/*
* Angela Lee
* Section E
* ahl2@andrew.cmu.edu
* Project 6 Abstract Clock
*/
function setup() {
createCanvas(400, 400);
}
function draw() {
// background
var top = color(249, 237, 201); // pale yellow color
var bottom = color(237, 165, 117); // peach color
setGradient(top, bottom); // background gradient
// time
var hours = hour();
var minutes = minute();
var seconds = second();
var milli = millis();
// halo changes color with milliseconds
halo(milli);
// the shadow grows longer with hours
setting(hours);
// the drink color changes with minutes
behindGls(minutes);
// the foam bubbles move with milliseconds
foam(milli);
// the drink color changes with minutes
frontGls(minutes);
// the straw moves with the seconds
straw(seconds);
}
// BACKGROUND GRADIENT
function setGradient(color1, color2) {
for(var a = 0; a <= height; a++) {
var amount = map(a, 0, height, 0, 1);
var back = lerpColor(color1, color2, amount);
stroke(back);
line(0, a, width, a);
}
}
// ----------BELOW THIS LINE ARE PIECES OF THE DRAWING -----------
// HALO AROUND LATTE
function halo(milli) {
big = 300; // big circle size
med = 245; // medium circle size
small = 185; // small circle size
x = width/2 // x position
y = height/2 - 30; // y position
r = 15 * sin(milli / 1000.0);
g = 15 * sin(milli / 1000.0 + HALF_PI);
b = 30 * sin(milli / 1000.0 - HALF_PI);
stroke("white");
strokeWeight(1);
fill(249 + r, 240 + g, 155 + b);
ellipse(x, y, big, big);
strokeWeight(2);
fill(250 + r, 237 + g, 130 + b);
ellipse(x, y, med, med);
strokeWeight(3);
fill(252 + r, 240 + g, 84 + b);
ellipse(x, y, small, small);
}
// TABLE, SHADOW
function setting(hours) {
// table
noStroke();
fill(133, 88, 51);
rect(0, 2/3 * height, width, height/3);
// shadow
fill(112, 67, 34);
shadowW = map(hours, 0, 24, 160, 240);
ellipse(240, 321, shadowW, 65);
}
// FIRST LAYERS OF LATTE
function behindGls(minutes) {
// glass
glsX = 119.799; // glass x-coordinate
glsY = 148.193; // glass y-coordinate
glsW = 160.403; // glass width
glsH = 169.456; // glass height
glsEllipseH = 50.513; // glass ellipse height
fill(231, 239, 242);
rect(glsX, glsY, glsW, glsH);
ellipse(glsX + glsW / 2, glsY + glsH, glsW, glsEllipseH);
// drink
var clrChange = map(minutes, 0, 60, 0, 40);
Green = color(197 - clrChange, 201 - clrChange, 125 - clrChange);
drnkX = 128.906; // drink x-coordinate
drnkY = 148.193; // drink y-coordinate
drnkW = 142.071; // drink width
drnkH = 165.456; // drink height
drnkEllipseY = 313.65; // bottom of glass ellipse y-coordinate
drnkEllipseH = 40.477; // bottom of glass ellipse height
fill(Green);
rect(drnkX, drnkY, drnkW, drnkH);
