sketchDownload
/*Name:Camellia(Siyun) Wang;
Section: C;
Email Address: siyunw@andrew.cmu.edu;*/
https://imgur.com/qOtkTrJ
var img;
var i = 0;
var a = 0;
function preload(){
img = loadImage("https://i.imgur.com/qOtkTrJ.jpg");
}
function setup(){
createCanvas(380, 480);
background(0);
img.resize(380, 480);//resize the image to fit into canvas
img.loadPixels();
}
//based on the example code
/*function draw(){
var sw = map(mouseX, 0, width, 1, 5);//
var x = random(img.width); //randomly select pixels of the image
var y = random(img.height);
var pointColor = img.get(x, y); //select the color of the chosen pixel
stroke(pointColor);
strokeWeight(sw);
line(x,y,x+5,y);
}*/
//based on the simple nested loop logic
/*function draw(){
var i = random ()
pointColor = img.get(i, a);
noStroke();
fill(pointColor);
ellipse(i,a,random(1,5),random(3,7));
i += 5;
if(i >= 380){
a += 5;
i = 0;
}
}*/
//based on spiral motion
var angle = 0;
var radius = 0;
var r = 6.2;
function draw() {
var center_x = width / 2;
var center_y = height / 2;
var x = radius *cos(radians(angle));
