// gnmarino@andrew.cmu.edu
// Gia Marino
// section D
var red; // variable for red in fill for gradient
var blue; // variable for blue in fill for gradient
function setup() {
createCanvas(400, 400);
background(200);
}
function draw() {
// maps variables red and blue so mouseX at 0 makes fill blue
// and at edge of the canvas mouseX makes color red
// creates a blue to red gradient
red = map(mouseX, 0, width, 0, 255);
blue = map(mouseX, width, 0, 0, 255);
// fills shapes and makes a red to blue gradient
fill(red, 0, blue);
// nested loop draws astroids going to the right and skips every other row
for (var y1 = 0; y1 <= width; y1 += 4*size) {
drawAstroid(0, y1);
for(var x1 = 0; x1 <= width + size; x1 += 3) {
drawAstroid(x1, y1);
}
}
// nested loop draws astroids going to the left and skips every other row
for (var y2 = 2 * size; y2 <= width; y2 += 4*size) {
drawAstroid(width, y2);
for(var x2 = width; x2 >= 0 - size; x2-=5) {
drawAstroid(x2, y2);
}
}
}
function drawAstroid(x, y) {
// push needed so translate doesn't effect rest of code
push();
translate(x, y);
beginShape();
// loop and astriod x and y implements math for astroid curve
for(var k = 0; k<360; k++){
// allows for mouseY to change size of astroid between 20 up to 80 pixels
size = map(mouseY, 0, height, 20, 80);
Astroidx = size * Math.pow(cos(radians(k)),3);
Astroidy = size * Math.pow(sin(radians(k)),3);
vertex(Astroidx,Astroidy);
}
endShape(CLOSE);
pop();
}
For this week I had a really difficult time figuring out what curves would work for my ideas. I originally wanted to try a whirl with a logarithmic spiral but it was too difficult to implement the math. I then found the astroid shape and thought it was cool so I figured out how to implement it into code and then I made it move with mouse X and mouse Y tried to find a pattern or drawing that would look cool. I eventually found a pattern by doing this and decided my idea. I then decided my parameters would be changing the size of the astroid which therefore changes how many rows you see, and the color.
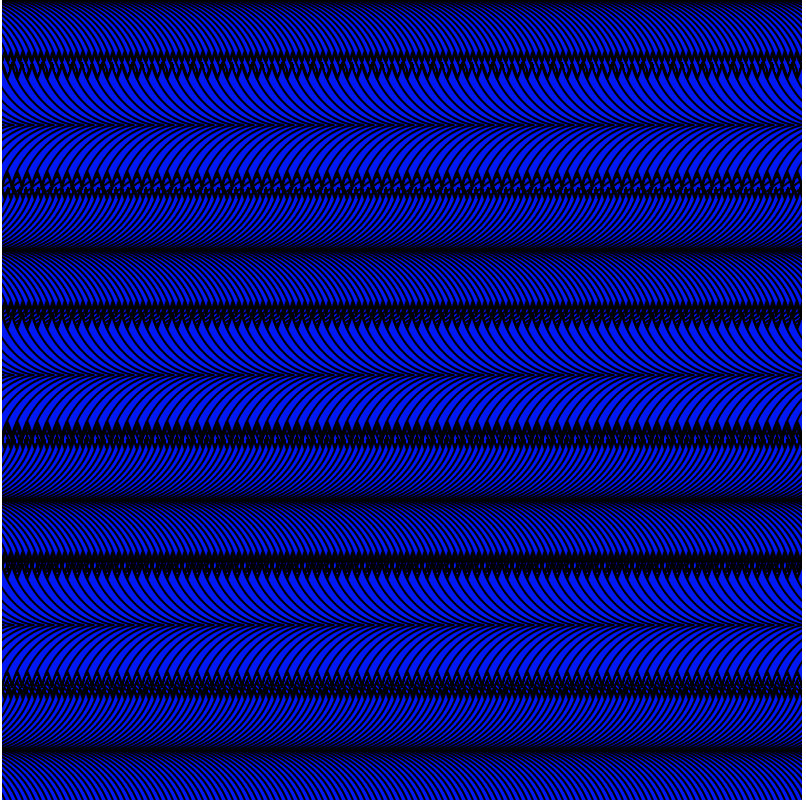
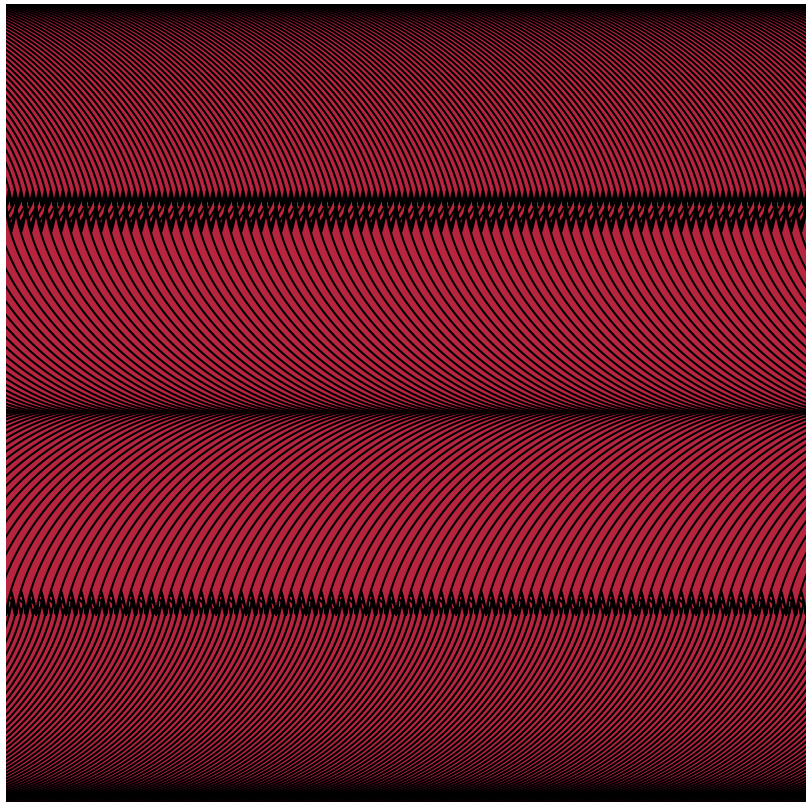