Air Max Campaign for Nike by ManvsMachine.
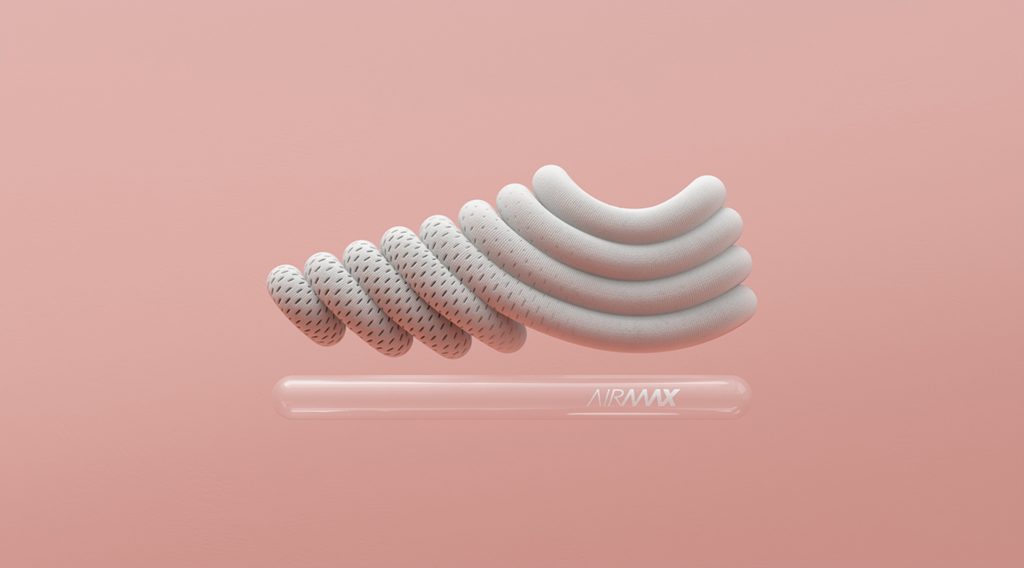
In this campaign, ManvsMachine created a series of 3d computer graphics that abstracted Nike’s Air Max sneaker into a layered composition. This project is admirable in its use of different textures to create variation between the abstracted shoes. Shading, transparency, and texture all help the graphics appear 3d. The textures he uses communicates the message of the shoe campaign as they demonstrate the airiness and lightness of the shoe, while his render of the shoe form composed from stones communicates a different aspect of the shoe’s performance.
The shadows casted on the graphics are what truly help make it appear 3d. It gives dimension to the illustration and generates surfaces that bend, fold, and capture the light differently. In the campaign video linked below, ManvsMachine animates the graphics in a way that responds to the textures of the graphics which additionally helps make them appear 3d and have qualities that are bound to the physics of our world like gravity.
Overall this project creatively uses all these methods to simulate the 3d experience of the graphic.