var y = radius *sin(radians(angle));
pointColor = img.get(width/ 2+x, height/2+y);
//pick color of the chosen pixel from the center of the image
//picking in spiral motion which corresponds to the sipral motion of the drawn ellipses
push();
//translate the canvas to draw the spiral from the center outwards
translate(center_x, center_y);
noStroke();
fill(pointColor);
circle(x, y, r);
radius += 0.1;
angle += 5;
r += 0.01;
pop();
}
I started this project with duplicating the example code, then I used the nested loop logic to do the second trial. However, the way this portrait was drawn did not seem interesting enough, since there’s no change in motion and each drawn square. Therefore, I changed the drawing logic to the spiral motion. In this way, the portrait is drawn from the center in gradually amplifying ellipses, so that the final piece has a clearer shape of face in the center and blurry periphery.
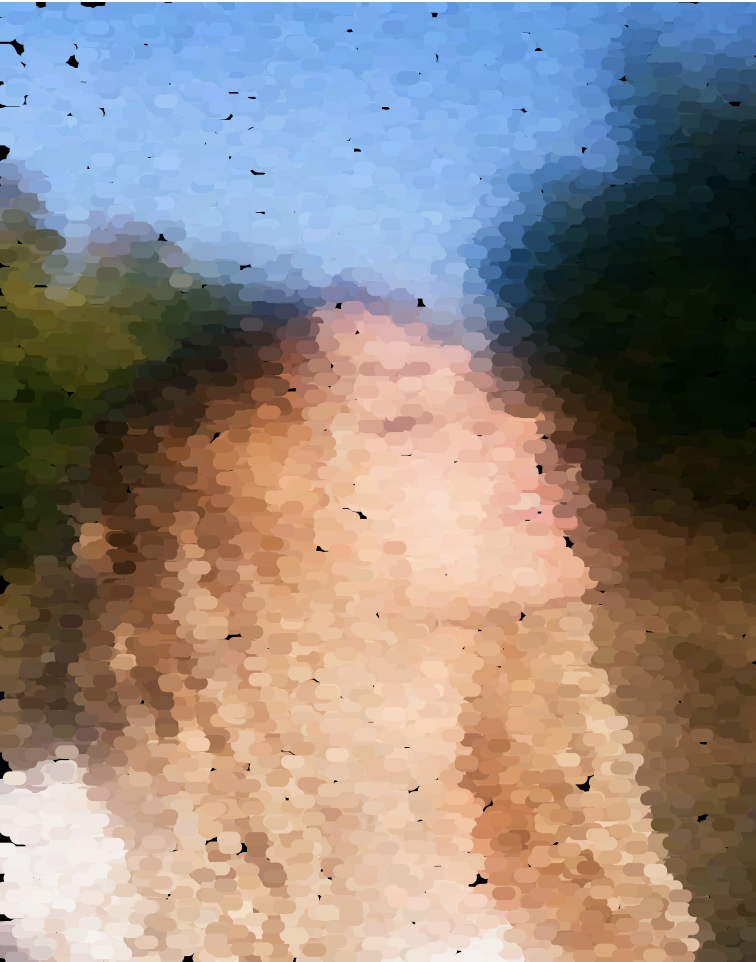
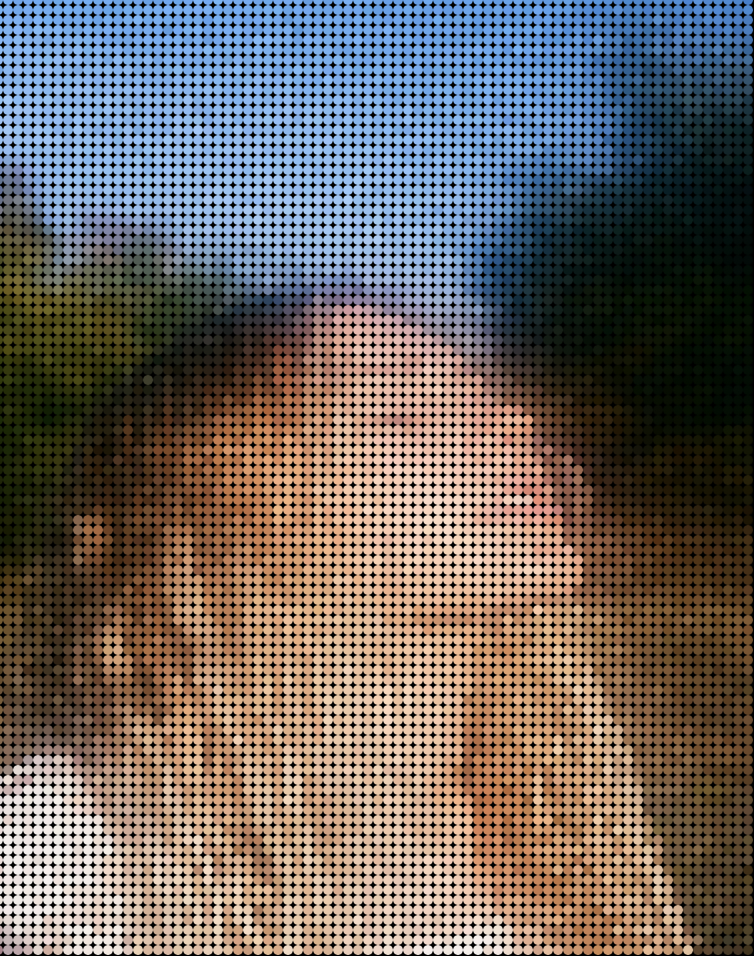
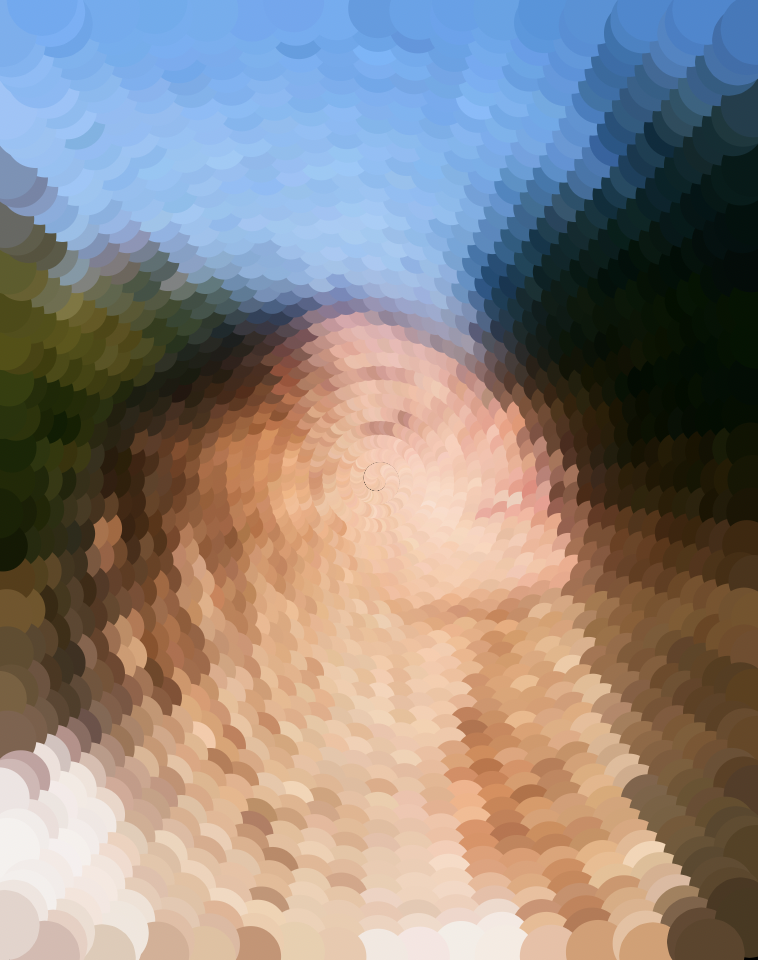