//gnmarino
//Gia Marino
//Section D
var sizeChange = .1; //makes everything 1/10th the original size
function setup() {
createCanvas(600, 600);
background(121, 181, 177); //cyan
}
function draw() {
fill(250, 250, 236); //cream
stroke(169, 96, 0); //dark orange
scale(sizeChange);
// makes the 1st row of mandalas and alternates every other row
// continues till it reaches border of canvas
for (var xSpacing = 0; xSpacing < 10000; xSpacing += 900){
for (var ySpacing = 0; ySpacing < 10000; ySpacing += 1800){
//push and pop makes it so spacing doesn't compound
push();
mandala(xSpacing, ySpacing);
pop();
}
}
// makes 2nd row of mandalas and alternates every other row
//continues till it reaches the point where it will not be on the page
for (var xSpacing = -450; xSpacing < 9000; xSpacing += 900){
for (var ySpacing = 900; ySpacing < 10000; ySpacing += 1800){
//push and pop makes it so spacing doesn't compound
push();
mandala(xSpacing, ySpacing);
pop();
}
}
//draws diamond pattern
for (var dxSpacing = 450; dxSpacing < 9000; dxSpacing += 900){
for (var dySpacing = 450; dySpacing < 10000; dySpacing += 900) {
//push and pop makes it so spacing doesn't compound
push();
diamond(dxSpacing, dySpacing);
pop();
}
}
noLoop();
}
function diamond (x2, y2) {
// translate with parameters allows the loop functions in draw to move the whole design easily
translate(x2, y2);
//push and pop to avoid settings effecting other functions
push();
translate (300, 300);
noStroke();
fill(169, 96, 0); //dark orange
triangle (-30, 0, 30, 0, 0, 30);
triangle(-30, 0, 30, 0, 0, -30);
pop();
}
function mandala(x, y) {
// translate with parameters allows the loop functions in draw to move the whole design easily
translate (x, y);
//whole shape is roughly 500 X 600 pixels before scaling
threeLeafShape();
circleBorder();
flowerAndCircle();
}
function threeLeafShape() {
//push pop makes it so the settings in this function doesn't effect other functions
push();
//moves top leaf to the middle of the mandala
translate(200, 0);
strokeWeight(5);
//draws top leaf
beginShape();
vertex(100, 200);
quadraticVertex(70, 125, 0, 100);
quadraticVertex(60, 90, 85, 115);
quadraticVertex(90, 40, 50, 20);
quadraticVertex(115, 40, 115, 115);
quadraticVertex(135, 85, 175, 80);
quadraticVertex(120, 125, 100, 200);
endShape();
//push pop makes it so the translation and rotation for the bottom leaf does not effect top leaf
push();
//translate and rotate moves bottom leaf so it's mirrored directly undernenth top leaf
translate(200, 600);
rotate(radians(180));
//draws bottom leaf
beginShape();
vertex(100, 200);