ellipse(drnkX + drnkW / 2, drnkEllipseY,
drnkW, drnkEllipseH);
}
// FRONT LAYERS OF LATTE
function frontGls(minutes) {
clrChange = map(minutes, 0, 60, 0, 50);
// TOP GLASS ELLIPSE
fill(231, 239, 242);
ellipse(glsX + glsW / 2, glsY, glsW, glsEllipseH);
// GREEN TOP ELLIPSE
fill(166 - clrChange, 180 - clrChange, 76 - clrChange);
ellipse(glsX + glsW / 2, glsY, drnkW, drnkEllipseH);
}
// FOAM
function foam(milli) {
foamH = 41.592;
// body of the foam
fill(246, 249, 220);
rect(drnkX, drnkY, drnkW, foamH);
ellipse(drnkX + drnkW/2, drnkY + foamH, drnkW, drnkEllipseH);
ellipse(164.455, 203.479, 34.55, 25.72);
ellipse(206.343, 210.407, 62.807, 26.791);
ellipse(239.538, 202.514, 34.55, 25.72);
// big bubbles
fill(197, 201, 125);
bigBbl = 5; // size of big bubbles
bigBblX = [143, 163, 180, 216, 219, 245, 253]; // big bubble x values
bigBblY = [174, 195, 194, 208, 190, 200, 183]; // big bubble y values
change = sin(map(milli, 0, 60, 0, 1));
// the big bubbles move left and right depending on milliseconds
for (var i = 0; i < bigBblX.length; i++) {
ellipse(bigBblX[i] + change, bigBblY[i] + change, bigBbl, bigBbl);
}
// small bubbles
fill(216, 216, 160);
smlBbl = 5; // size of big bubbles
smlBblX = [156, 170, 212, 239, 245]; // small bubble x values
smlBblY = [203, 181, 194, 203, 177]; // small ubble y values
// the small bubbles move left and right depending on milliseconds
for (var i = 0; i < smlBblX.length; i++) {
ellipse(smlBblX[i] - change, smlBblY[i] - change, smlBbl, smlBbl);
}
}
// STRAW
function straw(seconds) {
strX = map(seconds, 0, 60, 141, 218.587); // straw x-coordinate
strY = 210.407; // straw y-coordinate
strW = 12.49; // straw width
strH = 94.115; // straw height
strEllipseH = 4.065; // ellipse straw height
fill("white");
rect(strX, strY - 150, strW, strH);
ellipse(strX + strW/2, strY - 150 + strH, strW, strEllipseH);
ellipse(strX + strW/2, strY - 150, strW, strEllipseH);
// shadow within the straw
fill(232, 232, 232);
ellipse(strX + strW/2, strY - 150, strW - 2, strEllipseH - 1);
}
As I was sitting down to plan out my abstract clock, I was craving a matcha latte so I decided to make that the main visual of my clock. I wanted to create a piece that represented time in a more abstract manner, so I decided to make parts of my matcha latte move or change in accordance to different time variables. To plan out my piece, I first sketched out my matcha latte.
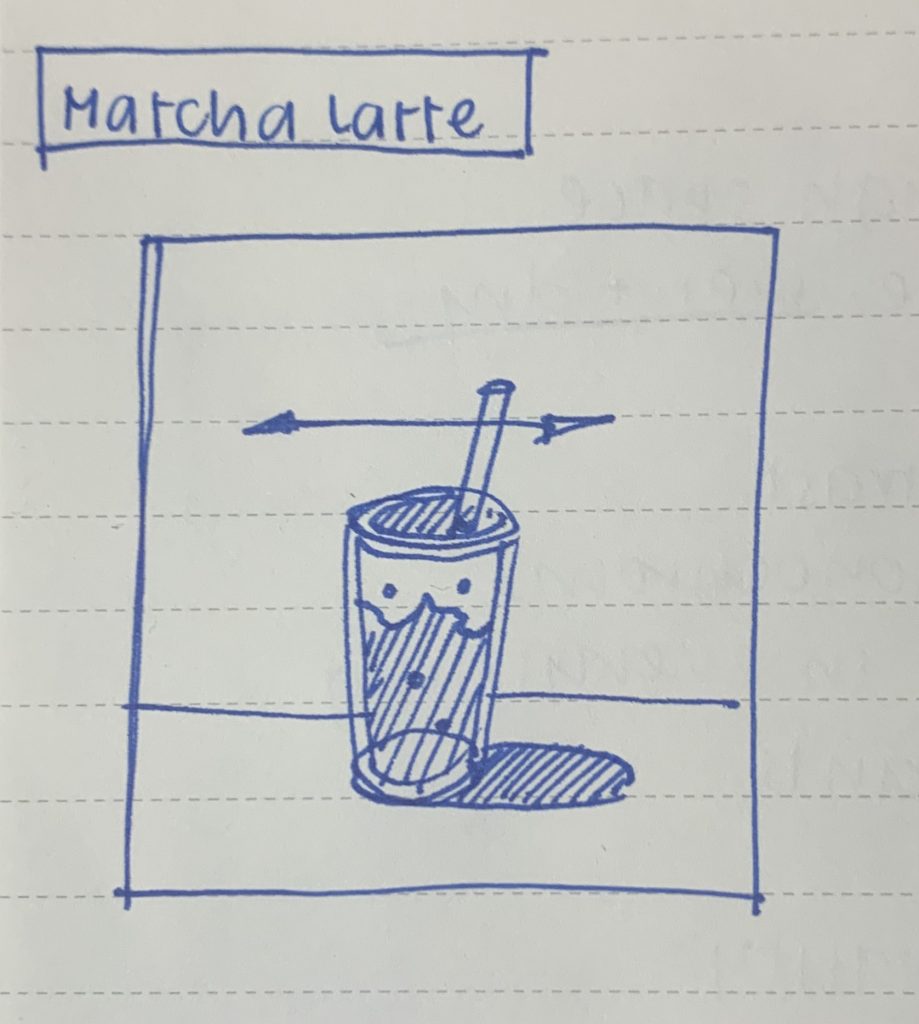
As I began to code the composition, I realized that I wanted more contrast between the latte and the background. So, I added a couple of halo-like ellipses to establish a focal point around the latte.