//Ankitha Vasudev
//ankithav@andrew.cmu.edu
//Section B
//Project 06
//global variables
var r = 148; // color of background
var g = 222;
var b = 255;
var r1 = 255; // color of starfish
var g1 = 130;
var b1 = 180;
var r2 = 120; // color of seaweed
var g2 = 200;
var b2 = 100;
var fishy = 200; //y position of fish
var x = [50, 65, 70, 62, 65, 50, 35, 39, 30, 45];
var y = [365, 372, 375, 380, 395, 385, 395, 380, 375, 370];
function setup() {
createCanvas(400, 400);
}
function draw() {
// Current Time
var H = hour();
var M = minute();
var S = second();
// Changes background color based on 8-hour periods
if (H >= 0 & H <= 8) {
background(r, g, b);
} else if (H > 8 & H < 16) {
background(r-50, g-50, b-20);
} else if (H >= 16 & H <= 24) {
background(r-150, g-150, b-50);
}
//position of fish according to seconds
fishx1 = map(S, 0, 59, 0, width+110);
fishx2 = map(M, 0, 59, width-20, -40);
fishx3 = map(H, 0, 23, width-20, -30);
//star color changes every second
//becomes lightest at the end of each minute
r1 = map(S, 0, 59, 200, 255);
g1 = map(S, 0, 59, 50, 200);
b1 = map(S, 0, 59, 50, 200);
//changes seaweed color every minute
r2 = map(M, 0, 59, 150, 0);
b2 = map(M, 0, 59, 120, 0);
//fish moving every second
noStroke();
fill(236, 185, 0);
ellipse(fishx1-55, fishy, 90, 30);
triangle(fishx1-95, fishy, fishx1-110, fishy-20, fishx1-110, fishy+20);
//fish moving every minute
fill(217, 180, 123);
ellipse(fishx2+20, fishy-100, 40, 15);
triangle(fishx2+30, fishy-100, fishx2+40, fishy-90, fishx2+40, fishy-110);
//fish moving every hour
fill(111, 72, 2);
ellipse(fishx3, fishy+100, 50, 15);
triangle(fishx3+20, fishy+100, fishx3+30, fishy+95, fishx3+30, fishy+105);
//seaweed
for (i = 0; i < width+5; i += 5) {
fill(r2, g2, b2);
triangle(i-5, height-30, i, height-60, i+15, height-30);
}
//sand
fill(240, 210, 142);
rect(0, height-40, width, 50);
//starfish
fill(r1, g1, b1);
beginShape();
for (var i = 0; i < 10; i++) {
vertex(x[i], y[i]);
endShape();
}
//text displaying time as reference
fill(100);
text("Hour: " + H, 10, 20);
text("Minute: " + M, 10, 35);
text("Second: " + S, 10, 50);
}
For this project I wanted to create an animation that included changing positions and colors according to the time. Each fish moves at a different pace – one every second, one every minute and one every hour. The starfish changes color every second and camouflages with the sand by the of every minute. The seaweed changes color every minute and is the greenest at the end of every hour. The color of the sea varies at 8-hour intervals to show the time of the day(morning, afternoon or evening).
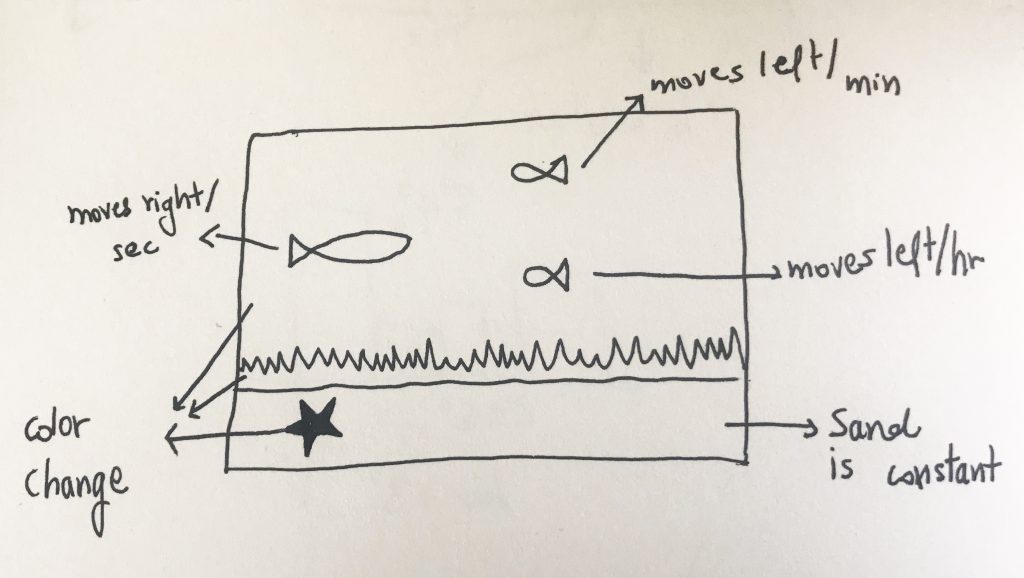