For my abstract clock, I chose to create an illustration of chickens in honor of a bunch of chickens I saw sitting in a pine tree the other day. I had a lot of trouble with this project because I’m still not very good at using arrays and loops yet, but I think the end result is pretty cute.
- Random pieces of corn sprinkle per second.
- The eating chicken moves every second and crosses the screen every minute.
- The standing chicken moves every minute and crosses the screen every hour.
- The background gets darker/more purple every hour into the night and lighter/more green every hour into the day.
//Maggie Ma
//Section D
var x=0; //x position of walking chicken
var y=140; //y position of walking chicken
var eaty=40; //y position of eating chicken
var h; //hours
var m; //minutes
var s; //seconds
function setup() {
createCanvas(480, 480);
noStroke();
frameRate(1);
}
function draw() {
h=hour();
m=minute();
s=second();
//background changes color every hour and gets darker at night
if(h<12) {
background(80,20*h,50);
} else {
background(80,255-20*(h-12),50);
}
//corn that don't move
fill(255,221,21);
ellipse(115,376,8);
ellipse(280,421,8);
ellipse(422,359,8);
ellipse(138,362,8);
ellipse(73,301,8);
ellipse(108,433,8);
//chicken standing walks across screen every hour
push();
translate(map(m,0,60,-80,width+50), y);
WalkingChicken();
pop();
//pieces of corn pop up every second
fill(255,221,21);
ellipse(random(3,477),random(250,477),8);
ellipse(random(3,477),random(250,477),8);
ellipse(random(3,477),random(250,477),8);
ellipse(random(3,477),random(250,477),8);
ellipse(random(3,477),random(250,477),8);
//chicken eating walks across screen every minute
push();
translate(map(-s, 0,20,200,width-70), eaty);
EatingChicken();
pop();
}
function EatingChicken(x,y) {
push();
translate(x,y);
//beak
fill(254,183,20);
arc(248,354,35.434,36.324,radians(150),radians(310),CHORD);
arc(245,350,20.866,27.618,radians(-90),radians(100),CHORD);
//feet
stroke(254,183,20);
strokeWeight(4);
line(362,334,352,361);
line(379,327,366,361);
line(331,361,381,361);
//crown
noStroke();
fill(198,56,68);
arc(228,296,59,59.358,radians(-4),radians(150),CHORD);
arc(241,295,30.434,33,radians(220),radians(400), CHORD);
arc(252,290,30.434,33,radians(-90),radians(90),CHORD);
//back tail wing
fill(50);
arc(359,167,101.194,111.382,radians(90),radians(270),CHORD);
arc(368,311,43.28,42.284,radians(-40),radians(160),CHORD);
//body
fill(245);
arc(297,247,213,199,radians(320),radians(130),CHORD);
circle(250,335,40);
//front wing
fill(50);
arc(320,253,110.751,111.427,radians(320),radians(130),CHORD);
//front tail wing
fill(245);
arc(391,176,98.952,95.477, radians(135),radians(-45),CHORD);
arc(353,320,43.299,45.877,radians(-20),radians(160),CHORD);
triangle(264,349,264,334,288,341);
//gobbler thing
fill(198,56,68);
ellipse(273,353,15.903,15.903);
triangle(252,354,271,361,271,345);
//eye
fill(0);
circle(244,347,4.771);
pop();
}
function WalkingChicken(x,y) {
push();
translate(x,y);
//crown
fill(198,56,68);
arc(34,-105,46,43,radians(-4),radians(150),CHORD);
arc(29,-93,40,36,radians(100),radians(-80), CHORD);
arc(19,-95,40,37,radians(45),radians(225),CHORD);
//beak
fill(254,183,20);
arc(44,-61,30.1,26.455,radians(180),radians(360),CHORD);
arc(39,-64,30.1,16,radians(0),radians(180),CHORD);
//feet
stroke(254,183,20);
strokeWeight(4);
line(0,90,0,134);
line(-12,134,16,134);
line(15,78,44,78);
line(44,78,45,100);
line(45,100,32,109);
line(32,109,25,103);
//back tail
noStroke();
fill(231,231,233);
arc(-65,-19,105,102.244,radians(225),radians(405),CHORD);
//body
fill(209,210,212);
arc(0,0,156,163,radians(0),radians(180),CHORD); //234,229
arc(2,6,151,150, radians(-90),radians(90),CHORD);
rect(2,-88,45,30,10);
arc(0,76,44,40,radians(-20),radians(190),CHORD);
//tail
arc(-79,6,95.1,91.244,radians(180),radians(360),CHORD);
//front wing
fill(231,231,233);
arc(-3,12,102,102.244,radians(0), radians(180),CHORD);
//gobbler
fill(198,56,68);
ellipse(44,-44,16,16);
triangle(36,-43,43,-64,52,-43);
//eye
fill(0);
ellipse(39,-69,4.771,4.771);
pop();
}
To start, I created some sketches on paper, then created my chickens in Illustrator.
