For this project, I was excited to explore different types of curves and how you can create new ones through simple interactions. I started off my process by looking at and trying different curves in Sublime to see which ones were the most interesting to change. I also spent some time plugging mouseX and mouseY into different parts of the equation to see how they changed. I found it interesting that curves can look completely different based on which variables were changed. For instance, I used a Cayley’s Sextic curve for one of my curves, which looks like a rounded heart. However, when you control the ratio multiplied by cosine, it changes the number of curves and their rotation so it looks like a flower.
Astroid curve in different phases:
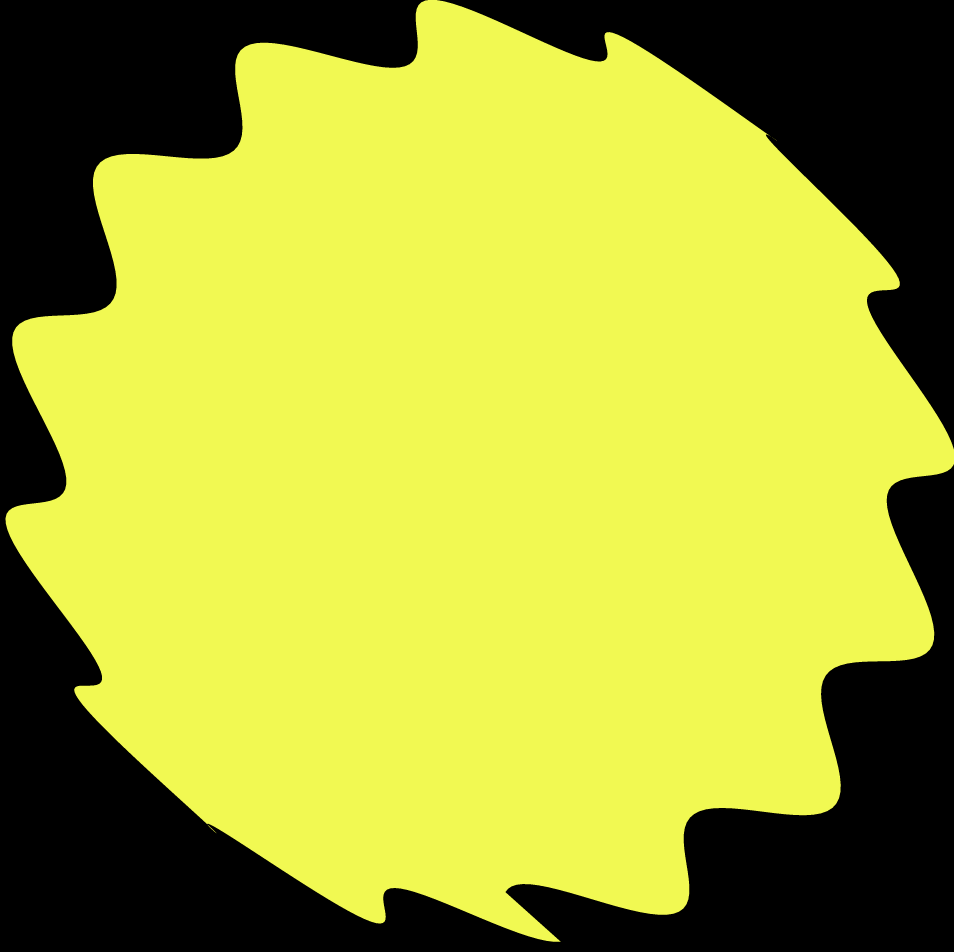
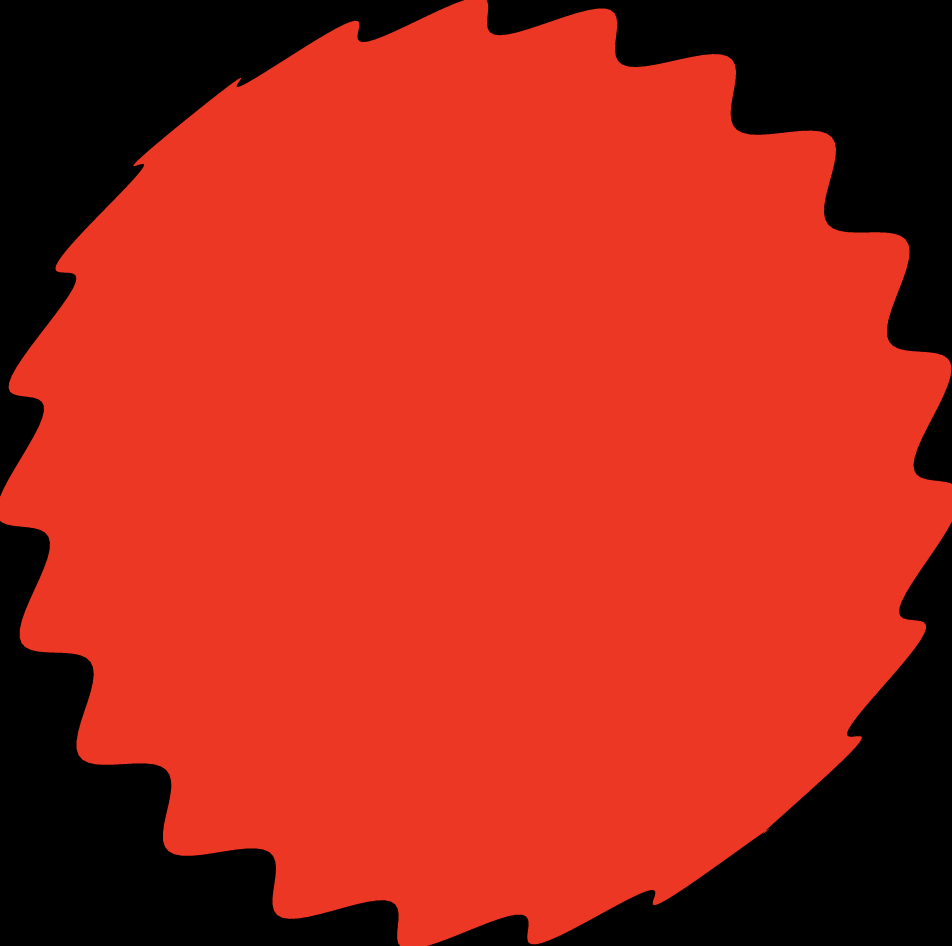
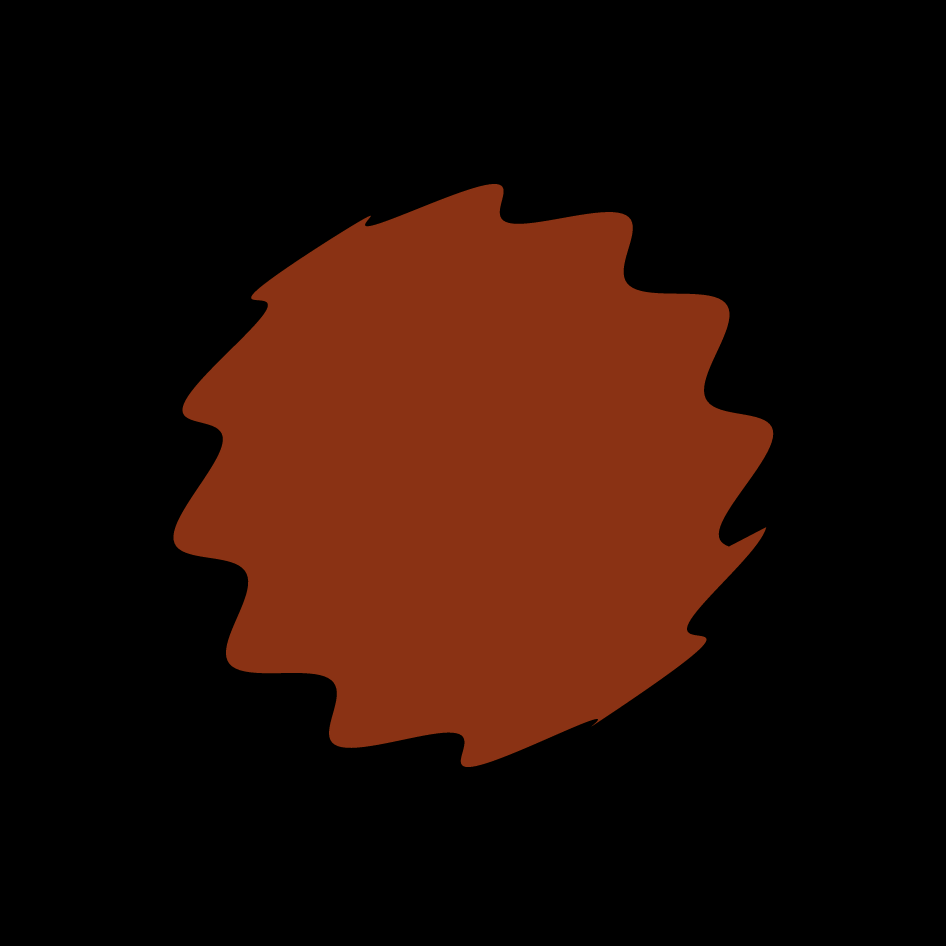
Cayley’s Sextic in different phases:
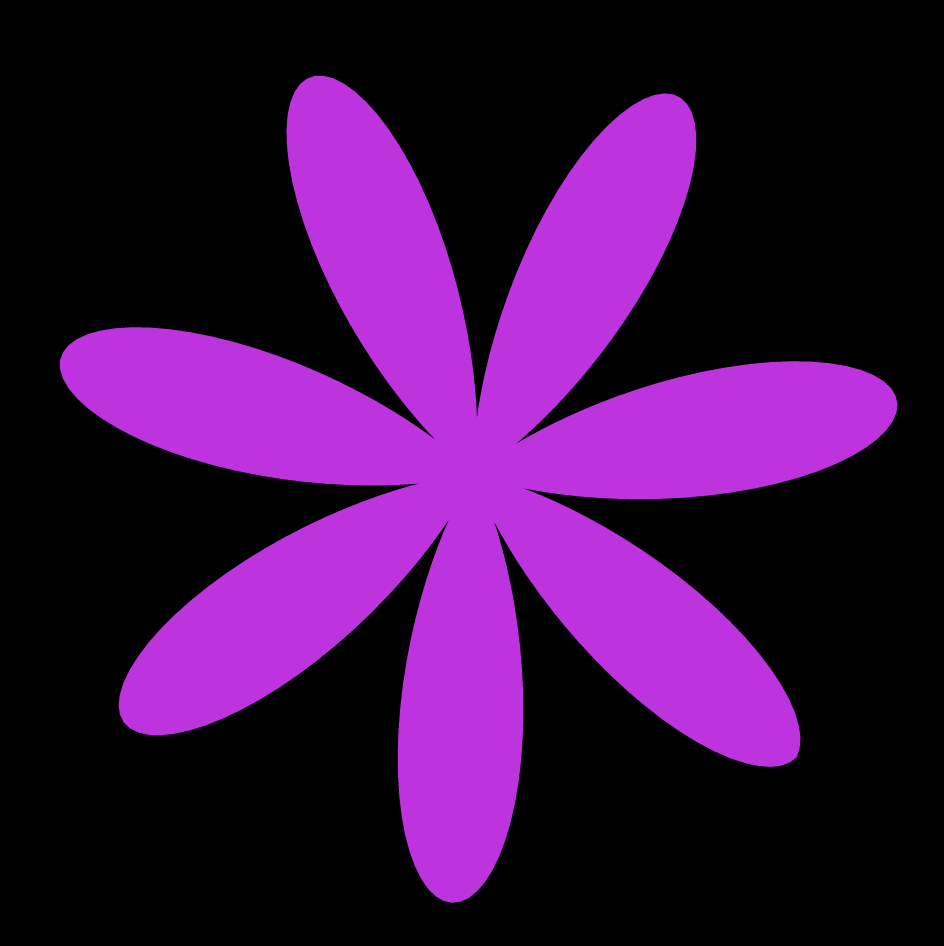
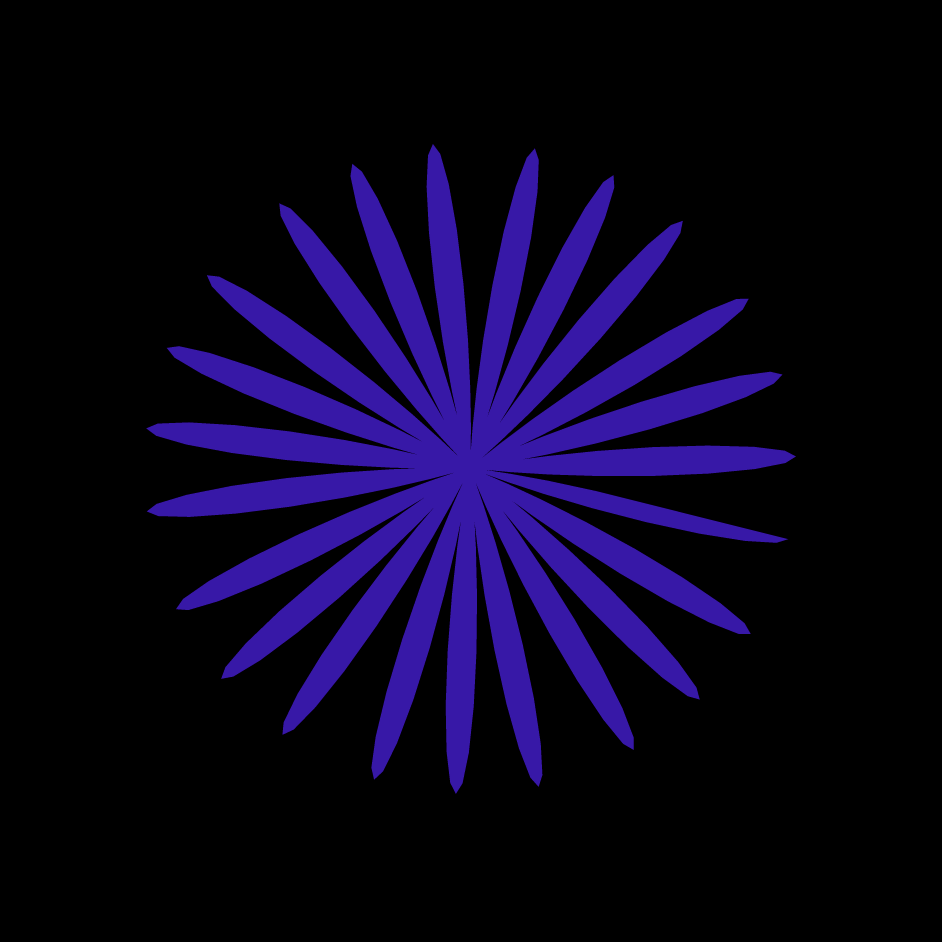
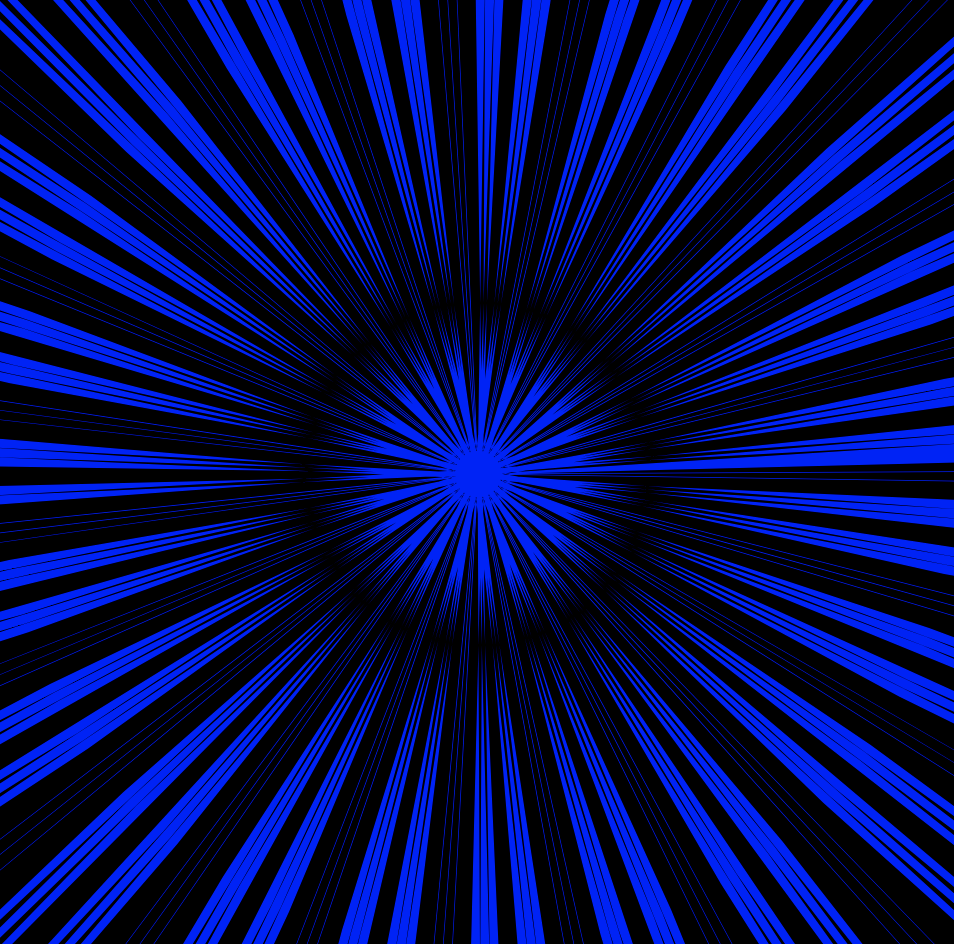
//Catherine Liu
//jianingl@andrew.cmu.edu
//Section D
//jianingl_07_Project
var type = 1 //keeps track of current type of curve
