function setup () {
createCanvas(600, 600);
headW = random(250, 450);
headH = random(300, 450);
noseH = random(60, 150);
noseW = random(50, 110);
eyeLX = 300 - (headW / 6);
eyeRX = 300 + (headW / 6);
eyeY = 300 - (headH / 5);
cheekLX = 300 - (headW / 4);
cheekRX = 300 + (headW / 4);
cheekY = 300 + (headW / 5);
r = random(40, 255);
g = random(40, 255);
b = random(40, 255);
}
function draw() {
background(255 - r, 255 - g, 255 - b);
fill(r, g, b);
noStroke(0);
ellipse(300, 300, headW, headH);
ellipse(cheekLX, cheekY, headW / 2, headH / 2);
ellipse(cheekRX, cheekY, headW / 2, headH / 2);
fill(240);
ellipse(eyeLX, eyeY, headW / 5, headH / 5);
ellipse(eyeRX, eyeY, headW / 5, headH / 5);
fill(230 - r, 230 - b, 230 - g);
ellipse(eyeLX + (headW / 40), eyeY + (headH / 40), 30, 30);
ellipse(eyeRX - (headW / 40), eyeY + (headH / 40), 30, 30);
fill(r - 100, g - 100, b - 100);
bezier(cheekLX, cheekY, cheekLX + headW / 4, cheekY + headH / 4, cheekRX - headW / 4, cheekY + headH / 4, cheekRX, cheekY);
fill(r - 30, g - 30, b - 30);
ellipse(300, 300, noseW, noseH);
ellipse(300 - (noseW / 2), 300 + (noseH / 6), noseH / 3, noseH / 3);
ellipse(300 + (noseW / 2), 300 + (noseH / 6), noseH / 3, noseH / 3);
}
function mousePressed() {
headW = random(250, 450);
headH = random(300, 450);
noseH = random(60, 150);
noseW = random(50, 110);
r = random(40, 255);
g = random(40, 255);
b = random(40, 255);
eyeLX = 300 - (headW / 6);
eyeRX = 300 + (headW / 6);
eyeY = 300 - (headH / 5);
cheekLX = 300 - (headW / 4);
cheekRX = 300 + (headW / 4);
cheekY = 300 + (headW / 5);
}
Category: Uncategorized
Claire Yoon-Project-02-Variable-Face
/*Claire Yoon
Section E
claireyo@andrew.cmu.edu
Assignment-02
*/
// variables for face.
var eyeSize = 35;
var pupilSize = 25;
var faceWidth = 110;
var faceHeight = 110;
var facecolorR = 255
var facecolorG= 204;
var facecolorB= 77;
var brow = 185;
var mouthx= 60
var mouthy= 40
function setup() {
createCanvas(640, 480);
}
function draw() {
background(249);
noStroke (0)
//face
fill(250, facecolorG, facecolorB)
ellipse(width / 2, height / 2, faceWidth*2, faceHeight*2);
//outer eye
fill("white")
var eyeLX = width / 2 - faceWidth * 0.40;
var eyeRX = width / 2 + faceWidth * 0.40;
ellipse(eyeLX, height / 2.05, eyeSize, eyeSize);
ellipse(eyeRX, height / 2.05, eyeSize, eyeSize);
//pupil
fill(103, 70, 0)
var pupilLX = width / 2 - faceWidth * 0.40;
var pupilRX = width / 2 + faceWidth * 0.40;
ellipse(pupilLX, height / 2.05, pupilSize, pupilSize);
ellipse(pupilRX, height / 2.05, pupilSize, pupilSize);
//blush
fill(238, 134, 154)
var eyeLX = width / 2 - faceWidth*0.7;
var eyeRX = width / 2 + faceWidth*0.7;
ellipse(eyeLX, height / 1.8, eyeSize, eyeSize);
ellipse(eyeRX, height / 1.8, eyeSize, eyeSize);
//eyebrow
stroke(103, 70, 0);
strokeWeight(9);
noFill();
beginShape();
curveVertex(250, 200);
curveVertex(250, 200);
curveVertex(270, brow);
curveVertex(300, brow);
curveVertex(300, brow);
endShape();
stroke(103, 70, 0);
strokeWeight(9);
noFill();
beginShape();
curveVertex(340, brow);
curveVertex(340, brow);
curveVertex(370, brow);
curveVertex(390, 200);
curveVertex(390, 200);
endShape();
//mouth
stroke(103, 70, 0);
strokeWeight(9);
noFill();
arc(width / 2, height / 1.8, mouthx, mouthy, TWO_PI, PI);
}
function mousePressed() {
// when the user clicks, these variables are reassigned
// to random values within specified ranges. For example,
// 'faceWidth' gets a random value between 75 and 150.