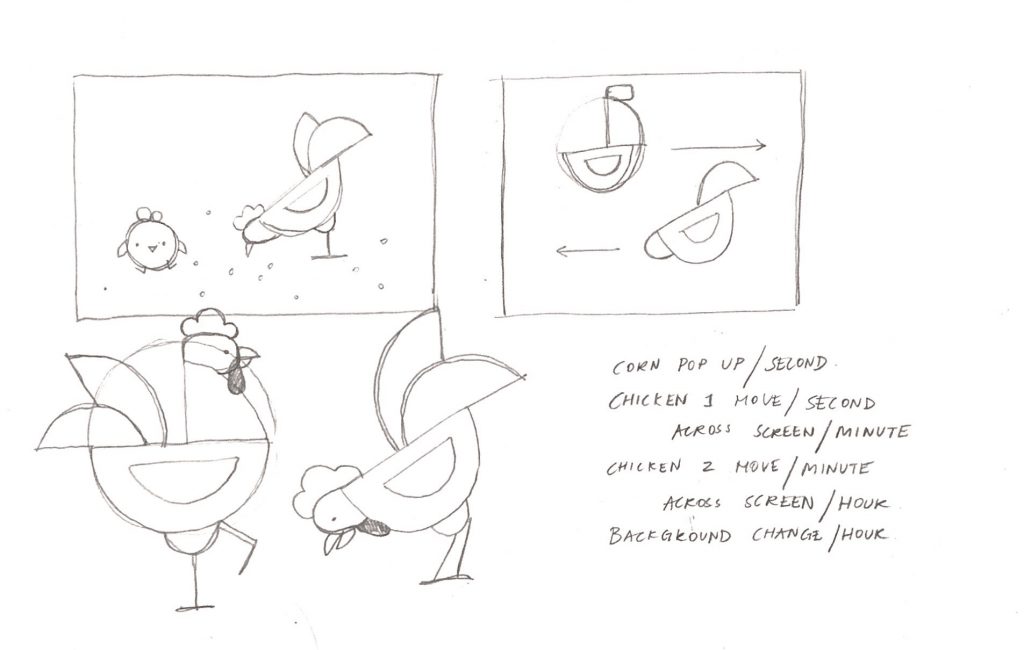
Right: My vector illustrations in Illustrator
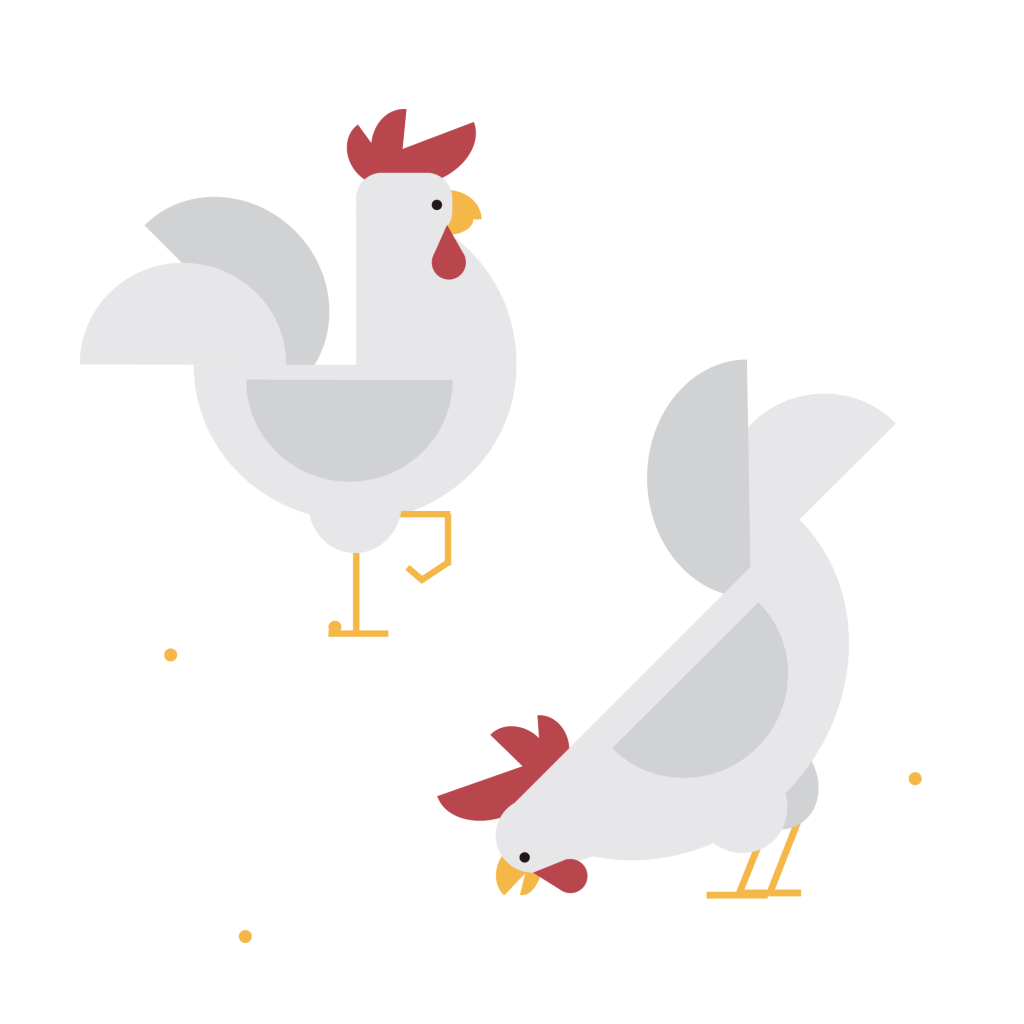
