//Rachel Kim
//15-104(Section C)
//rachelki@andrew.cmu.edu
//Project 09
var friendJer; //variable name for original image
var sentence; //variable name for array of words
function preload() {
friendJer = loadImage("https://i.imgur.com/ykv4p2z.jpg"); //loading in original image
sentence = ["This", "is", "my", "friend", "Jeremy", "!!!"] //sentence explaining who the person is in the picture
}
function setup() {
createCanvas(480, 300);
noStroke();
background(0); //black background
friendJer.resize(480, 300); //image to fit canvas
imageMode(CENTER); //placing image @ center
friendJer.loadPixels(); //loading words
frameRate(50); //speed of rendering
}
function draw() {
var sentX = random(friendJer.width); //random x-coordinates for words
var sentY = random(friendJer.height); //random y-coordinates for words
var ix = constrain(floor(sentX), 0, width);
var iy = constrain(floor(sentY), 0, height);
var xyColorLocation = friendJer.get(ix, iy); //color of original image @ xy location
textSize(12); //size of words
textFont('Georgia'); //type of font
if (sentY < 100) {
if (sentX < 150) {
fill(xyColorLocation);
text(sentence[0], sentX, sentY); //randomly placed "This"
} else if(sentX >= 150 & sentX < 200) {
fill(xyColorLocation);
text(sentence[1], sentX, sentY); //randomly placed "is"
} else {
fill(xyColorLocation);
text(sentence[2], sentX, sentY); //randomly placed "my"
}
} else if(sentY >= 100 & sentY < 350) {
if(sentX < 200) {
fill(xyColorLocation);
text(sentence[3], sentX, sentY); //randomly placed "friend"
} else if(sentX >= 200 & sentX < 480) {
fill(xyColorLocation);
text(sentence[4], sentX, sentY); //randomly placed "Jeremy"
} else {
fill(xyColorLocation);
text(sentence[5], sentX, sentY); //randomly placed "!!!"
}
}
}
For this project, I wanted to use a portrait of my friend’s side profile. Because the code renders the original image into an abstract image, I used words to load the image. The words are a sentence that states, “This is my friend Jeremy”. That way, the viewer knows who the person is. Below, is the rendering process of the portrait.
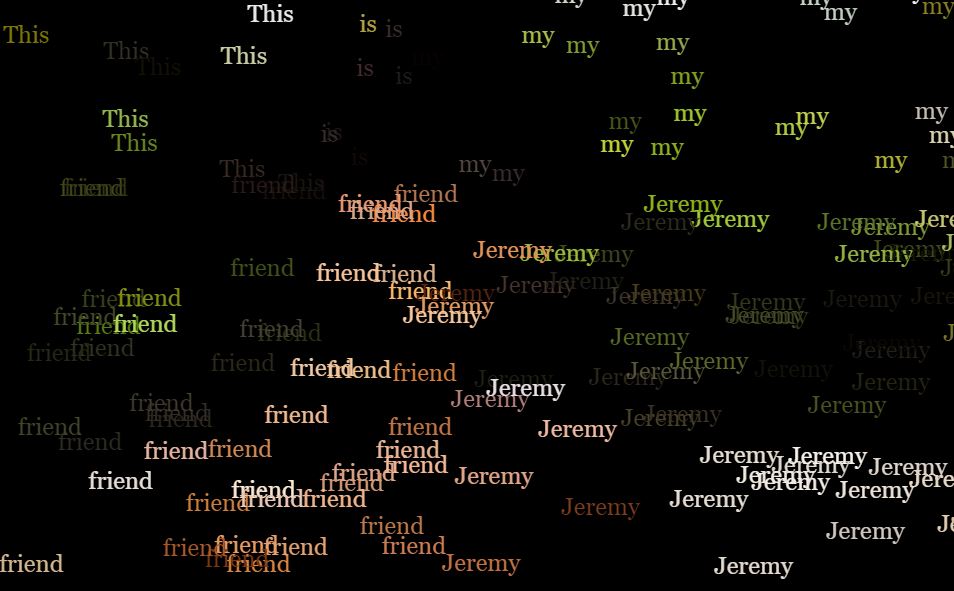
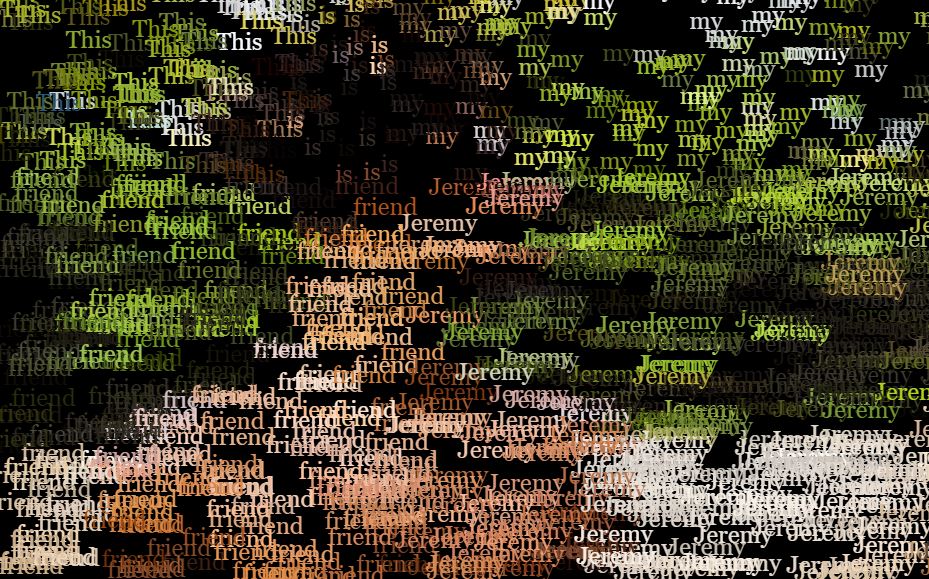
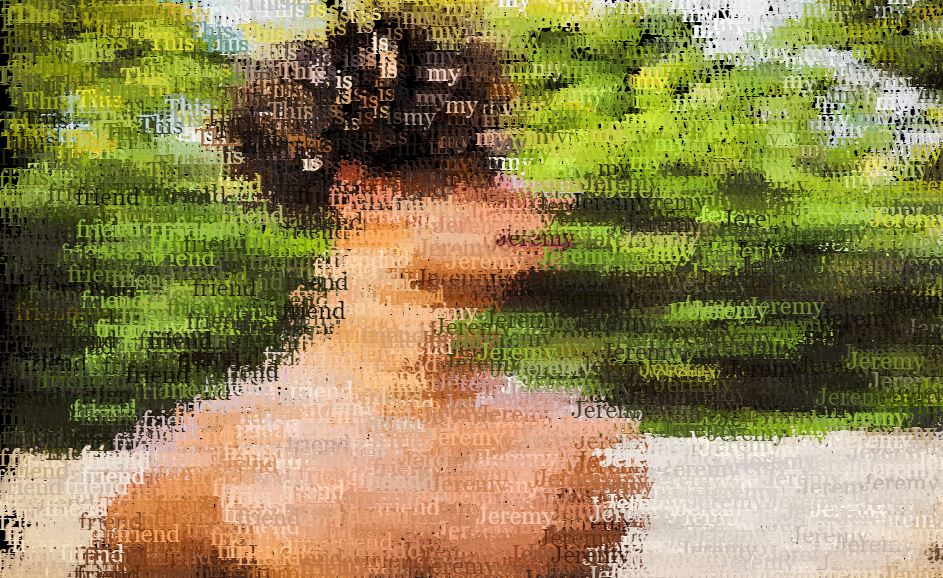