faceWidth = random(100, 130);
faceHeight = random(100, 130);
facecolorG = random(76, 250);
facecolorB = random(36, 120);
eyeSize = random(0,50)
pupilSize = random(20, 30);
brow = random(180,200)
mouthx = random(40,80)
mouthy= random(30,70)
}
I gained inspiration from the emoji and set some values for things such as the eyebrows, eyes and the color of the face to show the difference in emotions when clicking on it.
Shannon Ha – Project 02 – Variable Face
var bunW = 106;
var bunH = 88;
var bangsShowH = 166;
var bangsShowW = 305;
var bangsHideH = 83;
var bangsHideW = 305;
var faceWidth = 329;
var faceHeight = 267;
var eyesW = 44;
var eyesH = 31;
var pupilsA = 12;
var pupilsB = 12;
var pupilsXL = 235;
var pupilsY = 259;
var pupilsXR = pupilsXL + 140;
var mouthW = 74;
var mouthH = 21;
var mouthX = 336;
var mouthY = 341;
var backgroundR = 102;
var backgroundG = 45;
var backgroundB = 145;
var haircolor = 0;
function setup() {
createCanvas(640, 480);
noStroke();
}
function draw() {
//background
background(backgroundR,backgroundG,backgroundB);
//face
fill(240,199,156);
noStroke();
ellipse(320 , 267, faceWidth, faceHeight);
//bun hair
fill(haircolor);
noStroke();
ellipse(208,143,bunW,bunH);
ellipse(431,143,bunW,bunH);
//bangs hair
fill(haircolor);
noStroke();
ellipse(320,192,bangsShowW,bangsShowH);
rectMode(RADIUS);
noStroke();
fill(240,199,156);
rect(321,252,bangsHideW/2,bangsHideH/2);
//hair pins
stroke(238,28,72);
strokeWeight(3);
line(172,175,254,120);
line(386,120,467,175);
//eyes
fill(255);
noStroke();
ellipse(243,256,eyesW,eyesH);
ellipse(381,256,eyesW,eyesH);
//pupils
fill(0);
ellipse(pupilsXL,pupilsY,pupilsA,pupilsB);
ellipse(pupilsXR,pupilsY,pupilsA,pupilsB);
//nose
fill(170,134,77);
ellipse(304,291,30,21);
fill(240,199,156);
ellipse(305,285,30,21);
//mouth
fill(0);
ellipse(mouthX,mouthY,mouthW,mouthH);
}
function mousePressed() {
faceWidth = random(245,348);
faceHeight = random(272,309);
bangsHideW = random(236,250);
bangsHideH = random(50,100);
haircolor = random(0,255);
eyesW = random(44,60);
eyesH = random(31,52);
pupilsXL = random(229,260);
pupilsY = random(248,267);
pupilsA = random(11,29);
pupilsB = random(11,30);
mouthX = random(284,378);
mouthY = random(338,364);
mouthW = random(33,92);
mouthH = random(15,63);
backgroundR = random(0,225);
backgroundG = random(0,225);
backgroundB = random(0,225);
}
For this project, I explored how to make a variety of expressions using random variables. At first it was a challenge to figure out the relationships between each variable, but it got easier after I made several errors.