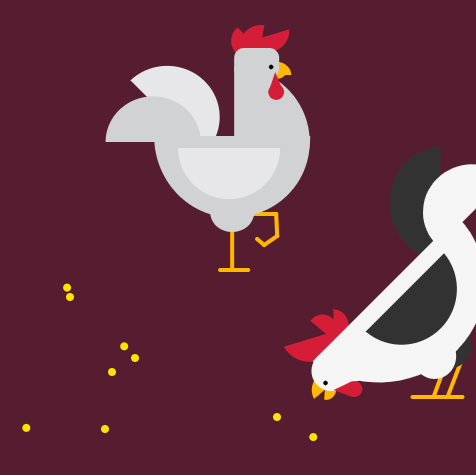
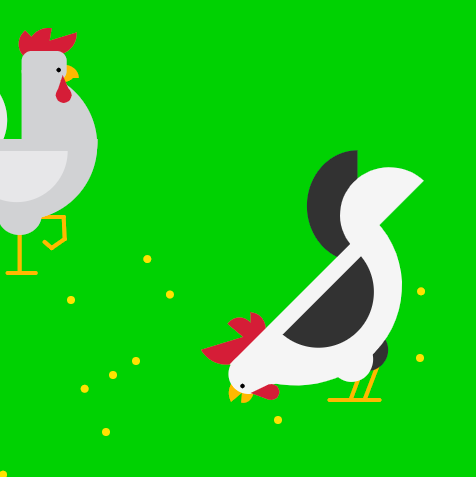
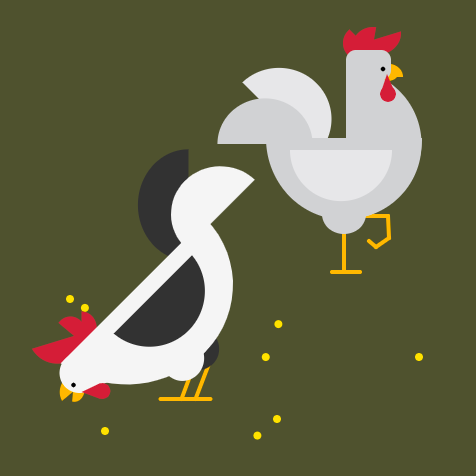