quadraticVertex(70, 125, 0, 100);
quadraticVertex(60, 90, 85, 115);
quadraticVertex(90, 40, 50, 20);
quadraticVertex(115, 40, 115, 115);
quadraticVertex(135, 85, 175, 80);
quadraticVertex(120, 125, 100, 200);
endShape();
pop ();
pop();
}
function circleBorder () {
//push pop makes it so the settings in this function doesn't effect other functions
push();
//translate to center of canvas to make it easier to make a border in a circular formation
translate(300, 300);
strokeWeight(4);
//radius of basline circle for the tiny circles to follow and make a border along
var r = 250;
//makes tiny circle border on right side
//constrained from theta -55 to theta 55 so circles do not collide with the 2 leaf shapes
for (var theta = -55; theta < 55; theta += 15) {
cx = r * cos(radians(theta));
cy = r * sin(radians(theta));
circle(cx, cy, 40);
}
//makes tiny circle border on left side
//constrained from theta 125 to theta 235 so circles do not collide with the 2 leaf shapes
for (var theta = 125; theta < 235; theta += 15) {
cx = r * cos(radians(theta));
cy = r * sin(radians(theta));
circle(cx, cy, 40);
}
pop();
}
function flowerAndCircle () {
//(push pop 1)push and pop make it so translated origin does not effect next function
push();
//puts origin in center so it is easier to draw in a circular formation
translate (300, 300);
//circle around flower
//push and pop so nofill doesn't effect flower
push();
noFill();
strokeWeight(5);
circle(0, 0, 200);
pop();
//flower center
strokeWeight(5);
circle (0, 0, 35);
//draws 8 flower petals that rotates around the center origin
for (theta = 30; theta <= 360; theta += 45){
//(push pop 2)
//the push and pop in this loop makes it so it rotates only 45 each time and not 45 + theta
push();
rotate(radians(theta));
ellipse( 55, 0, 45, 20);
//pop uses push 2
pop();
}
//this pop uses push 1
pop();
}
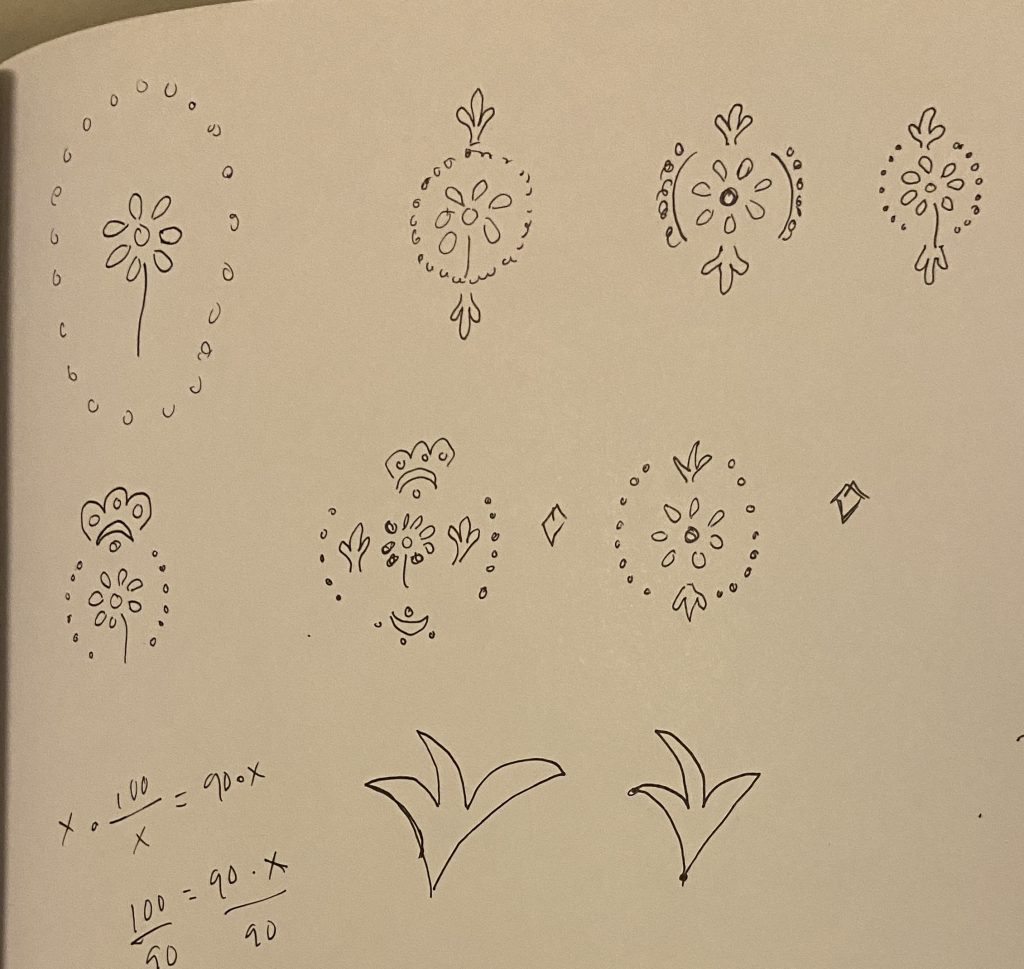
I started off with deciding a design that I could then repeat over and over. Then after I decided, I made the design in a 500×600 pixels dimensions. Then I scaled it down and looped it. Then I felt like the pattern needed something in-between the mandala I created so I made a diamond and made a separate nested loop to repeat it so it went in the empty spaces. I had to make two nested loops for the mandala so the columns were staggered and not lined up perfectly. For the circle border I used polar coordinates so I could space it out and also not have to complete the circle. But for the flower I had to use rotation so the ellipse turned and went in a circle.
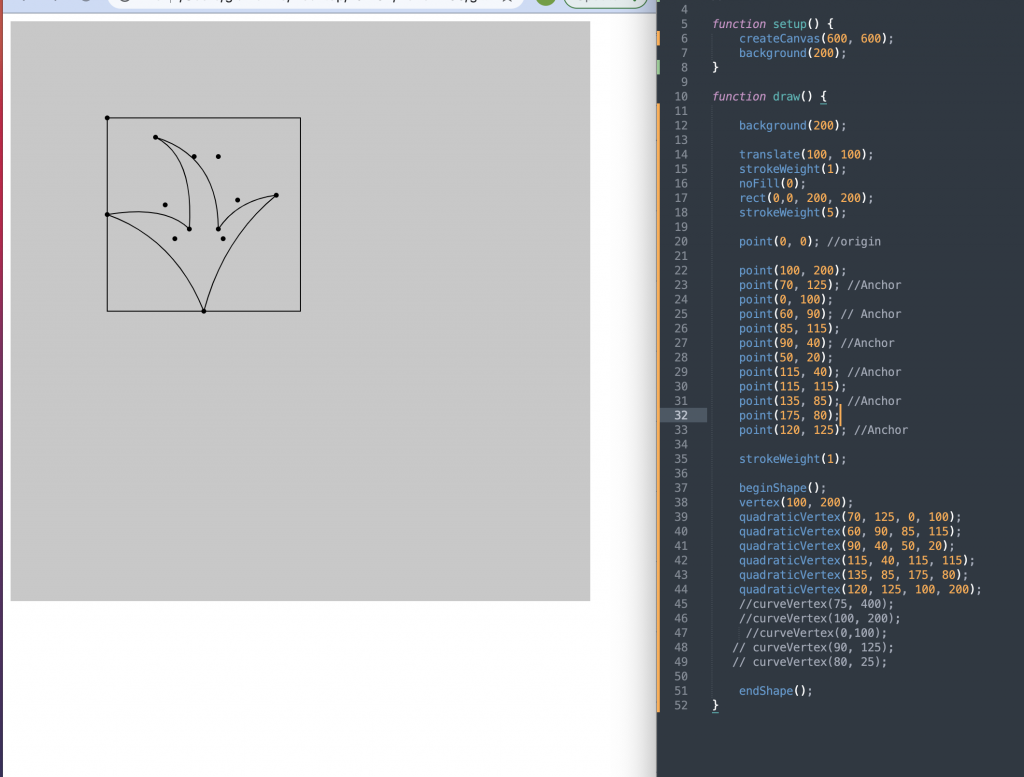