Sean Leo-Project 02-Variable Faces
//Sean B. Leo
//sleo@andrew.cmu.edu
//Section C
//Project_02
var eyeSize = 20;
var pupil = 10;
var faceWidth = 100;
var faceHeight = 150;
var red = ("red");
var green = ("green");
var blue = ("blue");
var r1 = 255;
var g1 = 255;
var b1 = 255;
var r2 = 255;
var g2 = 255;
var b2 = 255;
var A = 20;
var B = 40;
var C = 1;
function setup() {
createCanvas(600, 500);
}
function draw() {
background(red, green, blue);
let c = color(255, 255, 255);
//body
fill(r2, g2, b2);
ellipse(width/2, height/2 + faceHeight, faceWidth + (faceWidth/2), faceHeight + (faceHeight/2));
//ellipse((width / 2) + faceWidth, (height / 2) + faceHieght, faceWidth * 2, faceHeight * 2);
//head
fill(r1, g1, b1),
ellipse(width / 2, height / 2, faceWidth, faceHeight);
//eyes
fill(255);
var eyeHeightLX = (height/2);
var eyeHeightRX = (height/2);
var eyeLX = width / 2 - faceWidth * 0.25;
var eyeRX = width / 2 + faceWidth * 0.25;
ellipse(eyeLX, height / 2, eyeSize, eyeSize);
ellipse(eyeRX, height / 2, eyeSize, eyeSize);
//pupils
fill(0);
ellipse(eyeLX, eyeHeightLX, pupil, pupil);
ellipse(eyeRX, eyeHeightRX, pupil, pupil);
//eyebrows
{strokeWeight(3);
var eyeBrowLH1 = eyeHeightLX-pupil*2;
var eyeBrowLH2 = eyeHeightLX-pupil*C;
var eyeBrowRH1 = eyeHeightRX-pupil*C;
var eyeBrowRH2 = eyeHeightRX-pupil*2;
line(eyeLX-pupil, eyeBrowLH1, eyeLX+pupil, eyeBrowLH2);
line(eyeRX-pupil, eyeBrowRH1, eyeRX+pupil, eyeBrowRH2);
}
//mouth
fill(0);
var mouthHeight=height/2 + faceHeight *.30;
ellipse(width/2, mouthHeight, B, 20);
//nose
fill(r1, g1, b1);
arc(width/2, height/2 + faceHeight*.09, A, A, C, PI + QUARTER_PI, OPEN);
}
function mousePressed() {
red = random(0,255);
green = random(0, 255);
blue = random(0, 255);
r1 = random(20, 255);
g1 = random(20, 255);
b1 = random(20, 255);
r2 = random(0, 255);
g2 = random(0, 255);
b2 = random(0, 255);
A = random(1,30);
B = random(1,40);
C = random(1,3);
faceWidth = random(75, 250);
faceHeight = random(75, 250);
eyeSize = random(10, 30);
pupil = random(7, 12);
}
A big part of figuring out this project was trying to wrap my head around what was spatially dependent on what. It was easier to think of things modularly; if the eyebrow is linked to the eye and if the eye is linked to the head, if the head changes everything else will follow.
Alice Cai Project 02
var eyeSize = 50;
var faceWidth = 250;
var faceHeight = 300;
var mouthWidth = 100
var mouthHeight = 50
var flufF = 50
function setup() {
createCanvas(640,480);
}
function draw() {
background(252, 136, 3);
//body
fill(234, 255, 0);
strokeWeight(5);
ellipse(width / 2, height, faceWidth, faceHeight);
//face
fill(234, 255, 0);
strokeWeight(5);
ellipse(width / 2, height / 2, faceWidth, faceHeight);
//eyes
fill(255);
stroke(0);
strokeWeight(0);
var eyeLX = width / 2 - faceWidth * 0.2;
var eyeRX = width / 2 + faceWidth * 0.2;
ellipse(eyeLX + 10, height / 2, eyeSize + 10, eyeSize);
ellipse(eyeRX + 10 , height / 2, eyeSize + 10, eyeSize);
fill(0);
stroke(0);
strokeWeight(0);
var eyeLX = width / 2 - faceWidth * 0.2;
var eyeRX = width / 2 + faceWidth * 0.2;
ellipse(eyeLX, height / 2, eyeSize, eyeSize);
ellipse(eyeRX, height / 2, eyeSize, eyeSize);
//mouth
fill(194, 66, 66);
strokeWeight(0);
ellipse(width / 2, height / 1.5, mouthWidth, mouthHeight);
//fluff
stroke(234, 255, 0);
strokeWeight(30);
line(width / 2.05, 80, width /2.1 , 120);
stroke(234, 255, 0);
strokeWeight(20);
line(width / 2.5, 80, width /2.1 , 120);
stroke(234, 255, 0);
strokeWeight(10);
line(width / 2.3, 50, width /2.1 , 120);
}
function mousePressed() {
// when the user clicks, these variables are reassigned
// to random values within specified ranges. For example,
// 'faceWidth' gets a random value between 75 and 150.
faceWidth = random(200, 250);
faceHeight = random(250, 350);
eyeSize = random(30, 60);
mouthHeight = random(40, 60);
mouthWidth = random(75, 100);
}
This is my chick! I first made variables for the values that I wanted to change. Then made a canvas and shapes. I used canvas width/height variable to control a lot of shape positioning. Finally, I set some values to random when click, and also set restraints so shapes don’t come out of the face.
