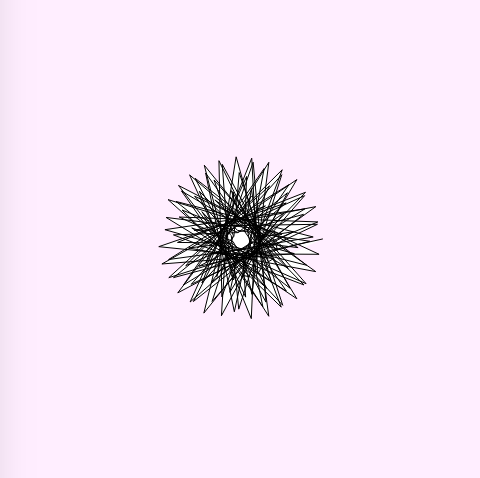
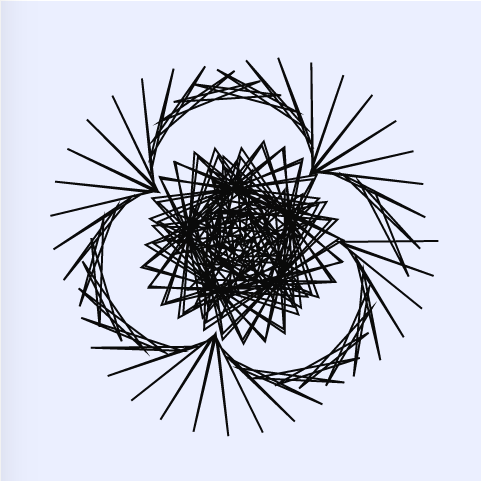
It took me quite a while to figure out how to use an equation of a hypocycloid in javascript. In addition, I spent a lot of time looking through the MathWorld website looking at different curves and equations. I really enjoyed looking at the curves. I like the colors that I chose. The background is blue when the mouse is on the left and changes to pink as the mouse moves right. In addition, I like that the color of the hypocycloid drawings change from black to white and contrasts the background color. I am pretty happy with my product. However, I prefer being able to imagine (in my mind) what my code will create/draw. And, I can imagine what one individual hypocycloid looks like on the screen, but I could never imagine all the changes of my drawing based on where the mouse is.