//Fanjie Jin
//Section C
//fjin@andrew.cmu.edu
//Project 7
function setup(){
createCanvas(480, 480);
//set a initial start x and y value
mouseX = 100;
mouseY = 100;
}
function draw(){
background(100);
push();
//tranlate geometry to the center of the canvas
translate(240, 240);
//constrain the mouse x value
var x = constrain(mouseX, 5, 480);
//constrain the mouse y value
var z0 = constrain(mouseY, 0, 240);
//constrain the mouse y value
var z1 = constrain(mouseY, 0, 240);
//constrain the mouse y value
var z2 = constrain(mouseY, 0, 360);
//remap the value of mouse X
var a = map(x, 0, 480, 0, 200);
//remap the value of mouse X
var b = map(x, 0, 480, 60, 60);
//remap the value of mouse X
var c = map(x, 0, 480, 0, 100);
noFill();
beginShape();
//inside circle size variable
//Hypotrochoid Variation 1
for (var i = 0; i < 120; i++) {
//defining the positions of points
//parametric eq1
x1 = (b - a) * sin(i) + z0 * sin((b - a) * i);
//parametric eq2
y1 = (b - a) * cos(i) - z0 * cos((b - a) * i);
//color variation
stroke(b * 2, a * 3, a + b);
vertex(x1, y1);
};
endShape();
//Hypotrochoid Variation 2
noFill();
beginShape();
for (var i = 0; i < 120; i++) {
//parametric eq3
x2 = (b - a) * cos(i) + z1 * cos((c - a) * i);
//parametric eq4
y2 = (b - a) * sin(i) - z1 * sin((c - a) * i);
stroke(b , c, a + b);
vertex(x2, y2);
};
endShape();
//Hypotrochoid Variation 3
noFill();
beginShape();
for (var i = 0; i < 120; i++) {
//parametric eq5
x3 = (b - a) * sin(i) + z2 * sin((a - c) * i);
//parametric eq6
y3 = (b - a) * cos(i) - z2 * cos((a - c) * i);
stroke(a , c, b);
vertex(x3, y3);
};