Sarah Kang – Looking Outwards – 02
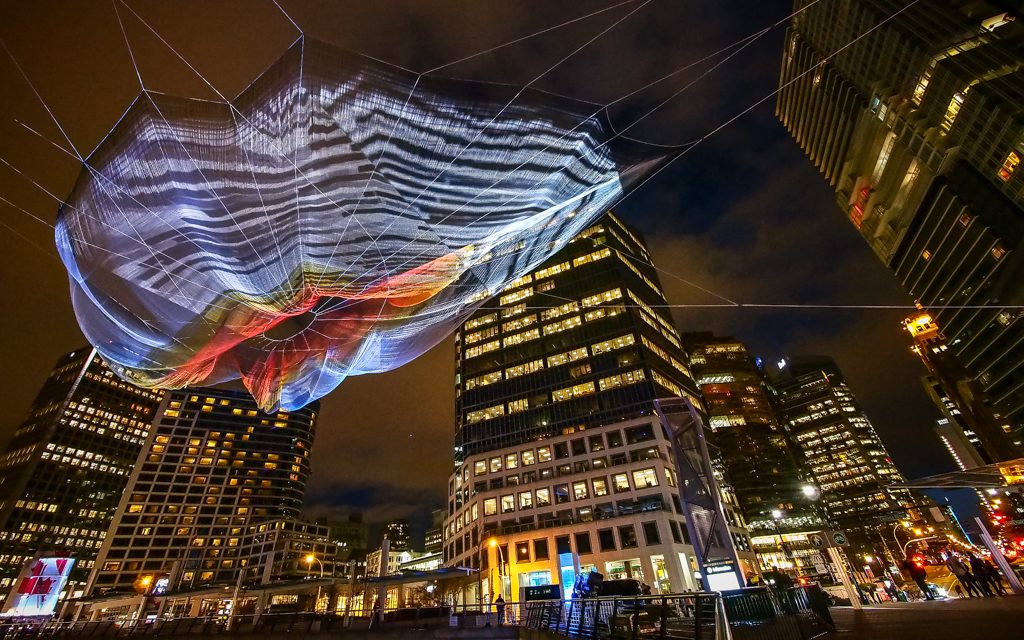
Unnumbered Sparks was an interactive installation project created through the collaboration generative artist Aaron Koblin and sculptor, Janet Echelman. This giant, floating canvas was installed in downtown Vancouver, Canada in March of 2014, generating through the real-time data sent through visitors’ mobile devices. I was first interested in this project by its striking visual quality, but then even more amazed by the rendering aspect of this artwork, as visitors directly painted magnified beams of colored light with just small movements on their phones. This project was entirely manifested on a giant, Google Chrome window and programmed using Go, a language that manages the visitor interactions and outputs the visuals to the light projectors. WebGL is a web technology that enables real-time graphics processing; with the WebSockets connection, when users make contact with their phones, the location data is transmitted to the server, allowing instant interaction with the giant canvas projections.
The Making of Unnumbered Sparks, from YouTube.
Aaron Koblin’s focus on data-based interactive digital art partnered with Janet Echelman’s beautiful, flowing sculptures creates a visual experience that users can directly experience and become a part of.
Shariq Shah – Looking Outwards- 02
Michael Hansmeyer is a computational designer interested specifically in the architectural implications of computational systems that generate a variety of complex design possibilities. Some of his latest works include a two full scale 3D printed sandstone grottoes and an iron and lace gazebo at the Gwangju Design Biennale. Hansmeyer employs a computational approach that emphasizes a level of hyper resolution in architectural spaces that can only be achieved rigorously with a computational and generative system. Because of this, algorithmic frameworks allow the specificity and complexity of surface conditions, ornament patterns, and material expression to take on a life of its own and characterize entirely new spatial conditions. As an architecture student, this work is fascinating as the role of architect is brought into question. This type of work calls for a paradigm shift from the view of the architect as the omniscient designer to one where the architect sets up a system and chooses from the results.
