// John Henley; jhenley; 15-104 section D
function setup() {
createCanvas(480, 480);
background(0);
}
function draw() { // draw loop for each spiral
background(0);
translate(width/2, height/2);
spiral1(); // calls spiral functions
spiral2();
}
function spiral1() { // horizontal spirals
beginShape();
noFill();
stroke(255);
for (var t = 0; t <= 100; t += 20) { // for loop for inner rings
for (var i = 1; i <= 100; i ++) { // loop for each spiral
var theta = map(i, 0, 100, 0, TWO_PI);
var a = map(mouseX, 0, width/2, 0, 1); // maps mouseX onto 0 - 1 scale
var x = a * t * cos(theta)^20;
var y = a * t * sin(theta);
vertex(x, y);
endShape();
}
}
}
function spiral2() { // vertical spiral
beginShape();
noFill();
stroke(255);
for (var t = 0; t <= 100; t += 20) { // for loop for inner rings
for (var i = 1; i <= 100; i ++) { // loop for each sprial
var theta = map(i, 0, 100, 0, TWO_PI);
var a = map(mouseX, 0, width/2, 0, 1); // maps mouseX onto 0 - 1 scale
var x = a * t * cos(theta);
var y = a * t * sin(theta)^20;
vertex(x, y);
endShape();
}
}
}
I wanted to make my curves very sharp and dramatic rather than the traditional smooth spiral. I accomplished this by using the equation for a spiral (r*cos(theta)^x, parametrically) and adjusted the exponent value and values in the map function until I achieved the desire effect. Below are two separate states of the program: the first is when the mouse is far to the left of the canvas, and the second is when the mouse is far to the right.
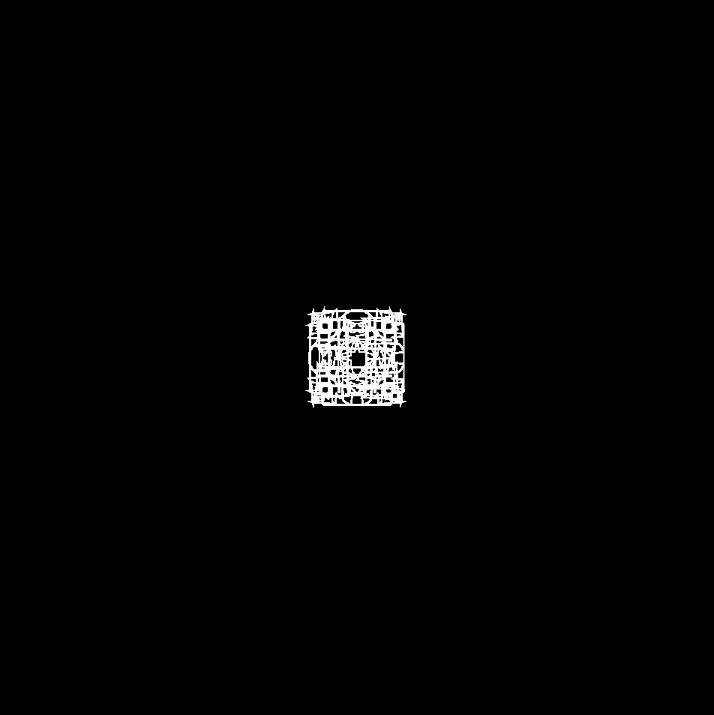
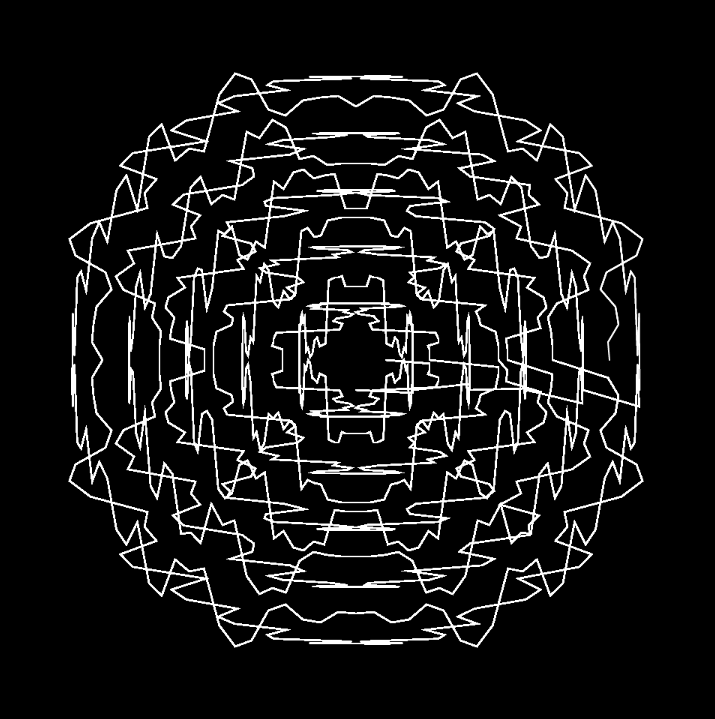