//draws two different types of curves that changes with mousePressed
function setup() {
createCanvas(480, 480);
}
function draw() {
//checks for current curve
if (type == 1) {
drawSextic();
} else if (type == 2) {
drawAstroid();
}
}
function drawAstroid() {
//Astroid
//https://mathworld.wolfram.com/Astroid.html
//creates a circular form with curves around the circumference
var red = min(mouseX, 255);
var green = min(mouseY, 255);
fill(red, green, 0);
noStroke();
translate(width/2,height/2);
background(0);
beginShape();
var x ;
var y ;
var b = map(mouseY, 0, 480, 10, 20); //controls number of curves around circumference
var a = constrain(mouseX, 0, width/2); //controls size of circle curve
rotate(radians(mouseY/3));
for (i = 0; i < 480; i++) {
var theta = map(i,0,480, 0, TWO_PI);
x = (a-b)*cos(theta) + b*cos((a-b)/b*(theta));
y = (a-b)*sin(theta) - b*cos((a-b)/b*(theta));
vertex(x,y);
}
endShape();
}
function drawSextic() {
//Cayley's Sextic
//https://mathworld.wolfram.com/CayleysSextic.html
//creates a flower form with different numbers of petals
push();
var blue= min(mouseX, 255);
var red = min(mouseY, 255);
translate(width/2,height/2);
fill(red,0,blue);
noStroke();
background(0);
beginShape();
var x ;
var y ;
var b = map(mouseY, 0, 480, 0, 1); //controls rotation and number of petals
var a = map(mouseX, 0, width, 0, 150); //controls size of form
rotate(radians(mouseY/5));
for (i = 0; i < 480; i++) {
var theta = map(i, 0, 480, 0, PI * 3);
x = (3*a*pow(cos((1/b)*theta),3)*cos(theta));
y = (3*a*pow(cos((1/b)*theta),3)*sin(theta));
vertex(x,y);
}
endShape();
pop();
}
function mousePressed() {
//switches current curve when mouse is pressed
if (type == 1) {
type += 1
} else if (type == 2) {
type = 1
}
}