Shariq Shah – Project 02 – Variable Faces
// Shariq M. Shah
// Section C
// shariqs@andrew.cmu.edu
// Assignment_01
//setting up variables
var eyeWidth = 20
var eyeHeight = 20
var eyePosL = 240
var eyePosR = 340
var mouthC = 80
var backColor = 200
var earPos = 280
var nosePos = 320
var faceWidth = 220
function setup() {
createCanvas(480, 640);
}
function draw() {
background(230, backColor, 211);
noStroke()
fill(250, 200, 0)
ellipse(300,600,600,300)
strokeWeight(2)
rect(220, 400, 150, 150, 15)
//Face
fill(200, 162, 132)
rect(170, 180, 250, 250, 30, 100, 150, 150)
fill(0,0,0)
ellipse(300,180, faceWidth, 150)
rect(150, 180, 300, 80,30)
//Left Spec
noFill()
stroke(300)
ellipse(240, 290, 60, 60)
ellipse(240, 290, 55, 55)
//Left Eye
stroke(300)
fill(300)
ellipse(240, 290, eyeWidth, eyeHeight)
fill(0)
ellipse(eyePosL, 290, 15, 20)
ellipse(eyePosL, 285, 2, 2)
//Right Eye
stroke(300)
fill(300)
ellipse(340, 290, eyeWidth, eyeHeight)
fill(0)
ellipse(eyePosR, 290, 15, 20)
ellipse(eyePosR, 285, 2, 2)
line(175, 180, 425, 180)
//Right Spec
noFill()
stroke(300)
ellipse(340, 290, 60, 60)
ellipse(340, 290, 55, 55)
line(310,290, 270, 290)
line(310,285, 270, 285)
line(210,285, 170, 280)
line(210,290, 170, 280)
line(370,285, 420, 280)
line(370,290, 420, 280)
//Nose
noStroke()
fill(240, 171, 142)
triangle(290, 300, 290, nosePos, 310, 360)
fill(214, 171, 139)
triangle(290, 300, 290, nosePos, 270, 360)
//Mouth
fill (300);
ellipse (290, 382, mouthC, 42);
fill (200, 162, 132);
rect (220, 360, 120, 21);
//Ears
ellipse(425,earPos, 20, 80)
ellipse(165,earPos, 20, 80)
fill(300-backColor)
//Logo
bezier(105, 20, 10, 10, 90, 90, 15, 80)
//Collar
bezier(220, 460, 300, 650, 150, 650, 370, 460)
}
function mousePressed() {
eyeWidth = random(15, 50);
eyeHeight = random(20, 35);
eyePosL = random(240, 250);
eyePosR = random(340, 350);
mouthC = random(30, 90);
backColor = random(100, 200);
earPos = random(300,310)
nosePos = random(320, 360)
faceWidth = random(220, 250)
}
In this exercise, I explored multiple ways to vary the way different shapes and colors that change as a result of the mousePressed() function. The result is a strange, expressive, and colorful face that alters itself randomly, with constraints, as a result of pressing on the image.
