//Margot Gersing - Project 11 - mgersing@andrew.cmu.edu - section E
var stars = [];
var terrainSpeedOne = 0.0002; //dark green moutain speed
var terrainSpeedTwo = 0.0004; // water speed
var terrainSpeedThree = 0.0006; // ligth green ground speed
var terrainDetailOne = 0.008; //green moutian
var terrainDetailTwo = 0.001; //water
var terrainDetailThree = 0.005; //light green moutain
function setup() {
createCanvas(480, 480);
frameRate(20);
//fills stars array
for (var i = 0; i < 75; i ++){
var strX = random(width);
var strY = random(0, height / 2);
stars[i] = makeStars(strX, strY);
}
}
function draw() {
background(214, 197, 204);
//moon
fill(169, 47, 35);
ellipse(375, 100, 150, 150);
displayStars(); //stars
moutainTwo(); //background moutians
water(); //water
moutainThree(); //foreground
}
function moutainTwo() {
noStroke();
fill(22, 56, 32); //darkgreen
beginShape();
for(var x = 0; x < width; x++){
var t = (x * terrainDetailOne) + (millis() * terrainSpeedOne);
var y = map(noise(t), 0, 1, 300, 250);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape(CLOSE);
}
function water() {
noStroke();
fill(72, 90, 103); //blue
beginShape();
for(var x = 0; x < width; x++){
var t = (x * terrainDetailTwo) + (millis() * terrainSpeedTwo);
var y = map(noise(t), 0, 2, 400, 300);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape(CLOSE);
}
function moutainThree() {
noStroke();
fill(110, 135, 84); //light green
beginShape();
for(var x = 0; x < width; x++){
var t = (x * terrainDetailThree) + (millis() * terrainSpeedThree);
var y = map(noise(t), 0, 2, 500, 300);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape(CLOSE);
}
function drawStars(){ //what stars will look like
noStroke();
fill(201, 165, 180, 150);
push();
translate(this.x, this.y);
ellipse (1, 10, 5, 5);
pop();
}
function makeStars(strX, strY){ //stars object
var star = {x: strX,
y: strY,
speed: -1,
move: moveStars,
draw: drawStars}
return star;
}
function moveStars(){ //how stars move
this.x += this.speed;
if(this.x <= -10){
this.x += width;
}
}
function displayStars(){ //display stars
for(i = 0; i < stars.length; i++){
stars[i].move();
stars[i].draw();
}
}
For this project I decided to go with mountain landscapes. I had a good time messing with colors to create something a little different. I also generated stars in the sky. I decided to make the stars transparent so they were more subtle and you could see them pass over the moon. I also made the objects further back move slower so it would imitate what you see when you look out the window.
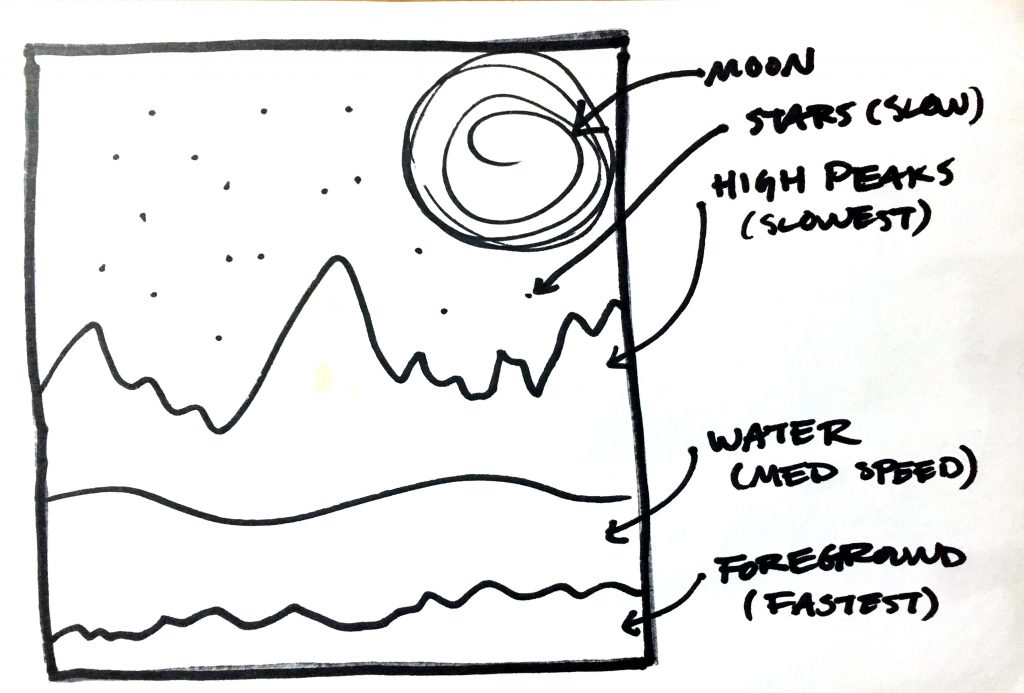