endShape();
pop();
}
In this project, I have tried to use the equation for Hypotrochoid to generate some mathematically interesting compositions. The equation express is above; however, I have simplified and add another parameter to the equation so that more parametric shapes would be generated. I really enjoyed this project as I think it is really great to learn how to actually use these mathematical to generate patterns and sometimes when you move the mouse a tiny bit, the entire composition will be drastically changed. In this project, there are three rings and each of them has a different equation and they would move simultaneously once the mouse position is changed.
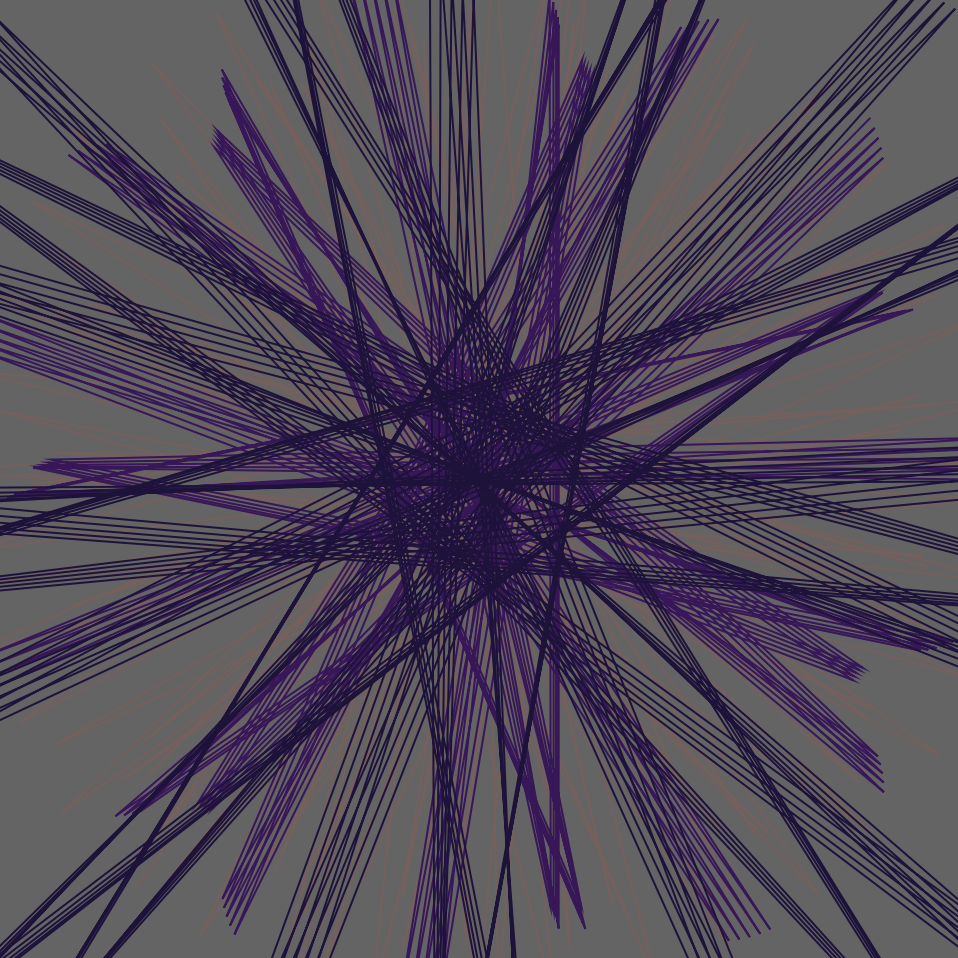
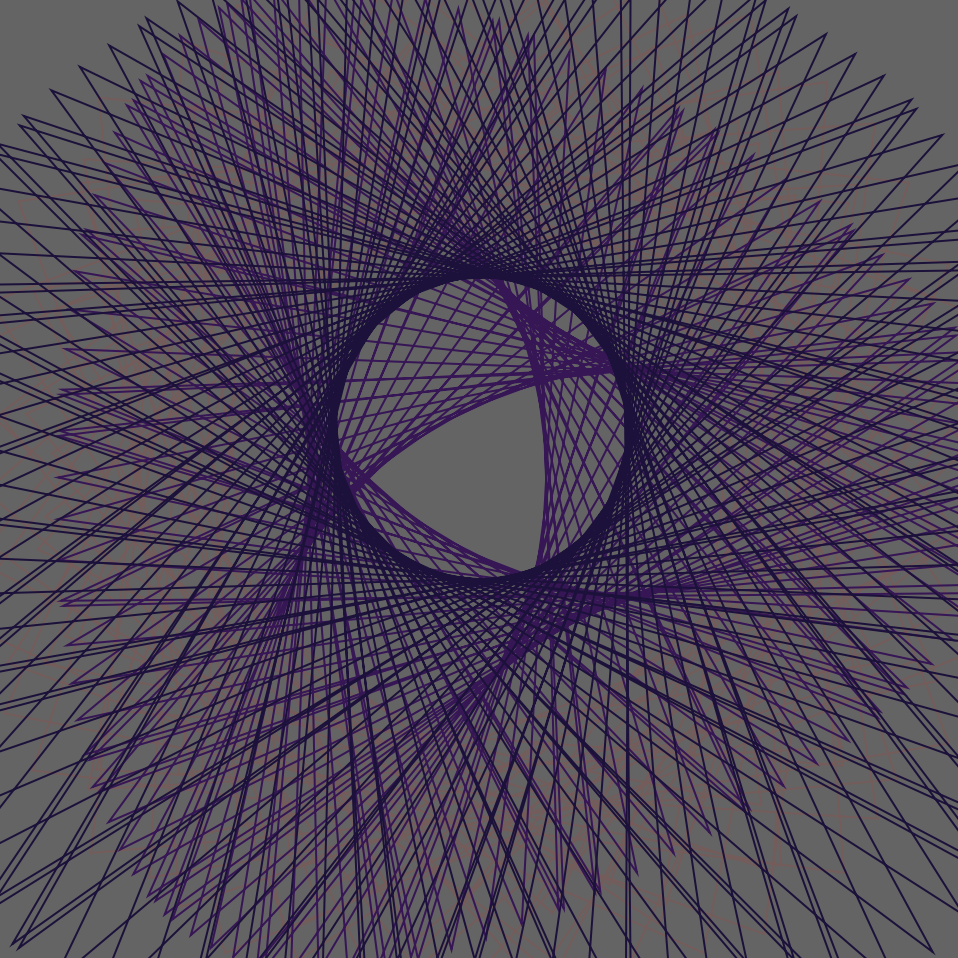
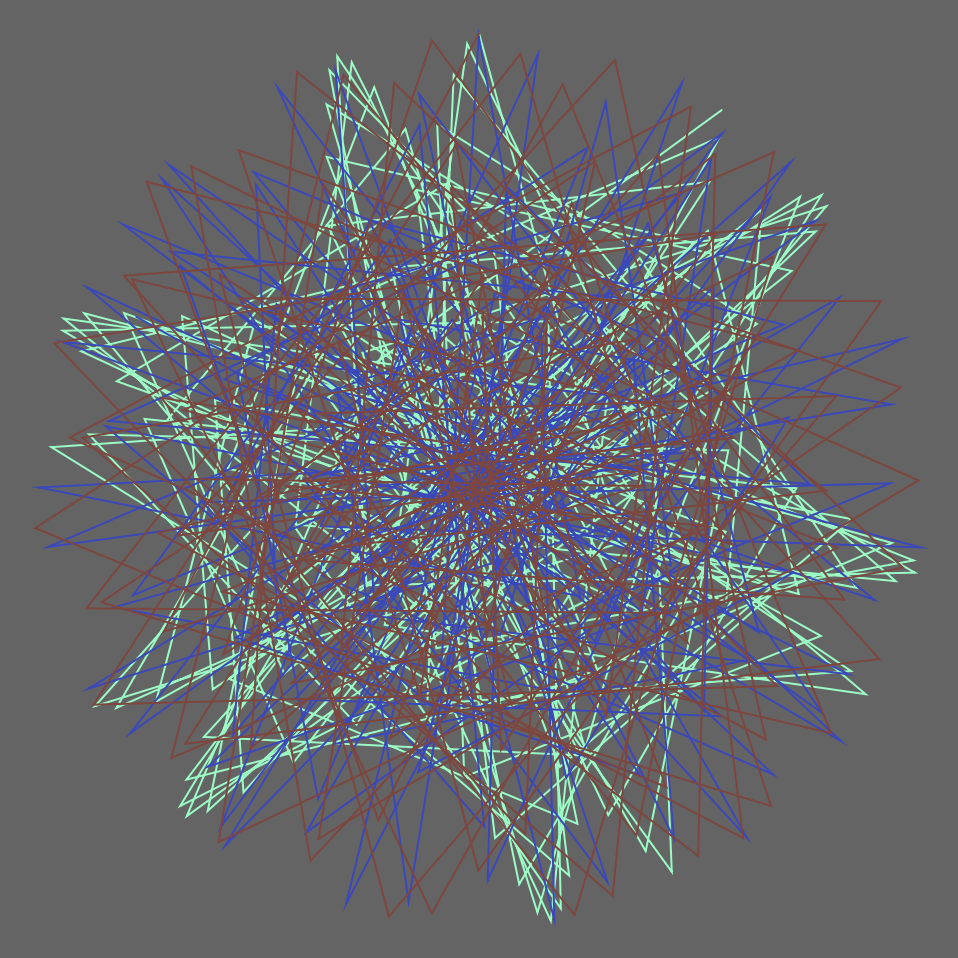