Shariq Shah – Project – 02 – Variable Faces
// Shariq M. Shah
// Section C
// shariqs@andrew.cmu.edu
// Assignment_01
//setting up variables
var eyeWidth = 20
var eyeHeight = 20
var eyePosL = 240
var eyePosR = 340
var mouthC = 80
var backColor = 200
var earPos = 280
var nosePos = 320
function setup() {
createCanvas(480, 640);
}
function draw() {
background(230, backColor, 211);
noStroke()
fill(250, 200, 0)
ellipse(300,600,600,300)
strokeWeight(2)
rect(220, 400, 150, 150, 15)
//Face
fill(200, 162, 132)
rect(170, 180, 250, 250, 30, 100, 150, 150)
fill(0,0,0)
ellipse(300,180, 250, 150)
rect(150, 180, 300, 80,30)
//Left Spec
noFill()
stroke(300)
ellipse(240, 290, 60, 60)
ellipse(240, 290, 55, 55)
//Left Eye
stroke(300)
fill(300)
ellipse(240, 290, eyeWidth, eyeHeight)
fill(0)
ellipse(eyePosL, 290, 15, 20)
ellipse(eyePosL, 285, 2, 2)
//Right Eye
stroke(300)
fill(300)
ellipse(340, 290, eyeWidth, eyeHeight)
fill(0)
ellipse(eyePosR, 290, 15, 20)
ellipse(eyePosR, 285, 2, 2)
line(175, 180, 425, 180)
//Right Spec
noFill()
stroke(300)
ellipse(340, 290, 60, 60)
ellipse(340, 290, 55, 55)
line(310,290, 270, 290)
line(310,285, 270, 285)
line(210,285, 170, 280)
line(210,290, 170, 280)
line(370,285, 420, 280)
line(370,290, 420, 280)
//Nose
noStroke()
fill(240, 171, 142)
triangle(290, 300, 290, nosePos, 310, 360)
fill(214, 171, 139)
triangle(290, 300, 290, nosePos, 270, 360)
//Mouth
fill (300);
ellipse (290, 382, mouthC, 42);
fill (200, 162, 132);
rect (220, 360, 120, 21);
//Ears
ellipse(425,earPos, 20, 80)
ellipse(165,earPos, 20, 80)
fill(300)
//Logo
bezier(105, 20, 10, 10, 90, 90, 15, 80)
//Collar
bezier(220, 460, 300, 650, 150, 650, 370, 460)
}
function mousePressed() {
eyeWidth = random(15, 50);
eyeHeight = random(20, 35);
eyePosL = random(240, 250);
eyePosR = random(340, 350);
mouthC = random(30, 90);
backColor = random(100, 200);
earPos = random(300,310)
nosePos = random(320, 360)
}
Yoshi Torralva-Project-02-Variable-Face
//Yoshi Torralva
//Section E
//yrt@andrew.cmu.edu
//Project 02 Variable Face
var eyeSize = 100;
var eyemvmnt = 50;
var faceWidth = 400;
var faceHeight = 600;
var hatbottom = 320;
var hatbottomvmt = 320;
var Qhat = 160;
var Qhatx1y1x2y2 = 160;
var windw = 400;
var windh = 80;
function setup() {
createCanvas(640, 480);
}
function draw() {
background(232, 253, 255);
//wind movements
fill(181, 246, 255);
noStroke();
rect(windh, windw/4, windw, windh);
rect(windh * 2, windw/2, windw *2, windh/2);
rect(windh - windh, windw, windw, windh);
rect(windh * 5, windw/3.5, windw * 3, windh/2.5);
//face
fill(255, 199, 15);
ellipse(faceWidth * 0.8, faceHeight, faceWidth, faceHeight);
//two parts of the hat. The base and top.
fill(5, 59, 66);
rect(hatbottom/2, hatbottom/1.1, hatbottomvmt, hatbottom/4);
quad(Qhatx1y1x2y2, Qhatx1y1x2y2/4, Qhatx1y1x2y2 * 3, Qhatx1y1x2y2/4, Qhat * 2.6, Qhat * 2, Qhat * 1.3, Qhat * 2);
//variables for eyes
var eyeL = width / 2 - faceWidth * 0.20;
var eyeR = width / 2 + faceWidth * 0.20;
//eye
fill(255);
ellipse(eyeL, eyeSize * 4.5, eyeSize, eyeSize);
fill(255);
ellipse(eyeR, eyeSize * 4.5, eyeSize, eyeSize);
//pupil
fill(0);
ellipse(eyeL, eyeSize * 4.6, eyemvmnt,eyemvmnt);
ellipse(eyeR, eyeSize * 4.6, eyemvmnt,eyemvmnt);
}
function mousePressed() {
faceWidth = random(370, 450);
//only used to animate the width of the hat for perspective
//only changes the first two quadrants of the top hat to keep the parts connected
Qhatx1y1x2y2 = random(200,330);
eyemvmnt = random(40,60);
hatbottomvmt = random(350,400);
windh = random(20,100);
windw = random(200, 1000);
}
With this project, I focused on using random() to create movement and slight perspective shifts within the piece. With the face, only certain variables were animated to create a similar perspective shift to the hat. Rectangles in the background add depth and additional visual cues of wind as the hat jolts to the right side of the screen.